Exploring Next.js: A Step-by-Step Guide to Building Your First Application
In the ever-evolving landscape of web development, server-side rendering (SSR) stands as a revolutionary technology that significantly enhances user experience by improving page load times and enabling search engine optimization. NEXT.js, a popular React framework, provides built-in support for SSR, making it an attractive choice for developers looking to build performant, SEO-friendly web applications. This benefit also explains why many companies choose to hire Next.js developers for their projects.
This blog post will delve into the concepts and methodologies around server-side rendering in NEXT.js. Whether you are a developer or looking to hire Next.js developers, understanding these concepts will be crucial. This guide is replete with practical examples, all aimed at helping you master this technology.
What is Server-side Rendering (SSR)?
Before we dive into NEXT.js, let’s understand the basics of server-side rendering. SSR is a method where the server computes the initial state of a web application and returns the rendered HTML to the client, rather than sending an almost empty HTML file that the browser then populates using JavaScript. This approach significantly improves the time to first paint (TTFP) and enhances the SEO capabilities of your web application.
Why NEXT.js?
NEXT.js is a powerful React framework that helps developers build JavaScript applications with less setup time and more focus on development. One of its key features is the built-in support for SSR, meaning you can leverage SSR without handling the complex configurations that would typically be required.
Getting Started With NEXT.js
To start using NEXT.js, you need to have Node.js (version 10.13 or later) and npm installed on your computer. This can be a daunting task for beginners, hence some companies opt to hire Next.js developers to set things up seamlessly. You can create a new NEXT.js application using create-next-app, which sets up everything automatically for you. Here’s how you, or the Next.js developers you hire, can kick-start the process. Run the following command to create a new app:
```bash npx create-next-app@latest my-app ```
This command will create a new NEXT.js app in a directory called “my-app”. You can replace “my-app” with the name of your project.
Utilizing SSR in NEXT.js
NEXT.js has a built-in function called `getServerSideProps()` that you can use to fetch data at request time. This function runs on the server-side for every request and can be used to fetch data that is required to render the page.
Let’s demonstrate this with an example of a blog post page:
```jsx import fetch from 'node-fetch'; function Post({ post }) { return ( <div> <h1>{post.title}</h1> <p>{post.body}</p> </div> ); } export async function getServerSideProps(context) { const { id } = context.query; const res = await fetch(`https://jsonplaceholder.typicode.com/posts/${id}`); const post = await res.json(); return { props: { post }, // will be passed to the page component as props }; } export default Post; ```
In this example, `getServerSideProps()` fetches a blog post based on the ID, which is taken from the page context. The fetched data is then passed to the page component via props.
This server-side rendered page will display the fetched blog post, and since the data is fetched at request time, the browser will receive a fully rendered page, enhancing the user’s perceived performance and SEO effectiveness.
Handling Errors in SSR
Errors can occur during server-side rendering, and it’s essential to handle them effectively. For instance, in the above example, if the fetch request fails, your application will crash. You can handle errors by using a try/catch block inside `getServerSideProps()`.
```jsx export async function getServerSideProps(context) { const { id } = context.query; try { const res = await fetch(`https://jsonplaceholder.typicode.com/posts/${id}`); if (res.ok) { const post = await res.json(); return { props: { post } }; } } catch (error) { console.error(error); } // Return not found page if post is not fetched return { notFound: true, }; } ```
In this updated example, if the fetch request fails or the response status is not OK, `getServerSideProps()` will return a notFound value, which will cause NEXT.js to render a 404 page.
Client-side Rendering vs. Server-side Rendering
It’s important to understand that not every page requires server-side rendering. Sometimes, you might not have the necessary data at the time of the request, or maybe the data is user-specific and cannot be cached by a CDN.
For pages that need data to be fetched on the client side, NEXT.js provides a hook called `useSWR()` that can be used to fetch data on the client side. This hook also provides many features like caching, revalidation, focus tracking, etc.
Let’s look at an example of client-side data fetching using `useSWR()`:
```jsx import useSWR from 'swr'; function Profile() { const { data, error } = useSWR('/api/user', fetch); if (error) return <div>Failed to load</div>; if (!data) return <div>Loading...</div>; return ( <div> <h1>{data.name}</h1> <p>{data.email}</p> </div> ); } export default Profile; ```
In this example, `useSWR()` is fetching data from the “/api/user” endpoint on the client side. While the data is being fetched, a loading message is displayed. If there is an error in fetching data, an error message is displayed.
Conclusion
In this post, we have explored server-side rendering in NEXT.js, providing practical examples along the way. By leveraging the power of SSR in NEXT.js, which is a key reason why many organizations hire Next.js developers, you can greatly enhance the performance and SEO of your web applications. However, it’s important to remember that SSR isn’t a one-size-fits-all solution and not every page requires SSR.
Being mindful of when to use client-side rendering vs. server-side rendering can help you design more efficient and user-friendly web applications. As always, understanding your project’s unique requirements, or those of the company for which you hire Next.js developers, will guide you in selecting the right approach.
Whether you’re new to SSR, looking to optimize an existing application, or looking to hire Next.js developers for your project, I hope this guide has provided you with some useful insights into mastering server-side rendering in NEXT.js.
Table of Contents
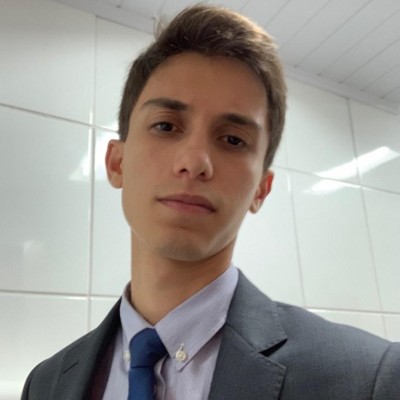
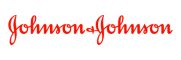