Exploring NEXT.js Optimizations: Code Splitting and Lazy Loading
In today’s fast-paced digital world, user experience is paramount. No one likes a slow-loading website. Visitors expect web pages to load quickly and efficiently, regardless of the device they are using. To meet these expectations, web developers are constantly seeking ways to optimize their applications and make them as fast and responsive as possible.
One powerful tool in the arsenal of modern web developers is Next.js, a React framework that simplifies building server-rendered React applications. Next.js not only offers a delightful developer experience but also provides several features for optimizing the performance of your web applications. In this blog post, we will dive deep into two crucial optimization techniques: code splitting and lazy loading.
1. What is Next.js?
Before we delve into optimization techniques, let’s briefly understand what Next.js is and why it’s a popular choice among web developers.
Next.js is an open-source React framework that simplifies the process of building modern, server-rendered React applications. It’s known for its developer-friendly features, such as automatic code splitting, server-side rendering, and routing out of the box. These features make Next.js an excellent choice for building high-performance web applications.
1.1. Why Optimize Your Next.js Application?
Optimizing your Next.js application is crucial for several reasons:
- Faster Load Times: Optimized applications load faster, providing a better user experience and reducing bounce rates.
- Improved SEO: Faster load times positively impact your website’s search engine ranking, increasing its visibility.
- Reduced Bandwidth Usage: Smaller bundles mean less data transfer, saving bandwidth for both users and server resources.
- Enhanced User Experience: A responsive and fast website keeps users engaged and satisfied.
Now that we understand the importance of optimization, let’s explore code splitting and lazy loading in more detail.
2. Code Splitting
2.1. What is Code Splitting?
Code splitting is a technique used to improve a web application’s performance by splitting the code into smaller, more manageable chunks. Instead of loading the entire JavaScript bundle when a user accesses a web page, code splitting allows you to load only the code required for that specific page.
This technique is particularly beneficial for large applications with multiple routes or pages. Without code splitting, users would have to download the entire application code, even if they only need a fraction of it. Code splitting reduces the initial load time and improves the perceived performance of your application.
2.2. Implementing Code Splitting in Next.js
Next.js makes implementing code splitting a breeze. By default, Next.js automatically code splits your application at the route level. This means that each page in your application becomes a separate JavaScript bundle.
Here’s a code snippet to illustrate how easy it is to create a dynamically imported module in a Next.js application:
javascript // pages/index.js import dynamic from 'next/dynamic'; const DynamicComponent = dynamic(() => import('../components/DynamicComponent')); function HomePage() { return ( <div> <p>This is a Next.js page with code splitting.</p> <DynamicComponent /> </div> ); } export default HomePage;
In this example, the dynamic function is used to import the DynamicComponent lazily. This means that DynamicComponent will only be loaded when it’s actually needed, improving the page’s initial load time.
2.3. Benefits of Code Splitting
Code splitting in Next.js offers several benefits:
- Faster Initial Page Load: Only the necessary JavaScript is loaded initially, reducing the time it takes for the page to become interactive.
- Smaller Bundle Sizes: Smaller bundles are quicker to download, making your application feel snappier.
- Improved User Experience: Users see content faster, leading to higher user satisfaction.
- Better Resource Management: Code splitting helps distribute the load on your server and client, optimizing resource usage.
3. Lazy Loading
3.1. What is Lazy Loading?
Lazy loading is a technique that defers the loading of non-essential resources until they are needed. In the context of web development, this often refers to images, videos, or additional JavaScript modules that are not required for the initial page load.
Lazy loading is particularly useful for improving the performance of web pages with extensive media content or interactive features that are only triggered by user actions.
3.2. Implementing Lazy Loading in Next.js
Next.js provides built-in support for lazy loading images using the next/image package. Here’s an example of lazy loading an image in a Next.js application:
javascript // components/ImageComponent.js import Image from 'next/image'; function LazyLoadedImage() { return ( <div> <Image src="/path/to/your/image.jpg" alt="Description of the image" width={500} height={300} /> </div> ); } export default LazyLoadedImage;
In this example, the Image component from next/image automatically handles lazy loading for you, ensuring that the image is loaded only when it becomes visible in the viewport.
3.3. Benefits of Lazy Loading
Lazy loading offers several advantages for web performance:
- Reduced Initial Load Time: Non-essential resources are deferred, leading to faster initial page rendering.
- Improved Page Speed: Faster loading times result in a snappier and more responsive user experience.
- Bandwidth Savings: Unused resources are not downloaded, saving bandwidth for users and server resources.
- Lower Bounce Rates: Improved user experience reduces the likelihood of users leaving your site prematurely.
4. Combining Code Splitting and Lazy Loading
4.1. When to Combine Both Techniques
While code splitting and lazy loading are powerful on their own, they can be even more effective when used together. Combining these techniques is especially beneficial in scenarios where you have a complex Next.js application with many routes, media content, and interactive components.
Here are some scenarios where combining code splitting and lazy loading can be advantageous:
- E-commerce Websites: Load product listings and details lazily while keeping the main navigation responsive.
- News Portals: Delay the loading of articles and images until the user scrolls to them.
- Web Apps: Load complex user interface components on-demand to optimize the initial load time.
4.2. How to Implement Both Techniques Together
To combine code splitting and lazy loading in Next.js, follow these steps:
- Identify the parts of your application that can benefit from code splitting, such as routes and components.
- Implement code splitting for those parts using dynamic imports, as shown in the previous code splitting example.
- Identify resources that can be lazily loaded, such as images, videos, or additional JavaScript modules.
- Implement lazy loading for those resources using appropriate Next.js features, such as the next/image package for images.
- Test your application to ensure that resources are loaded efficiently and that the user experience is improved.
By combining code splitting and lazy loading strategically, you can create a highly performant and responsive Next.js application that meets the expectations of modern web users.
5. Measuring the Impact
5.1. Analyzing Performance with Lighthouse
After implementing code splitting and lazy loading in your Next.js application, it’s essential to measure the impact of these optimizations. Google’s Lighthouse tool is a valuable resource for assessing web performance.
Here’s how you can use Lighthouse to evaluate your Next.js application:
- Open your website in Google Chrome.
- Right-click on the page and select “Inspect.”
- In the DevTools panel, go to the “Audits” tab.
- Click on “Run audits.”
- Lighthouse will analyze your website and provide a performance score along with recommendations for improvement.
Pay attention to the performance score and the specific suggestions provided by Lighthouse. This will help you fine-tune your code splitting and lazy loading implementations for optimal results.
5.2. Monitoring Bundle Size with Webpack Bundle Analyzer
To gain a deeper understanding of your application’s bundle size and dependencies, you can use tools like Webpack Bundle Analyzer. This tool provides a visual representation of your application’s webpack bundle, making it easier to identify large dependencies and areas for improvement.
Here’s how to use Webpack Bundle Analyzer with Next.js:
Install the webpack-bundle-analyzer package:
bash npm install --save-dev webpack-bundle-analyzer
Add a custom webpack configuration to your Next.js project. Create a webpack.config.js file in your project root if you don’t already have one, and configure it as follows:
javascript // webpack.config.js const { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer'); module.exports = { webpack: (config, { webpack }) => { config.plugins.push( new BundleAnalyzerPlugin({ analyzerMode: 'static', reportFilename: 'bundle-analyzer-report.html', }) ); return config; }, };
Run your Next.js application with the following command:
bash next build && next start
Access the Webpack Bundle Analyzer report by opening the generated bundle-analyzer-report.html file in your browser. This report will provide insights into your application’s bundle size and help you identify areas where code splitting and lazy loading can be further optimized.
6. Best Practices for Next.js Optimization
To maximize the benefits of code splitting and lazy loading in Next.js, consider the following best practices:
- Prioritize critical code: Identify and load critical code first to ensure a fast initial render.
- Profile and analyze: Use performance profiling tools to identify bottlenecks and areas for improvement.
- Optimize images: Compress and resize images to reduce their size and improve loading times.
- Use the Link component: When navigating between pages, use Next.js’s Link component to enable prefetching and improve user experience.
- Keep dependencies minimal: Be mindful of third-party dependencies and their impact on bundle size.
- Regularly test and monitor: Continuously measure and analyze your application’s performance to identify and address issues promptly.
Conclusion
In the world of web development, user experience is paramount. Slow-loading websites can drive users away and impact your business. Next.js, with its built-in support for code splitting and lazy loading, offers powerful tools to enhance the performance of your applications.
By implementing code splitting, you can reduce initial load times and create a more responsive user experience. Lazy loading allows you to defer the loading of non-essential resources, further improving performance.
When used together strategically, code splitting and lazy loading can take your Next.js applications to the next level, meeting the expectations of modern web users for fast, responsive, and engaging experiences. Don’t forget to measure the impact of your optimizations with tools like Lighthouse and Webpack Bundle Analyzer and follow best practices to ensure your Next.js applications are always at their best.
Table of Contents
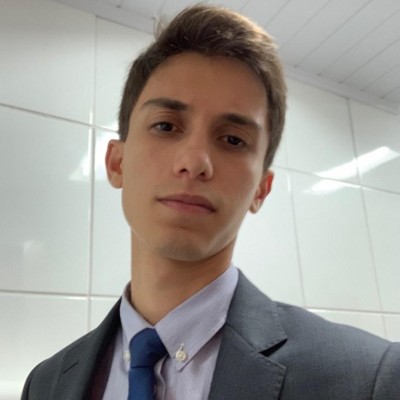
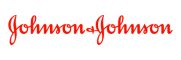