Using NEXT.js with AWS Lambda Edge: Serverless at the Edge
In today’s digital landscape, where every millisecond counts, delivering fast and responsive web experiences is paramount. Users expect websites to load quickly, regardless of their geographical location. This is where serverless computing at the edge comes into play, and when combined with NEXT.js, it becomes a powerful tool to supercharge your web applications. In this blog, we’ll explore the concept of serverless at the edge and demonstrate how to implement it using NEXT.js and AWS Lambda Edge.
1. Understanding Serverless at the Edge
1.1. What is AWS Lambda Edge?
AWS Lambda Edge is a service provided by Amazon Web Services (AWS) that allows you to run code in response to specific CloudFront events at edge locations. Edge locations are AWS data centers located in various geographic regions, bringing content closer to your end-users. AWS Lambda Edge enables you to execute code at these edge locations, which can significantly improve the performance and latency of your web applications.
1.2. Why Use Serverless at the Edge?
Serverless computing at the edge offers several advantages:
- Reduced Latency: By executing code closer to the end-users, you can significantly reduce the latency of your applications, resulting in faster load times.
- Scalability: Serverless architectures automatically scale based on demand, ensuring that your application can handle traffic spikes without manual intervention.
- Cost-Efficiency: You only pay for the compute resources used during execution, making it cost-effective for both small and large applications.
- Global Reach: Edge locations are spread worldwide, enabling you to deliver content globally with minimal latency.
Now that we have a basic understanding of AWS Lambda Edge, let’s dive into the practical aspects of setting up a NEXT.js application with serverless computing at the edge.
2. Setting Up Your NEXT.js Application
2.1. Prerequisites
Before we get started, make sure you have the following prerequisites in place:
- Node.js and npm installed on your development machine.
- An AWS account set up with the necessary permissions.
- The AWS CLI (Command Line Interface) installed and configured with your AWS credentials.
- A basic understanding of NEXT.js.
2.2. Creating a NEXT.js Project
Let’s begin by creating a new NEXT.js project. If you don’t have NEXT.js installed globally, you can do so by running:
bash npm install -g create-next-app
Now, create a new NEXT.js project with the following command:
bash npx create-next-app my-next-app
This will create a new directory called my-next-app with a basic NEXT.js project structure.
2.3. Building Your Application
Next, navigate to your project directory and start the development server:
bash cd my-next-app npm run dev
You can access your new NEXT.js application by visiting http://localhost:3000 in your web browser. You should see the default NEXT.js landing page.
With your NEXT.js application set up, it’s time to integrate it with AWS Lambda Edge for serverless computing at the edge.
3. Leveraging AWS Lambda Edge with NEXT.js
3.1. Deploying Your NEXT.js App to AWS Lambda
To deploy your NEXT.js application to AWS Lambda, you’ll need to create a serverless function that handles the requests at the edge locations. Follow these steps:
Step 1: Install Serverless Framework
The Serverless Framework simplifies the deployment process. Install it globally using npm:
bash npm install -g serverless
Step 2: Initialize Serverless Project
Navigate to your NEXT.js project directory and initialize a new Serverless project:
bash cd my-next-app serverless
This will create a serverless.yml file in your project directory, where you can define your serverless functions and their configurations.
Step 3: Define AWS Lambda Function
Edit the serverless.yml file to define your AWS Lambda function:
yaml service: my-next-app provider: name: aws runtime: nodejs14.x region: us-east-1 # Choose your desired region functions: edgeFunction: handler: handler.edge events: - http: path: / method: ANY
In this configuration, we define an AWS Lambda function named edgeFunction that responds to HTTP requests at the root path (/). The handler.edge refers to the code that will be executed.
Step 4: Create Lambda Function Code
Now, create a new file named handler.js in your project directory with the following content:
javascript module.exports.edge = async (event) => { const response = { statusCode: 200, body: JSON.stringify({ message: 'Hello from Lambda Edge!' }), }; return response; };
This code defines a basic Lambda function that returns a JSON response with a simple message.
Step 5: Deploy to AWS Lambda
Deploy your NEXT.js application and Lambda function to AWS by running:
bash serverless deploy
Once deployed, Serverless will provide you with the endpoint URL for your Lambda function. You can now configure AWS Lambda Edge to use this function at your CloudFront distribution.
3.2. Configuring AWS Lambda Edge
To configure AWS Lambda Edge to work with your NEXT.js application, follow these steps:
Step 1: Open AWS CloudFront Console
Log in to your AWS Management Console, navigate to the CloudFront service, and select your distribution.
Step 2: Add Trigger to CloudFront Distribution
- In the CloudFront distribution settings, go to the “Behaviors” tab.
- Select the behavior for which you want to use Lambda Edge.
- Click “Edit” and choose the Lambda function you deployed earlier (e.g., my-next-app-dev-edgeFunction).
- Save your changes.
Now, your NEXT.js application is set up to use AWS Lambda Edge for serverless computing at the edge.
4. Optimizing Your Website with Serverless at the Edge
4.1. Caching Strategies
Caching plays a crucial role in optimizing your website’s performance. With AWS Lambda Edge, you can implement caching strategies such as:
- Content Caching: Cache your website’s content at edge locations to reduce latency for subsequent requests.
- Dynamic Content: Implement caching for dynamic content, ensuring a balance between freshness and performance.
- Cache Invalidation: Use Lambda Edge to invalidate caches when content is updated.
4.2. Image Optimization
Serverless at the edge allows you to optimize images on-the-fly, delivering the right size and format based on the user’s device and network conditions. This reduces the bandwidth and improves the user experience.
4.3. Global Distribution
With AWS Lambda Edge, your NEXT.js application can be distributed globally, ensuring low-latency access for users across the world. This is crucial for providing a seamless user experience, especially for international audiences.
5. Monitoring and Troubleshooting
5.1. AWS CloudWatch
AWS Lambda Edge integrates seamlessly with AWS CloudWatch, allowing you to monitor the performance and troubleshoot any issues. Set up CloudWatch alarms to receive notifications for specific events or anomalies in your application.
5.2. Debugging and Testing
When debugging your Lambda Edge functions, consider using tools like AWS X-Ray for distributed tracing and AWS CloudTrail for auditing and tracking changes.
Conclusion
In this blog, we’ve explored the powerful combination of NEXT.js and AWS Lambda Edge for serverless computing at the edge. By leveraging this technology stack, you can deliver lightning-fast web experiences, optimize your content delivery, and scale your applications effortlessly. As the digital landscape continues to evolve, staying ahead of the curve with serverless at the edge can give your websites a significant competitive advantage.
Now, it’s time to put this knowledge into practice and transform your web applications into high-performing, globally accessible platforms. Embrace the world of serverless at the edge and take your web development to the next level.
Table of Contents
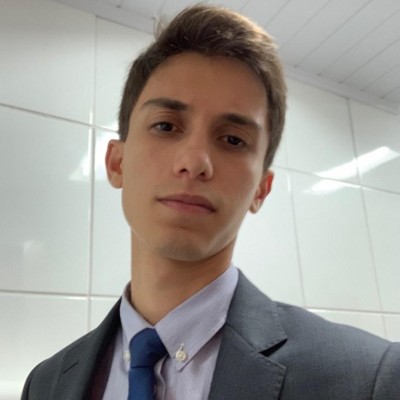
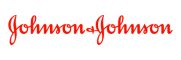