Building a Podcast App with NEXT.js and Podcaster
In the digital age, podcasts have become a popular medium for sharing information, stories, and entertainment. As the podcasting industry continues to grow, so does the demand for user-friendly podcast apps that provide a seamless listening experience. If you’re a web developer looking to create your own podcast app, you’re in luck! In this comprehensive guide, we’ll walk you through the process of building a feature-rich podcast app using NEXT.js and the Podcaster library.
1. Prerequisites
Before we dive into building our podcast app, let’s make sure you have the necessary prerequisites in place:
1.2. Node.js and npm
Ensure you have Node.js and npm (Node Package Manager) installed on your system. You can download them from the official website (https://nodejs.org/).
1.2. Basic Knowledge of React
Familiarize yourself with React, a JavaScript library for building user interfaces. If you’re new to React, you can start with the official documentation (https://reactjs.org/).
1.3. Text Editor or IDE
Choose a text editor or integrated development environment (IDE) of your preference. Popular choices include Visual Studio Code, Sublime Text, or JetBrains WebStorm.
1.4. Git
Install Git for version control. You can download it from the official website (https://git-scm.com/).
Now that we have our prerequisites covered, let’s start building our podcast app step by step.
2. Setting Up the Project
2.1. Create a New NEXT.js Project
Open your terminal and run the following commands:
bash npx create-next-app podcast-app cd podcast-app
This will create a new NEXT.js project called “podcast-app” and navigate to its directory.
2.2. Install Dependencies
Next, we’ll need to install some dependencies for our podcast app. Run the following command:
bash npm install podcaster
Podcaster is a lightweight library for integrating podcast functionality into your web app, making it perfect for our project.
3. Building the Podcast App
With our project set up and dependencies installed, it’s time to start building the podcast app.
3.1. Create the Podcast Component
Inside the pages directory of your project, create a new file called podcast.js. This will be the main component for displaying podcasts. Here’s a basic structure for your podcast.js file:
jsx import React from 'react'; import { Podcaster } from 'podcaster'; const Podcast = () => { return ( <div> {/* Add your podcast list here */} </div> ); }; export default Podcast;
3.2. Fetch Podcast Data
To display podcasts, you’ll need to fetch podcast data. You can use an API like Apple Podcasts API or create your own database of podcasts. For simplicity, let’s assume you have a JSON file containing podcast information.
Create a folder named data in your project root and add a JSON file named podcasts.json with sample podcast data:
json [ { "id": 1, "title": "Podcast 1", "description": "Description of Podcast 1", "audioUrl": "https://example.com/podcast1.mp3" }, { "id": 2, "title": "Podcast 2", "description": "Description of Podcast 2", "audioUrl": "https://example.com/podcast2.mp3" }, // Add more podcasts as needed ]
In your podcast.js component, import this data and map it to display the podcasts:
jsx import React from 'react'; import { Podcaster } from 'podcaster'; import podcastsData from '../data/podcasts.json'; const Podcast = () => { return ( <div> {podcastsData.map((podcast) => ( <div key={podcast.id}> <h2>{podcast.title}</h2> <p>{podcast.description}</p> <audio controls> <source src={podcast.audioUrl} type="audio/mpeg" /> Your browser does not support the audio element. </audio> </div> ))} </div> ); }; export default Podcast;
3.3. Styling Your Podcast App
To make your podcast app visually appealing, you can use CSS or a styling library like Tailwind CSS. Style your components as needed to create an attractive user interface.
3.4. Routing
To navigate to your podcast page, set up routing in NEXT.js. You can use the built-in Link component for this purpose. In your pages/index.js file, add a link to the podcast page:
jsx import Link from 'next/link'; const Home = () => { return ( <div> <h1>Welcome to My Podcast App</h1> <Link href="/podcast"> <a>Go to Podcasts</a> </Link> </div> ); }; export default Home;
3.5. Testing Your App
To test your podcast app locally, run the following command in your project directory:
bash npm run dev
This will start your development server, and you can access your app in your web browser at http://localhost:3000.
4. Deploying Your Podcast App
Once you’ve built and tested your podcast app locally, it’s time to deploy it to a hosting service of your choice. Some popular options for deploying NEXT.js apps include Vercel, Netlify, and AWS Amplify. Here, we’ll outline the basic steps to deploy your app on Vercel:
4.1. Sign Up for Vercel
If you don’t already have an account, sign up for a Vercel account at https://vercel.com/.
4.2. Install the Vercel CLI
Install the Vercel CLI by running the following command:
bash npm install -g vercel
4.3. Deploy Your App
Navigate to your project directory in the terminal and run the following command:
bash vercel
Follow the prompts to deploy your app. Once the deployment is complete, you’ll receive a URL where your podcast app is hosted.
Conclusion
Congratulations! You’ve successfully built and deployed a podcast app using NEXT.js and the Podcaster library. Your app is now accessible to users worldwide, allowing them to explore and enjoy podcasts effortlessly.
Remember that you can further enhance your app by adding features such as search functionality, user profiles, and subscription options. Continuously improving your podcast app will help it stand out in the competitive podcasting market.
Now that you have the knowledge and skills to create your own podcast app, feel free to explore additional features and customization options to make it uniquely yours. Happy coding, and happy podcasting!
Table of Contents
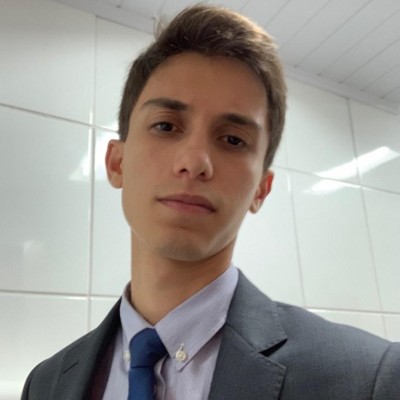
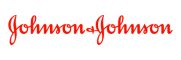