Using NEXT.js with Firebase Firestore: Real-time Database Integration
In today’s rapidly evolving web development landscape, building dynamic and real-time web applications has become increasingly important. To achieve this, developers often turn to technologies like Firebase Firestore and NEXT.js. Firebase Firestore is a real-time NoSQL database offered by Google, while NEXT.js is a popular React framework that enables server-side rendering and seamless client-side routing
In this blog post, we will walk through the process of integrating Firebase Firestore with NEXT.js. We’ll start with setting up Firebase Firestore, explore data modeling, and perform CRUD (Create, Read, Update, Delete) operations on the database. Additionally, we’ll delve into the fascinating world of real-time updates with Firestore, enabling your web application to respond dynamically to changes in the database.
Are you ready to unlock the potential of real-time data in your web applications? Let’s get started!
1. Setting up Firebase Firestore
1.1. Creating a Firebase Project
To begin, you’ll need a Firebase project. If you don’t already have one, follow these steps:
- Go to the Firebase Console.
- Click on “Add Project” and follow the on-screen instructions to create a new project.
- Once your project is created, click on it in the Firebase Console.
1.2. Initializing Firebase in NEXT.js
Now that you have a Firebase project, it’s time to integrate it into your NEXT.js application. You can do this by initializing Firebase in your project.
First, make sure you have the Firebase CLI installed. If you don’t have it, you can install it globally using npm:
bash npm install -g firebase-tools
Now, navigate to your NEXT.js project directory and run the following command:
bash firebase init
Follow the prompts to set up Firebase in your project. This will include selecting the Firebase project you created earlier and choosing Firestore as one of the Firebase features to set up.
Once Firebase is initialized, you’ll have a firebaseConfig object that contains your Firebase project’s configuration details. You’ll need this configuration to connect your NEXT.js application to Firebase Firestore.
2. Data Modeling with Firestore
2.1. Collections and Documents
Firestore organizes data into collections, which are analogous to tables in traditional relational databases, and documents, which are individual records within those collections. Data is stored in JSON-like format, making it flexible and easy to work with.
Before we dive into coding, let’s define the data structure for our application. For example, if you’re building a real-time chat application, you might have a collection called “messages,” where each document represents a message with fields like “text,” “sender,” and “timestamp.”
2.2. Firestore Data Types
Firestore supports various data types, including strings, numbers, booleans, timestamps, and more. Understanding these data types is crucial when defining your data schema. Here are some common Firestore data types:
- String: Represents a text value.
- Number: Represents a numeric value.
- Boolean: Represents a true or false value.
- Timestamp: Represents a point in time.
- Array: Represents an ordered list of values.
- Map: Represents a nested set of key-value pairs.
Now that you have a basic understanding of Firestore’s data structure and types, let’s move on to CRUD operations.
3. CRUD Operations with Firestore
3.1. Creating Data
To create data in Firestore, you can use the add method on a collection reference. Here’s an example of adding a new message to the “messages” collection:
javascript import firebase from 'firebase/app'; import 'firebase/firestore'; // Get a reference to the Firestore database const db = firebase.firestore(); // Reference to the "messages" collection const messagesRef = db.collection('messages'); // Data to be added const newMessage = { text: 'Hello, Firestore!', sender: 'John Doe', timestamp: new Date(), }; // Add the new message to the collection messagesRef.add(newMessage) .then((docRef) => { console.log('Document written with ID: ', docRef.id); }) .catch((error) => { console.error('Error adding document: ', error); });
In this code, we import Firebase and Firestore, get a reference to the Firestore database, and then create a reference to the “messages” collection. We define the data we want to add as an object and use the add method to insert it into the collection.
3.2. Reading Data
Reading data from Firestore is straightforward. You can use the get method to retrieve documents from a collection. For example, to fetch all messages from the “messages” collection:
javascript // Fetch all messages from the "messages" collection messagesRef.get() .then((querySnapshot) => { querySnapshot.forEach((doc) => { console.log(`${doc.id} => ${doc.data().text}`); }); }) .catch((error) => { console.error('Error getting documents: ', error); });
In this code, we use the get method to fetch all documents in the “messages” collection and then iterate through the result using forEach. You can access document fields using the data method.
3.3. Updating Data
To update data in Firestore, you can use the update method on a document reference. Let’s say you want to update the text of a message:
javascript // Reference to a specific message document const messageDocRef = messagesRef.doc('messageId'); // Update the message's text messageDocRef.update({ text: 'Updated message text', }) .then(() => { console.log('Document successfully updated'); }) .catch((error) => { console.error('Error updating document: ', error); });
In this example, we first obtain a reference to the specific document we want to update using doc(‘messageId’). Then, we use the update method to modify the document’s data.
3.4. Deleting Data
Deleting data in Firestore is achieved using the delete method on a document reference:
javascript // Reference to a specific message document const messageDocRef = messagesRef.doc('messageId'); // Delete the message messageDocRef.delete() .then(() => { console.log('Document successfully deleted'); }) .catch((error) => { console.error('Error deleting document: ', error); });
In this code, we obtain a reference to the document we want to delete and then call the delete method on it.
4. Real-time Updates with Firestore
One of the most powerful features of Firestore is its real-time synchronization. Firestore provides a way to listen for changes in data and react to those changes in real-time.
4.1. Listening for Changes
You can listen for changes to a document or a query by using the onSnapshot method. This method sets up a persistent connection to Firestore and invokes a callback whenever the data changes.
javascript // Listen for real-time changes to a specific message document messageDocRef.onSnapshot((doc) => { if (doc.exists) { console.log('Current data: ', doc.data()); } else { console.log('Document does not exist'); } });
In this example, we listen for changes to a specific message document. When the document changes, the provided callback is executed with the updated data. You can also check if the document exists using doc.exists.
4.2. Reacting to Real-time Data
Real-time updates open up numerous possibilities for creating dynamic web applications. For instance, you can build a chat application where new messages instantly appear in the chat window as they are sent, or create a collaborative whiteboard where changes made by one user are immediately visible to others.
To demonstrate real-time updates in a NEXT.js app, let’s build a simple real-time chat application.
5. Building a Sample NEXT.js App
5.1. Setting Up the NEXT.js Project
If you haven’t already created a NEXT.js project, you can do so using the following commands:
bash npx create-next-app my-realtime-app cd my-realtime-app npm install firebase
This will set up a new NEXT.js project and install the Firebase package we’ll need.
5.2. Integrating Firestore
To integrate Firestore with your NEXT.js app, you’ll need to use the firebaseConfig object we obtained earlier when initializing Firebase. Import it into your project:
javascript // Import the Firebase configuration import { firebaseConfig } from '../path-to-your-firebase-config'; import firebase from 'firebase/app'; import 'firebase/firestore'; // Initialize Firebase with the configuration if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); } // Get a reference to the Firestore database const db = firebase.firestore();
Ensure you replace ‘../path-to-your-firebase-config’ with the actual path to your Firebase configuration file.
5.3. Displaying Real-time Data
Now, let’s create a simple chat application that displays messages in real-time as they are added to Firestore. We’ll use React components and the Firestore real-time listener to achieve this.
javascript import React, { useEffect, useState } from 'react'; function ChatApp() { const [messages, setMessages] = useState([]); const messagesRef = db.collection('messages'); useEffect(() => { // Set up a real-time listener for messages const unsubscribe = messagesRef.orderBy('timestamp').onSnapshot((snapshot) => { const updatedMessages = []; snapshot.forEach((doc) => { updatedMessages.push(doc.data()); }); setMessages(updatedMessages); }); // Clean up the listener when the component unmounts return () => unsubscribe(); }, []); const addMessage = () => { // Add a new message to Firestore messagesRef.add({ text: 'Hello, World!', sender: 'User', timestamp: new Date(), }); }; return ( <div> <h1>Real-time Chat App</h1> <div className="chat-box"> {messages.map((message, index) => ( <div key={index} className="message"> <strong>{message.sender}: </strong> {message.text} </div> ))} </div> <button onClick={addMessage}>Send Message</button> </div> ); } export default ChatApp;
In this code, we use React’s useState and useEffect hooks to manage the state of the messages and set up a real-time listener for the “messages” collection. When new messages are added or existing ones are updated, the component re-renders with the latest data.
To test the chat app, you can render the ChatApp component in your NEXT.js application.
Conclusion
In this blog post, we explored how to integrate Firebase Firestore with NEXT.js to create web applications with real-time database capabilities. We started by setting up a Firebase project and initializing it in our NEXT.js project. Then, we delved into data modeling, CRUD operations, and real-time updates with Firestore.
By combining the power of Firestore’s real-time synchronization and NEXT.js’s server-side rendering, you can build dynamic and responsive web applications that engage users with up-to-the-second data updates.
Now that you’ve learned the fundamentals of using Firestore with NEXT.js, you’re well-equipped to create your own real-time web applications and bring your ideas to life. So go ahead, start building, and unlock the potential of real-time data in your projects! Happy coding!
Table of Contents
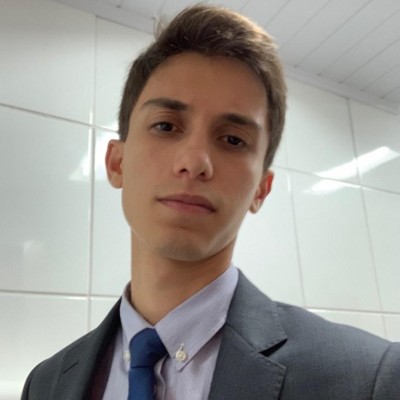
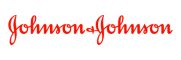