Enhancing Web Design with Next.js: A Deep Dive into CSS-in-JS and Styled Components
Next.js, developed by Vercel, has surged in popularity among developers and led to an increase in demand to hire Next.js developers. This unique approach to developing React applications, with its simple server-side rendering and static site generation, are just a few of its many appealing features. In addition, the design capabilities of Next.js don’t stop there. This blog post delves into how you, or the Next.js developers you hire, can style your Next.js applications to the next level using CSS-in-JS and styled components. As a result, hiring skilled Next.js developers can help you leverage these capabilities to their full potential.
Understanding CSS-in-JS
Before we delve into the nitty-gritty of styling in Next.js, it’s crucial to understand the concept of CSS-in-JS. Traditional CSS (Cascading Style Sheets) has been the standard for styling web content. Yet, as web development evolved, the need for more dynamic, component-based styles emerged. CSS-in-JS, a styling technique where CSS is composed using JavaScript, was introduced to address these challenges.
This method involves writing your styles in JavaScript files, which has the added benefit of offering the full power of JS to your stylesheets. Variables, conditionals, and functions can be leveraged to create dynamic styles that react to your application’s state.
The Power of Styled Components
Enter Styled Components. This library, built for both React and React Native, opens the door to innovative component-level styles in your application that are written with a blend of JavaScript and CSS. Styled components utilize tagged template literals to generate actual CSS in your JS files, thus providing a robust toolkit for Next.js developers.
Now, whether you’re an experienced Next.js developer or looking to hire Next.js developers for your project, it’s key to understand the implementation of Styled Components in a Next.js project. Let’s take a closer look at how this can be accomplished.
Setting up Styled Components in Next.js
To get started, we will install Styled Components in our Next.js project:
```bash npm install --save styled-components ```
Once we have installed styled-components, we can import it into our files like so:
```javascript import styled from 'styled-components'; ```
And now we can create a styled component:
```javascript const RedText = styled.h1` color: red; `; ```
In the above snippet, `RedText` is now a React component that we can use like any other component in our application, and it will have a red text color.
```javascript export default function HomePage() { return ( <div> <RedText>Hello, world!</RedText> </div> ); } ```
The resulting HTML will look like this:
```html <div> <h1 class="classNameGeneratedByStyledComponents">Hello, world!</h1> </div> ```
The CSS generated by Styled Components is attached to the head of the document, scoped to the component, and the class name is generated automatically, avoiding naming clashes.
Dynamic Styling With Props
Styled components allow us to pass props and dynamically adjust our styles:
```javascript const ColorText = styled.h1` color: ${props => props.color || "black"}; `; export default function HomePage() { return ( <div> <ColorText color="purple">I am a purple text!</ColorText> <ColorText>I am a black text!</ColorText> </div> ); } ```
In this example, the `ColorText` component’s color is determined by the `color` prop. If no `color` prop is passed, the text will default to black.
Styled Components and Server-Side Rendering
Next.js excels at server-side rendering, but we need to ensure our styles load correctly. To fix this, we need to use the `ServerStyleSheet` and `StyleSheetManager` from Styled Components.
Let’s adjust our `_document.js` file to handle server-side rendering:
```javascript import Document from 'next/document' import { ServerStyleSheet } from 'styled-components' export default class MyDocument extends Document { static async getInitialProps(ctx) { const sheet = new ServerStyleSheet() const originalRenderPage = ctx.renderPage try { ctx.renderPage = () => originalRenderPage({ enhanceApp: (App) => (props) => sheet.collectStyles(<App {...props} />), }) const initialProps = await Document.getInitialProps(ctx) return { ...initialProps, styles: ( <> {initialProps.styles} {sheet.getStyleElement()} </> ), } } finally { sheet.seal() } } } ```
The above snippet collects all of our styles from our components and puts them into one server-side stylesheet.
Global Styles in Styled Components
What if you want to apply some global styles, like a global font or background color? Styled Components has you covered there as well. The `createGlobalStyle` function allows us to inject global styles:
```javascript import { createGlobalStyle } from 'styled-components'; const GlobalStyle = createGlobalStyle` body { margin: 0; padding: 0; font-family: Open-Sans, Helvetica, Sans-Serif; background-color: papayawhip; } `; // in your component export default function HomePage() { return ( <div> <GlobalStyle /> <RedText>Hello, world!</RedText> </div> ); } ```
In the above example, we use `createGlobalStyle` to set global styles for the `body` tag. Don’t forget to include `<GlobalStyle />` in your component!
Conclusion
The combination of Next.js with Styled Components offers a robust solution for building and styling modern web applications. This combination becomes particularly powerful when you hire Next.js developers with a deep understanding of these tools. By doing so, you gain the flexibility of CSS-in-JS, enabling more dynamic, component-scoped styles, while leveraging the power of server-side rendering from Next.js. Whether you’re a Next.js developer yourself or looking to hire Next.js developers, understanding and utilizing these libraries can provide you with the tools to craft truly next-level applications.
Recommended Readings
- Styled-components official documentation (https://styled-components.com/)
- Next.js official documentation (https://nextjs.org/docs)
- CSS-in-JS: Benefits, Drawbacks, and Tooling (https://www.robinwieruch.de/react-css-in-js)
By diving into CSS-in-JS and Styled Components, we hope you have found the inspiration to take your styling in Next.js to new heights. The modern web demands both functionality and aesthetically pleasing design, and with these tools at your disposal, you can confidently deliver both. Happy coding!
Table of Contents
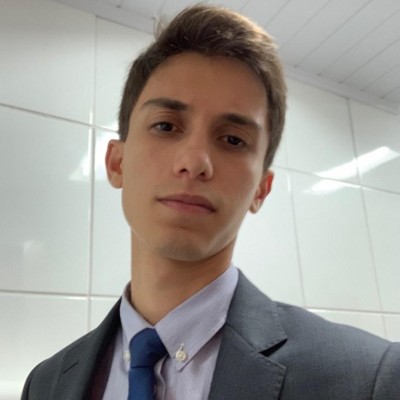
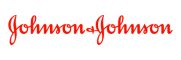