Data-Driven Decisions: Integrating Google Analytics with NEXT.js
In the fast-paced world of web development, staying ahead of the competition means not only creating a fantastic user experience but also understanding how your users behave. One powerful tool for tracking user behavior is Google Analytics. In this blog post, we’ll explore how to integrate Google Analytics seamlessly with your NEXT.js application, and we’ll provide you with examples to get you started on the right track.
Table of Contents
1. Why Track User Behavior?
Before we dive into the technical details of integrating Google Analytics with NEXT.js, let’s briefly discuss why tracking user behavior is essential, especially for early-stage startup founders, VC investors, and tech leaders. Understanding how your users interact with your website or app can help you make informed decisions, improve user engagement, and ultimately outperform the competition.
Here are some key reasons why tracking user behavior is crucial:
1.1. Data-Driven Decisions
Having access to data about user behavior allows you to make data-driven decisions. You can identify which features are most popular, discover bottlenecks in the user journey, and prioritize improvements based on real user interactions.
1.2. Personalization
With user behavior data, you can personalize the user experience. Tailoring content and recommendations based on individual preferences can significantly enhance user satisfaction and conversion rates.
1.3. Optimize Marketing Efforts
Understanding how users find your website or app, where they drop off, and which marketing channels perform best can help you allocate resources more effectively. This optimization can lead to higher ROI on your marketing campaigns.
Now that we’ve established the importance of tracking user behavior, let’s get into the technical aspects of integrating Google Analytics with your NEXT.js project.
2. Integration Steps
2.1. Set Up a Google Analytics Account
If you don’t already have a Google Analytics account, you’ll need to create one. Go to the Google Analytics website – https://analytics.google.com/ and follow the instructions to set up your account.
2.2. Create a New Property
Once you have your account set up, create a new property for your NEXT.js application. This property will generate a unique tracking ID that you’ll use to link your app to Google Analytics.
2.3. Install Required Packages
To integrate Google Analytics with your NEXT.js project, you’ll need to install the `react-ga` package, which provides a convenient way to add Google Analytics to a React application. You can install it using npm or yarn:
```bash npm install react-ga # or yarn add react-ga ```
2.4. Initialize Google Analytics
In your NEXT.js project, you’ll want to initialize Google Analytics in a central location, such as your `_app.js` file. Import the `react-ga` package and initialize it with your tracking ID as follows:
```javascript import ReactGA from 'react-ga'; ReactGA.initialize('YOUR_TRACKING_ID'); ```
2.5. Track Page Views
Now that Google Analytics is initialized, you can start tracking page views. To do this, add the following code to your `useEffect` in your page components:
```javascript import { useEffect } from 'react'; import ReactGA from 'react-ga'; const MyPage = () => { useEffect(() => { ReactGA.pageview(window.location.pathname); }, []); return ( // Your page content ); }; export default MyPage; ```
This code will send a pageview event to Google Analytics every time a user navigates to a new page within your NEXT.js application.
2.6. Track Events
In addition to tracking page views, you can also track custom events that are relevant to your application. For example, you can track button clicks, form submissions, or video views. To track an event, use the following code:
```javascript import ReactGA from 'react-ga'; // Track a custom event ReactGA.event({ category: 'User Interaction', action: 'Button Clicked', label: 'Learn More Button', }); ```
3. Real-World Examples
Let’s take a look at some real-world examples of how early-stage startup founders, VC investors, and tech leaders can use Google Analytics in a NEXT.js application:
Example 1: A/B Testing
Suppose you’re running an A/B test to compare two different variations of your website’s homepage. By tracking user behavior using Google Analytics, you can determine which version performs better in terms of engagement, click-through rates, and conversions.
Example 2: User Onboarding
For a SaaS startup, optimizing user onboarding is crucial. By tracking user behavior during the onboarding process, you can identify where users drop off and make necessary improvements to increase user retention.
Example 3: Content Marketing
If your content marketing strategy involves blogging, you can use Google Analytics to track which blog posts are the most popular, how long users spend on each post, and which posts lead to the most conversions.
Conclusion
Integrating Google Analytics with your NEXT.js application can provide valuable insights into user behavior, enabling you to make data-driven decisions and enhance the user experience. By understanding how your users interact with your site, you can stay ahead of the competition and continuously improve your product.
Remember that tracking user behavior is an ongoing process. Regularly analyze the data, identify patterns, and adapt your strategy accordingly. With the right tools and a commitment to optimizing user experiences, you can lead your startup to success in the competitive tech industry.
In this blog post, we explored the importance of tracking user behavior, the steps to integrate Google Analytics with your NEXT.js project, and provided real-world examples of how this data can benefit early-stage startup founders, VC investors, and tech leaders. To learn more about Google Analytics and web development best practices, check out the following external resources:
- Google Analytics – https://analytics.google.com/ – Official website for Google Analytics.
- React GA Documentation – https://github.com/react-ga/react-ga – Official documentation for the `react-ga` package.
- A/B Testing Guide – https://www.optimizely.com/ab-testing/ – Learn more about A/B testing and optimization strategies.
Table of Contents
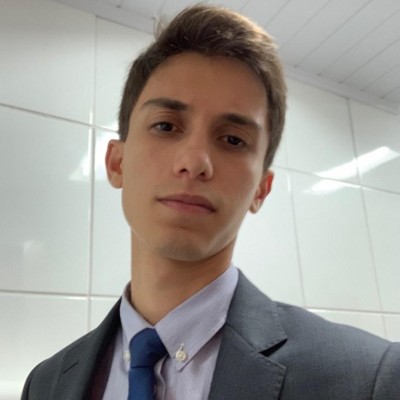
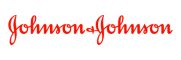