Building a Multi-language Website with NEXT.js: i18n and Next.js Internationalized Routing
In our interconnected world, the ability to create websites that cater to a global audience is more important than ever. One of the key aspects of building a truly global website is providing content in multiple languages. Fortunately, with the advent of modern web development tools, this task has become significantly easier. In this tutorial, we’ll explore how to create a multi-language website using NEXT.js, a popular React framework, while harnessing its i18n capabilities and internationalized routing.
1. Understanding the Basics
Before diving into the technicalities, let’s get a clear picture of what internationalization (i18n) and internationalized routing mean in the context of web development.
- Internationalization (i18n): Internationalization involves designing your application in a way that allows it to be easily adapted to different languages, regions, and cultures. It separates the content from the code, enabling you to provide translations for different languages without modifying the core functionality of your application.
- Internationalized Routing: Internationalized routing involves creating routes for different languages, allowing users to access content in their preferred language while maintaining a consistent URL structure. This not only enhances user experience but also has SEO benefits.
2. Setting Up Your NEXT.js Project
To start building our multi-language website, we’ll first set up a new NEXT.js project.
2.1. Create a new project:
Open your terminal and run the following commands:
bash npx create-next-app my-multi-language-site cd my-multi-language-site
2.2. Install Dependencies:
We’ll need a few packages to enable i18n and internationalized routing in our NEXT.js project:
bash npm install next-translate @next/router
3. Implementing i18n with next-translate
next-translate is a fantastic package that simplifies the process of adding internationalization to your NEXT.js application.
3.1. Configure next-translate:
Create a next.config.js file in the root of your project and add the following:
javascript const nextTranslate = require('next-translate'); module.exports = nextTranslate();
3.2. Prepare Your Translations:
Inside your project’s root directory, create a folder named locales. Within this folder, create separate JSON files for each supported language. For instance, en.json for English and es.json for Spanish.
Here’s an example of how your translation files might look:
json // locales/en.json { "welcome": "Welcome to our website!", "about": "About Us", "contact": "Contact Us" } // locales/es.json { "welcome": "¡Bienvenidos a nuestro sitio web!", "about": "Acerca de Nosotros", "contact": "Contáctanos" }
3.3. Implement Translations:
In your application’s components or pages, you can now use the useTranslation hook from next-translate to access translated content. Here’s an example of how you might use it in a React component:
javascript import { useTranslation } from 'next-translate'; function HomePage() { const { t } = useTranslation(); return ( <div> <h1>{t('welcome')}</h1> <p>{t('about')}</p> <p>{t('contact')}</p> </div> ); }
4. Implementing Internationalized Routing
NEXT.js provides a versatile routing system, and with the help of the @next/router package, we can easily set up internationalized routing.
4.1. Create Language-specific Routes:
In your project’s pages directory, create subdirectories for each language you want to support. For example, en for English and es for Spanish. Inside these subdirectories, create pages that correspond to your main site’s pages.
Your project structure might look like this:
plaintext pages/ ??? en/ ? ??? index.js (Home page for English) ? ??? about.js (About page for English) ? ??? contact.js (Contact page for English) ??? es/ ? ??? index.js (Home page for Spanish) ? ??? about.js (About page for Spanish) ? ??? contact.js (Contact page for Spanish)
4.2. Implement Language-aware Routing:
To make sure users are directed to the appropriate language-specific routes, modify your main pages/index.js file like this:
javascript import { useRouter } from '@next/router'; function HomePage() { const router = useRouter(); const handleLanguageChange = (language) => { router.push('/', '/', { locale: language }); }; return ( <div> <h1>{t('welcome')}</h1> <button onClick={() => handleLanguageChange('en')}>English</button> <button onClick={() => handleLanguageChange('es')}>Español</button> </div> ); }
In this example, clicking the language buttons will route the user to the homepage in the selected language.
5. Enhancing User Experience and SEO
Creating a multi-language website isn’t just about translations and routes; it’s also about enhancing the user experience and maintaining good SEO practices.
5.1. Language Switcher:
Consider adding a language switcher component that allows users to easily switch between languages on any page. You can create a reusable component that toggles the language via the useRouter hook, similar to the example shown above.
5.2. SEO Considerations:
For SEO purposes, it’s important to include the lang attribute in your HTML head tag to indicate the language of your content. You can do this using the next/head module in your page components.
javascript import Head from 'next/head'; function AboutPage() { return ( <div> <Head> <title>About Us</title> <meta name="description" content="Learn about our company." /> <meta lang="en" /> </Head> <h1>{t('about')}</h1> </div> ); }
Conclusion
Building a multi-language website using NEXT.js is a powerful way to cater to a global audience while maintaining a seamless user experience. With the combination of the next-translate package and internationalized routing, you can easily provide translations for different languages and ensure that users are directed to the correct language-specific content. By considering additional features like a language switcher and adhering to SEO best practices, you can create a website that truly speaks to users from around the world.
Incorporating i18n and internationalized routing might initially seem complex, but the benefits in terms of user engagement, user satisfaction, and improved SEO make the effort well worth it. So why wait? Start building your own multi-language website with NEXT.js today and embark on a journey to reach a global audience like never before.
Table of Contents
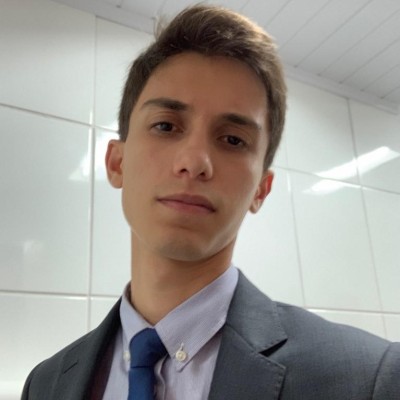
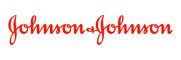