Implementing Data Visualization in NEXT.js with D3.js
In the world of web development, data visualization plays a crucial role in conveying complex information in an understandable and engaging manner. If you’re building a web application with NEXT.js and looking to add data visualizations, D3.js, short for Data-Driven Documents, is a JavaScript library that simplifies the process of creating data visualizations in web applications. In this guide, we will explore how to integrate D3.js into a NEXT.js project and create interactive data visualizations from scratch.
1. Setting Up Your NEXT.js Project
Before diving into D3.js, let’s set up our NEXT.js project if you haven’t already. If you’re new to NEXT.js, you can follow these steps to create a new project:
bash npx create-next-app my-data-viz-app cd my-data-viz-app npm install npm run dev
This will create a basic NEXT.js application that we will enhance with D3.js-powered data visualizations.
2. Understanding D3.js
D3.js is a JavaScript library that allows you to bind data to Document Object Model (DOM) elements and apply data-driven transformations to the document. In simpler terms, it helps you create dynamic and interactive visualizations by associating data with elements on your web page.
2.1. Key Concepts of D3.js
Before we proceed, let’s grasp some key concepts of D3.js:
- Selections: D3.js allows you to select elements in the DOM and bind data to them.
- Data Binding: You can associate data with selected elements, creating a relationship between the data and the visual representation.
- Scales: D3.js provides scale functions to map data values to visual attributes, such as position and size.
- Axes: For many visualizations, you’ll want to add axes to label and provide context to your data.
- Transitions: D3.js enables smooth transitions between different states of your visualization.
- Enter-Update-Exit Pattern: This pattern is fundamental to D3.js and is used to create, update, and remove elements based on changes in your data.
Now that we have a basic understanding of D3.js, let’s move on to creating our first data visualization.
3. Creating Your First Data Visualization
In this section, we’ll walk through the process of creating a simple bar chart using D3.js within a NEXT.js application.
3.1. Installing D3.js
First, we need to install D3.js as a dependency in our project:
bash npm install d3
3.2. Creating an SVG Container
Let’s start by creating an SVG container in which we’ll draw our bar chart. In your React component, import D3.js and create an SVG element:
jsx import React, { useEffect, useRef } from 'react'; import * as d3 from 'd3'; function BarChart() { const svgRef = useRef(); useEffect(() => { // Create an SVG container const svg = d3.select(svgRef.current) .append('svg') .attr('width', 500) .attr('height', 300); // Add your chart elements here }, []); return ( <div> <h2>Bar Chart</h2> <div ref={svgRef}></div> </div> ); } export default BarChart;
3.3. Binding Data to Elements
To create a bar chart, you’ll need some data. For simplicity, let’s use an array of numbers to represent the heights of the bars. We can then bind this data to SVG rectangle elements:
jsx // ... function BarChart() { const svgRef = useRef(); const data = [10, 30, 20, 50, 40]; // Sample data useEffect(() => { // Create an SVG container const svg = d3.select(svgRef.current) .append('svg') .attr('width', 500) .attr('height', 300); // Bind data to rectangles svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('x', (d, i) => i * 60) .attr('y', (d) => 300 - d * 5) .attr('width', 50) .attr('height', (d) => d * 5) .attr('fill', 'blue'); }, []); // ... }
In this code, we bind the data to SVG rectangles and use attributes like x, y, width, height, and fill to define their positions and appearance.
3.4. Scaling and Axes
While the above example is a basic bar chart, real-world data often varies significantly. You should use scales and axes to ensure your visualizations are proportionate and informative. Here’s how to add scales and axes to our bar chart:
jsx // ... function BarChart() { const svgRef = useRef(); const data = [10, 30, 20, 50, 40]; const width = 500; const height = 300; useEffect(() => { // Create an SVG container const svg = d3.select(svgRef.current) .append('svg') .attr('width', width) .attr('height', height); // Define scales const xScale = d3.scaleBand() .domain(data.map((_, i) => i)) .range([0, width]) .padding(0.1); const yScale = d3.scaleLinear() .domain([0, d3.max(data)]) .nice() .range([height, 0]); // Add bars svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('x', (_, i) => xScale(i)) .attr('y', (d) => yScale(d)) .attr('width', xScale.bandwidth()) .attr('height', (d) => height - yScale(d)) .attr('fill', 'blue'); // Add x-axis svg.append('g') .attr('transform', `translate(0,${height})`) .call(d3.axisBottom(xScale)); // Add y-axis svg.append('g') .call(d3.axisLeft(yScale)); }, []); // ... }
Now, our bar chart has proper scaling and labeled axes.
4. Customizing Your Data Visualization
Creating a basic data visualization is just the beginning. Let’s explore how to customize and enhance your visualization further.
4.1. Styling with CSS
You can style your data visualization by adding CSS classes to SVG elements. For example, you can define CSS rules to change the color of bars or add animations for a more dynamic look. Here’s a simple CSS example:
css /* styles.css */ .bar { fill: steelblue; transition: fill 0.3s; } .bar:hover { fill: orange; }
Apply the class to your bars like this:
jsx // ... function BarChart() { // ... useEffect(() => { // ... // Add bars with CSS class svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('x', (_, i) => xScale(i)) .attr('y', (d) => yScale(d)) .attr('width', xScale.bandwidth()) .attr('height', (d) => height - yScale(d)) .attr('class', 'bar'); // Apply the CSS class }, []); // ... }
4.2. Adding Interactivity
Interactivity can greatly enhance user engagement. D3.js provides easy ways to add interactions like tooltips or animations. Here’s how to add a simple tooltip on hover:
jsx // ... function BarChart() { // ... useEffect(() => { // ... // Add bars with tooltips svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('x', (_, i) => xScale(i)) .attr('y', (d) => yScale(d)) .attr('width', xScale.bandwidth()) .attr('height', (d) => height - yScale(d)) .attr('class', 'bar') .on('mouseover', (event, d) => { // Show tooltip on hover tooltip.html(`Value: ${d}`) .style('visibility', 'visible') .style('top', `${event.pageY}px`) .style('left', `${event.pageX}px`); }) .on('mouseout', () => { // Hide tooltip on mouseout tooltip.style('visibility', 'hidden'); }); // Create tooltip element const tooltip = d3.select(svgRef.current) .append('div') .attr('class', 'tooltip') .style('visibility', 'hidden'); }, []); // ... }
With these enhancements, your bar chart becomes more engaging and user-friendly.
5. Integrating D3.js with NEXT.js
Now that you’ve created your data visualization with D3.js, let’s integrate it seamlessly into your NEXT.js application.
5.1. Using React Refs
In the previous examples, we used a React ref (svgRef) to reference the DOM element where our chart is rendered. This approach ensures compatibility with React’s lifecycle and is essential for integrating D3.js with React-based frameworks like NEXT.js.
5.2. Fetching Data
In real-world applications, you’ll often need to fetch data from an API or other sources. In a NEXT.js app, you can use the built-in getServerSideProps or getStaticProps functions to fetch data on the server side before rendering the page. You can then pass this data as props to your D3.js-powered components.
Here’s an example of how you might fetch data and pass it to your BarChart component:
jsx // pages/index.js import BarChart from '../components/BarChart'; function Home({ data }) { return ( <div> <h1>Data Visualization with D3.js in NEXT.js</h1> <BarChart data={data} /> </div> ); } export async function getServerSideProps() { // Fetch data from your API or other sources const data = await fetchData(); return { props: { data, }, }; } export default Home;
5.3. Updating the Visualization
When your data changes, you can update the visualization by passing the new data as props to your D3.js component. Inside your component, use the componentDidUpdate lifecycle method (or useEffect with dependencies) to handle updates and transitions gracefully.
6. Deploying Your NEXT.js Application
Once you’ve created and integrated your data visualizations, it’s time to deploy your NEXT.js application. You can choose from various hosting platforms like Vercel, Netlify, or AWS Amplify, depending on your preferences and requirements. Follow the deployment instructions provided by your chosen hosting service to make your application accessible on the web.
Conclusion
In this guide, we’ve explored the process of implementing data visualizations in a NEXT.js application using D3.js. We started with the basics, creating a simple bar chart, and then moved on to customizing it with CSS styles and interactivity. We also discussed the integration of D3.js with NEXT.js, including data fetching and updating visualizations.
By combining the power of D3.js and the flexibility of NEXT.js, you can create dynamic and engaging data-driven web applications that provide valuable insights to your users. Now, armed with this knowledge, you’re ready to embark on your journey to build stunning data visualizations in your NEXT.js projects. Happy coding!
Table of Contents
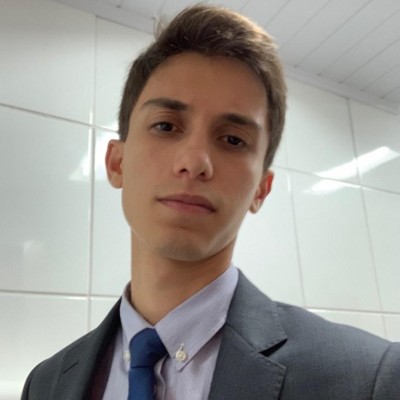
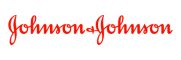