Building a Blogging Platform with NEXT.js and Markdown
In today’s digital age, sharing your thoughts, experiences, and expertise has become easier than ever with the advent of blogging platforms. However, with so many options available, it’s essential to stand out by providing an excellent user experience and leveraging modern technologies. In this tutorial, we’ll guide you through the process of building a blogging platform using NEXT.js and Markdown, combining the simplicity of Markdown with the performance and flexibility of NEXT.js.
1. Why NEXT.js and Markdown?
NEXT.js has gained immense popularity as a React framework that enables server-side rendering, static site generation, and client-side rendering, all in one package. This makes it an ideal choice for building performant and SEO-friendly web applications. On the other hand, Markdown is a lightweight markup language that allows you to write content using a simple syntax. By using Markdown, you can focus on your content without getting bogged down in complex HTML formatting.
2. Prerequisites
Before diving into the implementation, make sure you have the following prerequisites set up:
- Node.js and npm installed on your machine.
- Basic knowledge of React and JavaScript.
- Familiarity with NEXT.js is a plus, but not mandatory.
3. Setting Up the Project
Let’s start by setting up our project using the following steps:
Step 1: Initialize a new project
Open your terminal and create a new directory for your project. Navigate to the newly created directory and run the following commands:
bash mkdir nextjs-blog-platform cd nextjs-blog-platform npm init -y
Step 2: Install dependencies
1. We’ll need to install several packages to get our blogging platform up and running:
bash npm install next react react-dom npm install gray-matter remark remark-html
2. next, react, and react-dom are essential dependencies for building a NEXT.js application.
3. gray-matter helps us parse frontmatter from Markdown files.
4. remark and remark-html are used to process Markdown content and convert it to HTML.
4. Creating the Blog Structure
Now that our project is set up and dependencies are installed, let’s create the basic structure for our blog.
Step 1: Create folders
Inside the root directory of your project, create the following folders:
bash mkdir pages mkdir posts
The pages directory is where our application’s pages will reside.
The posts directory will contain our blog posts in Markdown format.
Step 2: Create sample posts
Inside the posts directory, create a sample Markdown file named first-post.md with the following content:
markdown --- title: My First Blog Post date: 2023-08-19 ---
Welcome to my first blog post using NEXT.js and Markdown! In this post, I’ll share my experiences with building a blogging platform.
5. Building the Blog Layout
With the basic structure in place, let’s create the layout for our blog.
Step 1: Create the index page
Inside the pages directory, create an index.js file with the following content:
jsx import fs from 'fs'; import path from 'path'; import Link from 'next/link'; import matter from 'gray-matter'; import React from 'react'; export default function Home({ posts }) { return ( <div> <h1>Welcome to My Blog</h1> <ul> {posts.map((post) => ( <li key={post.slug}> <Link href={`/posts/${post.slug}`}> <a>{post.frontmatter.title}</a> </Link> </li> ))} </ul> </div> ); } export async function getStaticProps() { const postsDirectory = path.join(process.cwd(), 'posts'); const filenames = fs.readdirSync(postsDirectory); const posts = filenames.map((filename) => { const filePath = path.join(postsDirectory, filename); const fileContents = fs.readFileSync(filePath, 'utf8'); const { data: frontmatter } = matter(fileContents); return { slug: filename.replace('.md', ''), frontmatter, }; }); return { props: { posts, }, }; }
In this code, we’re using NEXT.js’s getStaticProps function to fetch the list of blog posts from the posts directory. We’re using gray-matter to parse the frontmatter from the Markdown files.
6. Displaying Individual Blog Posts
To display individual blog posts, we need to create a dynamic route that fetches the content of a specific post.
Step 1: Create a dynamic route
Inside the pages directory, create a [slug].js file with the following content:
jsx import fs from 'fs'; import path from 'path'; import matter from 'gray-matter'; import React from 'react'; export default function Post({ post }) { return ( <div> <h1>{post.frontmatter.title}</h1> <div dangerouslySetInnerHTML={{ __html: post.content }} /> </div> ); } export async function getStaticPaths() { const postsDirectory = path.join(process.cwd(), 'posts'); const filenames = fs.readdirSync(postsDirectory); const paths = filenames.map((filename) => ({ params: { slug: filename.replace('.md', '') }, })); return { paths, fallback: false, }; } export async function getStaticProps({ params }) { const { slug } = params; const filePath = path.join(process.cwd(), 'posts', `${slug}.md`); const fileContents = fs.readFileSync(filePath, 'utf8'); const { data: frontmatter, content } = matter(fileContents); return { props: { post: { slug, frontmatter, content, }, }, }; }
In this code, the [slug].js file fetches the specific Markdown file for the requested post using getStaticProps and displays its content.
7. Styling and Customization
To enhance the visual appeal of your blogging platform, you can style it using CSS or popular UI frameworks like Tailwind CSS.
Conclusion
Congratulations! You’ve successfully built a blogging platform using NEXT.js and Markdown. This combination of technologies allows you to create and manage your blog content efficiently while maintaining excellent performance and SEO optimization. With the foundation in place, you can further customize and expand your platform by adding features like category filters, pagination, and user authentication. Happy blogging!
In this tutorial, we’ve covered the essential steps to build a blogging platform using NEXT.js and Markdown. From setting up the project to displaying individual blog posts, you now have the tools to create a fully functional and performant blogging platform. Remember to explore the vast possibilities for customization, such as adding comments, social sharing buttons, and enhancing the design. Happy coding and happy blogging!
Table of Contents
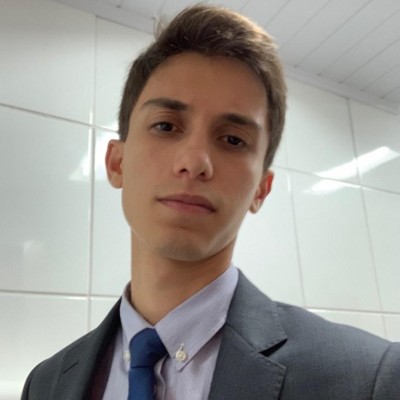
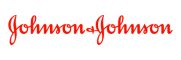