Mastering Next.js: A Comprehensive Guide to Building Dynamic Web Applications
Next.js is an open-source development framework built on top of Node.js and React.js, offering functionalities for server-side rendering, static site generation, and API routes. This robust tool provides developers a simple and fast way to build dynamic and powerful web applications, making it worthwhile to hire Next.js developers for your project needs.
In this blog post, we’ll examine the key features of Next.js and walk you through the process of creating a dynamic web application using this tool. Whether you’re considering to hire Next.js developers or want to dive in yourself, we’ll also showcase some illustrative examples to offer a hands-on perspective on its potential.
Why Choose Next.js?
Before we dive into the hands-on session, let’s look at why Next.js is a brilliant choice for dynamic web application development:
- Server-Side Rendering (SSR): Next.js automatically renders your pages on the server, improving performance and SEO.
- Static Site Generation (SSG): It allows developers to generate static HTML pages at build time, rather than per request, reducing server load.
- API Routes: Next.js comes with built-in support for creating API endpoints, eliminating the need for a separate server.
- Automatic Code Splitting: To improve loading times, Next.js automatically splits the code for each page.
- Built-In CSS and Sass Support: It provides support for CSS and Sass, eliminating the need for external libraries.
- Hot Code Reloading: Any changes made in the code are immediately reflected in the application, without a full page refresh.
Setting Up a Next.js Application
Getting started with a Next.js application is pretty straightforward. Here’s a step-by-step guide on setting up your first Next.js application:
First, ensure you have Node.js (10.13 or later) installed on your computer. Once done, create a new Next.js application using the `create-next-app` package. Navigate to the directory where you want your project to reside, and run the following command:
```bash npx create-next-app@latest my-next-app ```
Replace “my-next-app” with your desired project name. This command will create a new directory with your project name and set up a new Next.js application inside it.
After creating the application, navigate into your new project directory:
```bash cd my-next-app ```
Now, to start the development server, run:
```bash npm run dev ```
If everything is set up correctly, you should be able to see your application running at `http://localhost:3000`.
Building a Simple Blog with Next.js
For our hands-on example, we’ll be building a simple blog application with Next.js. This blog will feature dynamic pages for each blog post, created using server-side rendering.
Step 1: Creating Pages
In Next.js, a page is a React component exported from a `.js` file in the `pages` directory. Let’s create an `index.js` file inside the `pages` directory:
```jsx // pages/index.js import Link from 'next/link' export default function Home() { return ( <div> <h1>Welcome to My Blog!</h1> <ul> <li> <Link href="/posts/first-post"> <a>First Post</a> </Link> </li> </ul> </div> ) } ```
Here, we have created the home page for our blog, with a link to our first blog post.
Step 2: Creating Dynamic Pages
Next, let’s create the page for our first blog post. For dynamic pages, we use a feature called Dynamic Routes. Create a `[id].js` file inside the `pages/posts` directory:
```jsx // pages/posts/[id].js import { useRouter } from 'next/router' export default function Post() { const router = useRouter() const { id } = router.query return ( <div> <h1>Post: {id}</h1> <p>This is the blog post content.</p> </div> ) } ```
This page will render for any route that matches the pattern `/posts/:id`.
Step 3: Fetching Data for Pages
Next.js also allows us to fetch data on the server before rendering a page. Let’s fetch data for our blog posts from a hypothetical API endpoint.
Update your `[id].js` file as follows:
```jsx // pages/posts/[id].js import { useRouter } from 'next/router' export async function getServerSideProps(context) { const { params } = context const response = await fetch(`https://my-api.com/posts/${params.id}`) const data = await response.json() return { props: { post: data }, // Will be passed to the page component as props } } export default function Post({ post }) { return ( <div> <h1>Post: {post.title}</h1> <p>{post.content}</p> </div> ) } ```
In the `getServerSideProps` function, a crucial feature that Next.js developers often use, we fetch data from an API endpoint based on the page’s dynamic route parameter, `id`. This function showcases why you might want to hire Next.js developers for your project. The fetched data is then passed as props to the page component, where it is expertly rendered, again demonstrating the value of having skilled Next.js developers on your team.
Conclusion
Next.js is a robust framework that provides easy-to-use, yet powerful features for building dynamic web applications. With its server-side rendering capabilities, static site generation, and API routes, it brings to the table the ideal toolkit for both simple and complex applications alike. This breadth of functionality makes it beneficial to hire Next.js developers for your project needs.
Our example of a blog application barely scratches the surface of what you can do with Next.js. The framework also offers features like image optimization, internationalized routing, and more, making it an excellent choice for modern web development.
So, if you’re looking to start a new web application project, consider hiring Next.js developers or give Next.js a try yourself. It might just be the tool you’re looking for to bring your vision to life.
Table of Contents
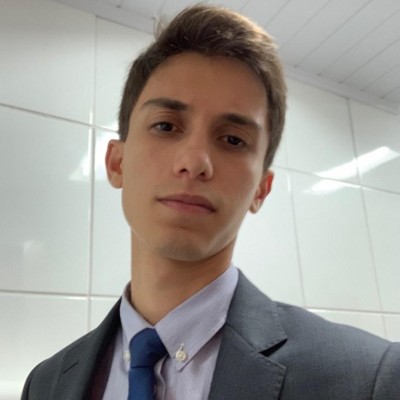
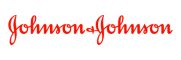