Building a Single-page Application with NEXT.js: React Router Integration
When it comes to building modern web applications, providing users with a seamless navigation experience is paramount. Single-page applications (SPAs) have gained immense popularity due to their ability to deliver dynamic content without page reloads. Combining this approach with the power of NEXT.js and the flexibility of React Router can elevate your application’s user experience to new heights.
In this guide, we’ll walk you through the process of integrating React Router into your NEXT.js application to create a smooth, single-page navigation flow. By following the steps outlined here, you’ll be able to take advantage of the best features of both libraries while crafting a more engaging and interactive user interface.
1. Introduction
Single-page applications offer a fluid and immersive browsing experience, where users can access different parts of the application without the need for full page reloads. Combining the power of NEXT.js, a popular React framework for server-rendered applications, with React Router, a powerful routing library for React, can result in a potent toolset for building SPAs that feel seamless and responsive.
2. Prerequisites
Before diving into the integration process, ensure you have the following prerequisites in place:
- Basic understanding of React.js
- Familiarity with NEXT.js concepts
- Node.js and npm installed on your system
3. Setting Up a NEXT.js Application
If you’re not already familiar with creating a NEXT.js application, here’s a quick rundown of the initial steps:
1. Open your terminal and run the following commands:
bash npx create-next-app your-spa-app cd your-spa-app
2. Once the installation is complete, you can start your development server with:
bash npm run dev
3. Your NEXT.js application should now be up and running on http://localhost:3000.
4. Integrating React Router
To integrate React Router into your NEXT.js application, follow these steps:
1. Install the required packages:
bash npm install react-router-dom
2. Open the _app.js file in the pages directory. This file acts as the wrapper for all pages in your application. Import the necessary components from React Router and modify the code as follows:
jsx import { BrowserRouter as Router } from 'react-router-dom'; function MyApp({ Component, pageProps }) { return ( <Router> <Component {...pageProps} /> </Router> ); } export default MyApp;
5. Creating Routes and Components
In this section, we’ll create the basic structure for the home, about, and contact pages.
5.1 Home Page
Inside the pages directory, create a new file named index.js.
Add the following code to set up the Home page component:
jsx import React from 'react'; const Home = () => { return ( <div> <h1>Welcome to the Home Page</h1> {/* Add your content here */} </div> ); }; export default Home;
5.2 About Page
Create a new file named about.js in the pages directory.
Add the following code for the About page component:
jsx import React from 'react'; const About = () => { return ( <div> <h1>About Us</h1> {/* Add your content here */} </div> ); }; export default About;
5.3 Contact Page
Similarly, create a new file named contact.js in the pages directory.
Add the following code for the Contact page component:
jsx import React from 'react'; const Contact = () => { return ( <div> <h1>Contact Us</h1> {/* Add your content here */} </div> ); }; export default Contact;
6. Navigating Between Routes
With the components in place, let’s implement navigation between these pages using React Router’s Link component.
Open the index.js file and add the following code to set up navigation links:
jsx import { Link } from 'react-router-dom'; // ... (existing code) const Home = () => { return ( <div> <h1>Welcome to the Home Page</h1> <nav> <ul> <li> <Link to="/">Home</Link> </li> <li> <Link to="/about">About</Link> </li> <li> <Link to="/contact">Contact</Link> </li> </ul> </nav> </div> ); }; export default Home;
Similarly, add navigation links in the about.js and contact.js files.
7. Enhancing User Experience
7.1 Highlighting Active Links
To provide visual feedback to users about the active route, you can style the active link differently.
1. Open your main CSS file (e.g., styles/globals.css) and add the following code:
css /* ... (existing styles) */ /* Style for active links */ nav a.active { font-weight: bold; }
2. Update the navigation links in the components to include the NavLink component, which automatically adds the active class to the active link:
jsx import { NavLink } from 'react-router-dom'; // ... (existing code) const Home = () => { return ( <div> <h1>Welcome to the Home Page</h1> <nav> <ul> <li> <NavLink exact to="/" activeClassName="active"> Home </NavLink> </li> <li> <NavLink to="/about" activeClassName="active"> About </NavLink> </li> <li> <NavLink to="/contact" activeClassName="active"> Contact </NavLink> </li> </ul> </nav> </div> ); };
7.2 Adding Transitions
Adding smooth transitions between route changes can enhance the overall user experience.
1. Install the react-transition-group package:
bash npm install react-transition-group
2. Wrap your route components in a CSSTransition component to apply transitions. Update the code in the index.js file as follows:
jsx import { CSSTransition } from 'react-transition-group'; // ... (existing code) const Home = () => { return ( <div> <h1>Welcome to the Home Page</h1> <nav> <ul> {/* ... (existing navigation links) */} </ul> </nav> <CSSTransition in={true} // Add your logic to determine when the transition should occur timeout={300} classNames="page" unmountOnExit > <div> {/* Your page content */} </div> </CSSTransition> </div> ); }; Add the corresponding CSS for the transition: css Copy code /* ... (existing styles) */ /* Transition animation */ .page-enter { opacity: 0; transform: translateY(10px); } .page-enter-active { opacity: 1; transform: translateY(0); transition: opacity 300ms, transform 300ms; } .page-exit { opacity: 1; transform: translateY(0); } .page-exit-active { opacity: 0; transform: translateY(-10px); transition: opacity 300ms, transform 300ms; }
8. Deployment and Final Thoughts
Congratulations! You’ve successfully integrated React Router into your NEXT.js application, creating a seamless single-page navigation experience. You can now deploy your application using platforms like Vercel, Netlify, or your preferred hosting service.
By combining the power of NEXT.js and React Router, you’ve opened up a world of possibilities for building SPAs with efficient routing, smooth transitions, and a user-friendly interface. As you continue to explore these libraries and their features, remember to keep user experience at the forefront of your design decisions. Happy coding!
Conclusion
In this guide, we’ve explored the process of integrating React Router into a NEXT.js application to create a single-page navigation experience. By following the steps outlined here, you can create a smoother and more engaging user experience for your web application. From setting up routes and components to enhancing navigation with active links and transitions, you now have the tools to build powerful and dynamic SPAs. Whether you’re a seasoned developer or just getting started, this integration can take your web development skills to new heights. So, why wait? Dive in, experiment, and craft exceptional single-page applications with NEXT.js and React Router.
Table of Contents
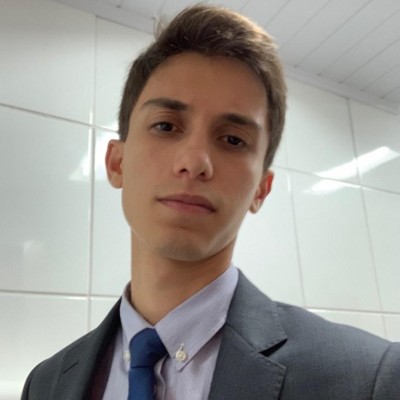
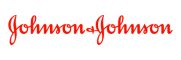