Building a Quiz App with NEXT.js and React Quiz Component
In the world of web development, creating interactive and engaging applications is a top priority. One popular way to captivate your audience is by building quiz applications. Whether it’s for educational purposes, entertainment, or even as a tool for collecting user data, quiz apps can be incredibly effective. In this tutorial, we’ll guide you through the process of building a quiz app using NEXT.js and the React Quiz Component. You’ll learn how to create quizzes with multiple-choice questions, track user scores, and provide immediate feedback. So, let’s dive in!
1. Prerequisites
Before we start building our quiz app, make sure you have the following prerequisites installed on your system:
- Node.js and npm (Node Package Manager)
- A code editor (e.g., Visual Studio Code)
- Basic knowledge of React and JavaScript
2. Setting Up the Project
To get started, we’ll set up a new NEXT.js project and install the required dependencies. Open your terminal and follow these steps:
Create a new NEXT.js project by running the following command:
bash npx create-next-app quiz-app
Change into the project directory:
bash cd quiz-app
Install the React Quiz Component package:
bash npm install react-quiz-component
Start the development server:
bash npm run dev
Your NEXT.js project is now set up and ready to build your quiz app.
3. Creating the Quiz Component
Let’s start by creating a reusable Quiz component using the React Quiz Component library. This component will handle the rendering of quiz questions, answer choices, and user interaction.
In your project directory, create a new file named Quiz.js inside the components folder. Here’s a basic structure for your Quiz.js file:
jsx import React from 'react'; import { Quiz } from 'react-quiz-component'; const quizData = [ { question: 'What is the capital of France?', answer: 'Paris', options: ['London', 'Berlin', 'Madrid', 'Paris'], }, { question: 'Which planet is known as the Red Planet?', answer: 'Mars', options: ['Earth', 'Mars', 'Venus', 'Jupiter'], }, // Add more quiz questions here ]; const QuizComponent = () => { return ( <div> <Quiz quiz={quizData} /> </div> ); }; export default QuizComponent;
In this code, we import the Quiz component from react-quiz-component and create an array quizData containing the quiz questions, correct answers, and answer choices. You can customize this array with your own questions and options.
Now, let’s include the QuizComponent in your pages/index.js file:
jsx import Head from 'next/head'; import QuizComponent from '../components/Quiz'; export default function Home() { return ( <div> <Head> <title>Quiz App</title> <meta name="description" content="Test your knowledge with our interactive quiz app." /> <link rel="icon" href="/favicon.ico" /> </Head> <main> <h1>Quiz App</h1> <QuizComponent /> </main> <footer> {/* Add your footer content here */} </footer> </div> ); }
Now, if you visit http://localhost:3000 in your browser, you should see your quiz app in action.
4. Customizing the Quiz Component
The react-quiz-component library provides a basic quiz layout, but you can customize it to match the look and feel of your app. Here are some ways to do that:
4.1. Styling
You can style the quiz component by applying CSS styles to the HTML elements within the Quiz component. For example, you can target the question text, answer choices, and buttons using CSS classes or inline styles.
jsx <Quiz quiz={quizData} customStyles={{ questionTitle: { color: 'blue', fontSize: '18px', fontWeight: 'bold', }, answerOption: { backgroundColor: 'lightgray', padding: '10px', borderRadius: '5px', }, }} />
4.2. Adding Images
To include images in your quiz questions or answer choices, you can use HTML <img> tags within the question’s question or an answer option’s option field in the quizData array.
jsx const quizData = [ { question: 'What is the capital of France?', answer: 'Paris', options: [ 'London', 'Berlin', 'Madrid', '<img src="/paris.jpg" alt="Paris" />', // Include an image ], }, // ... ];
4.3. Handling User Interaction
You can add functionality to handle user interactions, such as showing feedback after each question and keeping track of the user’s score. To do this, you can use React state and event handling.
jsx import React, { useState } from 'react'; import { Quiz } from 'react-quiz-component'; const quizData = [ { question: 'What is the capital of France?', answer: 'Paris', options: ['London', 'Berlin', 'Madrid', 'Paris'], }, { question: 'Which planet is known as the Red Planet?', answer: 'Mars', options: ['Earth', 'Mars', 'Venus', 'Jupiter'], }, // Add more quiz questions here ]; const QuizComponent = () => { const [score, setScore] = useState(0); const [currentQuestion, setCurrentQuestion] = useState(0); const handleAnswerClick = (answer) => { if (answer === quizData[currentQuestion].answer) { setScore(score + 1); } if (currentQuestion < quizData.length - 1) { setCurrentQuestion(currentQuestion + 1); } }; return ( <div> <Quiz quiz={quizData} currentQuestion={currentQuestion} customState={{ currentQuestion: setCurrentQuestion, score: setScore, }} callback={handleAnswerClick} /> <p>Score: {score}</p> </div> ); }; export default QuizComponent;
In this updated code, we use React state to keep track of the user’s score and the current question. We also create a handleAnswerClick function that is called when a user clicks an answer choice. If the selected answer is correct, we increment the score. We then update the current question, and the quiz component automatically displays the next question.
Conclusion
Congratulations! You’ve successfully built an interactive quiz app using NEXT.js and the React Quiz Component. You’ve learned how to create a quiz component, customize its appearance, and handle user interactions such as scoring and question progression.
This is just the beginning of what you can achieve with quiz apps. You can expand your app by adding features like timers, leaderboards, and user authentication. Feel free to experiment and make your quiz app even more engaging and entertaining.
Remember that building web applications is an iterative process, so don’t hesitate to explore additional libraries, tools, and techniques to enhance your quiz app further. Good luck with your project, and happy quizzing!
In this blog post, we’ve covered the process of building a quiz app with NEXT.js and the React Quiz Component. We began by setting up the project and then created a customizable quiz component. We discussed ways to style the quiz, add images, and handle user interactions. Building a quiz app is not only a great way to engage your audience but also an opportunity to showcase your creative and technical skills as a web developer. So, go ahead and start building your own quiz app today!
Table of Contents
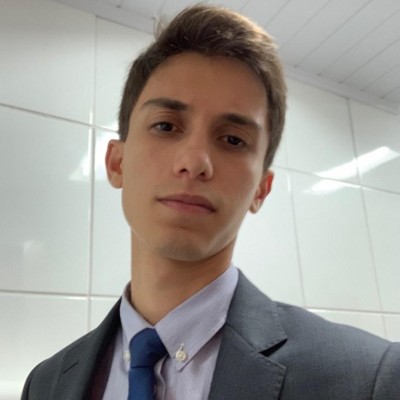
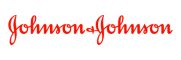