Building a Social Media Dashboard with NEXT.js and Firebase
In today’s digital age, social media plays a pivotal role in our personal and professional lives. As businesses and individuals alike strive to maintain a strong online presence, managing multiple social media platforms efficiently becomes crucial. This is where a social media dashboard comes into play – a centralized hub to monitor, analyze, and interact with various social media accounts. In this tutorial, we’ll guide you through the process of building your own social media dashboard using the NEXT.js framework and Firebase, a powerful backend-as-a-service platform. By combining these technologies, you’ll create a dynamic and responsive dashboard that provides real-time insights and enhances user engagement.
1. Prerequisites
Before we dive into the implementation, make sure you have a basic understanding of JavaScript, React, and Firebase. You’ll also need Node.js and npm (Node Package Manager) installed on your machine. If you’re new to these technologies, don’t worry – we’ll provide explanations and code samples along the way.
2. Setting Up the Project
Let’s start by setting up the project structure and installing the necessary dependencies.
2.1. Creating a NEXT.js App:
Open your terminal and run the following commands:
bash npx create-next-app social-media-dashboard cd social-media-dashboard
2.2. Installing Firebase:
Firebase offers various services that simplify backend development. To add Firebase to your project, run:
bash npm install firebase
2.3. Initializing Firebase:
To configure Firebase, you’ll need to create a Firebase project and obtain the configuration details. Visit the Firebase Console and follow the steps to create a new project. Once created, click on “Project settings” and then “Add Firebase to your web app.” Copy the configuration object provided.
In your project’s root directory, create a new file named firebaseConfig.js and paste the configuration object:
javascript // firebaseConfig.js const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; export default firebaseConfig;
Replace “YOUR_API_KEY”, “YOUR_AUTH_DOMAIN”, and other placeholders with your actual Firebase configuration.
3. Building the Social Media Dashboard
Now that we have our project set up, let’s start building the social media dashboard.
3.1. Authentication:
To ensure secure access to the dashboard, we’ll implement authentication using Firebase Authentication. This will allow users to sign up, sign in, and manage their accounts.
3.1.1. Setting up Authentication:
Open the firebaseConfig.js file and import Firebase:
javascript import firebase from "firebase/app"; import "firebase/auth"; // ... (rest of the config) firebase.initializeApp(firebaseConfig); export const auth = firebase.auth();
3.1.2. Creating the Authentication Components:
In the pages directory, create a new folder named auth. Inside this folder, create signup.js, signin.js, and signout.js files. We’ll only show the code for the signup.js file:
jsx import { useState } from "react"; import { auth } from "../../firebaseConfig"; export default function SignUp() { const [email, setEmail] = useState(""); const [password, setPassword] = useState(""); const handleSignUp = async () => { try { await auth.createUserWithEmailAndPassword(email, password); console.log("User registered successfully!"); } catch (error) { console.error("Error registering user:", error); } }; return ( <div> <h1>Sign Up</h1> <input type="email" placeholder="Email" value={email} onChange={(e) => setEmail(e.target.value)} /> <input type="password" placeholder="Password" value={password} onChange={(e) => setPassword(e.target.value)} /> <button onClick={handleSignUp}>Sign Up</button> </div> ); }
Repeat a similar process for signin.js and signout.js files, adapting the code to handle signing in and signing out.
3.2. Fetching Social Media Data:
Once users are authenticated, we need to fetch their social media data. For this example, let’s assume we’re fetching data from a Twitter account.
3.2.1. Setting up the Twitter API:
To interact with the Twitter API, you’ll need to create a developer account and create a Twitter App to obtain the necessary API keys and tokens.
3.2.2. Fetching Tweets:
In the pages directory, create a new folder named dashboard. Inside this folder, create a tweets.js file to display fetched tweets:
jsx import { useState, useEffect } from "react"; import { auth } from "../../firebaseConfig"; export default function Tweets() { const [tweets, setTweets] = useState([]); const user = auth.currentUser; useEffect(() => { // Fetch tweets using the Twitter API // Update the 'tweets' state with fetched data }, []); return ( <div> <h1>Welcome, {user.email}</h1> <h2>Your Tweets</h2> <ul> {tweets.map((tweet) => ( <li key={tweet.id}>{tweet.text}</li> ))} </ul> </div> ); }
3.3. Real-time Updates:
A great advantage of using Firebase is its real-time database capabilities. We can leverage this feature to provide users with real-time updates of their social media data.
3.3.1. Updating Tweets in Real Time:
Modify the tweets.js file to listen for real-time updates:
jsx // ... (imports and setup) export default function Tweets() { const [tweets, setTweets] = useState([]); const user = auth.currentUser; useEffect(() => { // Fetch tweets using the Twitter API // Update the 'tweets' state with fetched data const tweetsRef = firebase.database().ref(`users/${user.uid}/tweets`); tweetsRef.on("value", (snapshot) => { const tweetsData = snapshot.val(); const tweetsArray = Object.values(tweetsData || {}); setTweets(tweetsArray); }); return () => tweetsRef.off("value"); }, []); return ( // ... (JSX and UI) ); }
Conclusion
Congratulations! You’ve successfully built a social media dashboard using NEXT.js and Firebase. By integrating frontend and backend technologies, you’ve created a dynamic platform for users to authenticate, fetch, and monitor their social media data in real time. This project serves as a solid foundation that you can expand upon to add more features, such as additional social media platforms, analytics, and scheduling capabilities. As you continue to explore and learn, remember that the possibilities for enhancing user experiences in the realm of social media are limitless. Happy coding!
Table of Contents
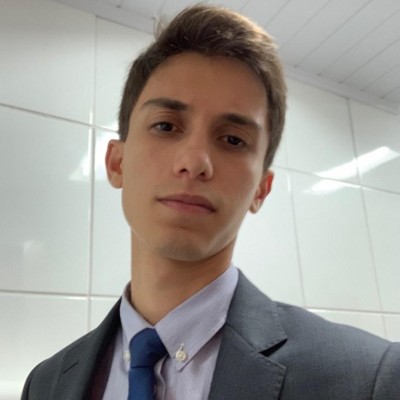
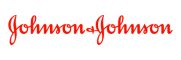