Building a Blog with NEXT.js: Creating Dynamic Content
In the world of web development, building a blog is a common task. With the rise of modern frameworks and libraries, creating dynamic content for a blog has become easier than ever. In this tutorial, we will explore how to build a blog using NEXT.js, a popular React framework known for its powerful server-side rendering capabilities. We will dive into the concepts of server-side rendering, API routes, and dynamic content generation to create a robust and scalable blog.
1. Setting up the Environment:
Before we dive into building our blog, we need to set up the development environment. First, let’s install NEXT.js globally using npm or yarn.
bash npm install -g next # or yarn global add next
Once NEXT.js is installed, we can create a new project using the following command:
bash npx create-next-app my-blog # or yarn create next-app my-blog
2. Creating the Blog Layout:
A good blog should have a clean and organized layout. Let’s start by setting up the basic structure of our blog.
2.1 Setting up the Navigation:
In the pages directory of your NEXT.js project, create a new file called _app.js. This file will act as the entry point for our application. Inside _app.js, we can set up the navigation component.
jsx import React from 'react'; import Link from 'next/link'; const MyApp = ({ Component, pageProps }) => { return ( <div> <nav> <Link href="/">Home</Link> <Link href="/about">About</Link> <Link href="/contact">Contact</Link> </nav> <Component {...pageProps} /> </div> ); }; export default MyApp;
2.2 Building the Header and Footer:
Next, let’s create a reusable Header and Footer component. Inside the components directory, create two files: Header.js and Footer.js.
Header.js:
jsx import React from 'react'; const Header = () => { return <header>This is the header</header>; }; export default Header;
Footer.js:
jsx import React from 'react'; const Footer = () => { return <footer>This is the footer</footer>; }; export default Footer;
Now, let’s import and use these components in our _app.js file.
jsx import React from 'react'; import Link from 'next/link'; import Header from '../components/Header'; import Footer from '../components/Footer'; const MyApp = ({ Component, pageProps }) => { return ( <div> <Header /> <nav> <Link href="/">Home</Link> <Link href="/about">About</Link> <Link href="/contact">Contact</Link> </nav> <Component {...pageProps} /> <Footer /> </div> ); }; export default MyApp;
3. Server-side Rendering with NEXT.js:
One of the key features of NEXT.js is its server-side rendering (SSR) capability. SSR allows us to pre-render pages on the server before sending them to the client. Let’s explore how to fetch blog posts from an API using server-side rendering.
3.1 Understanding Server-side Rendering:
In NEXT.js, we can use the getServerSideProps function to fetch data from an API during the server-side rendering process. Inside the page component, define the getServerSideProps function to fetch the blog posts.
jsx import React from 'react'; const Home = ({ posts }) => { return ( <div> <h1>Welcome to my Blog</h1> {posts.map((post) => ( <div key={post.id}>{post.title}</div> ))} </div> ); }; export async function getServerSideProps() { const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); return { props: { posts, }, }; } export default Home;
3.2 Fetching Blog Posts from an API:
In the above example, we fetch the blog posts from an API endpoint using the fetch function. We then pass the fetched data as a prop to the Home component. By doing this, the blog posts will be available during the server-side rendering process.
4. Building Dynamic Blog Pages:
Next, let’s focus on creating dynamic blog pages. We want to generate individual pages for each blog post.
4.1 Creating a Dynamic Page Template:
In the pages directory, create a new directory called blog and inside it, create a new file called [slug].js. This file will act as a template for rendering individual blog post pages.
jsx import React from 'react'; const BlogPost = ({ post }) => { return ( <div> <h1>{post.title}</h1> <p>{post.content}</p> </div> ); }; export async function getServerSideProps(context) { const { slug } = context.query; const res = await fetch(`https://api.example.com/posts/${slug}`); const post = await res.json(); return { props: { post, }, }; } export default BlogPost;
4.2 Generating Dynamic Routes:
To generate dynamic routes for each blog post, we need to create a file called index.js inside the pages/blog directory.
jsx import React from 'react'; import Link from 'next/link'; const Blog = ({ posts }) => { return ( <div> <h1>Blog Posts</h1> {posts.map((post) => ( <div key={post.id}> <Link href={`/blog/${post.slug}`}> <a>{post.title}</a> </Link> </div> ))} </div> ); }; export async function getServerSideProps() { const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); return { props: { posts, }, }; } export default Blog;
4.3 Fetching Dynamic Data:
In the above example, we fetch the individual blog post data by accessing the slug parameter from the context.query object. The slug parameter is extracted from the URL and used to fetch the corresponding blog post from the API.
5. Creating API Routes for Content Management:
To manage the content of our blog, we need to create API routes for handling CRUD operations.
5.1 Setting up the API Routes:
Inside the pages/api directory, create a new file called posts.js. This file will handle the API routes for managing blog posts.
jsx const handler = (req, res) => { if (req.method === 'GET') { // Handle GET request for fetching blog posts // ... } else if (req.method === 'POST') { // Handle POST request for creating a new blog post // ... } else if (req.method === 'PUT') { // Handle PUT request for updating an existing blog post // ... } else if (req.method === 'DELETE') { // Handle DELETE request for deleting a blog post // ... } else { res.status(405).json({ message: 'Method Not Allowed' }); } }; export default handler;
5.2 Handling POST Requests:
Inside the posts.js file, let’s focus on handling the POST request for creating a new blog post.
jsx const handler = (req, res) => { if (req.method === 'POST') { const { title, content } = req.body; // Validate the request body if (!title || !content) { res.status(400).json({ message: 'Title and content are required' }); return; } // Perform the necessary operations to create the blog post // ... res.status(201).json({ message: 'Blog post created successfully' }); } else { res.status(405).json({ message: 'Method Not Allowed' }); } }; export default handler;
Conclusion:
In this tutorial, we explored how to build a dynamic blog using NEXT.js. We covered the basics of setting up the environment, creating the blog layout, implementing server-side rendering, generating dynamic blog pages, and creating API routes for content management. NEXT.js provides a powerful and efficient way to build dynamic and scalable blogs. With the knowledge gained from this tutorial, you can now create your own custom blog with ease using NEXT.js. Happy coding!
Table of Contents
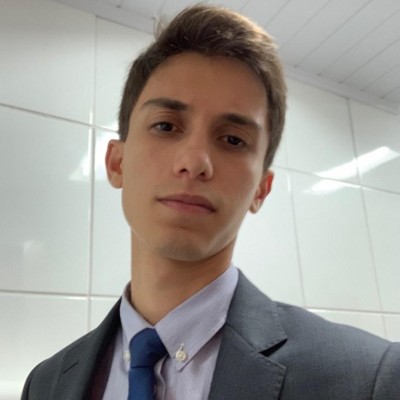
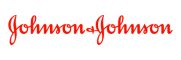