Enhance User Experience with Maps: A NEXT.js and Google Maps Integration
Location-based applications have become a fundamental part of our daily lives. Whether it’s finding the nearest coffee shop or tracking the location of your delivery, these apps rely heavily on integrating mapping services. One of the most popular choices for developers to integrate maps into their web applications is Google Maps API. In this blog post, we’ll explore how to use NEXT.js, a popular React framework, to create location-based applications using Google Maps API.
Table of Contents
1. What is NEXT.js?
NEXT.js is a React framework that helps you build server-rendered React applications with ease. It’s known for its simplicity, performance, and great developer experience. By combining NEXT.js with Google Maps API, you can create dynamic and interactive location-based features in your web applications.
2. Getting Started
To begin, make sure you have Node.js installed on your machine. If not, you can download it from Node.js website – https://nodejs.org/.
Now, let’s set up a new NEXT.js project. Open your terminal and run the following commands:
```bash npx create-next-app my-location-app cd my-location-app npm install ```
This will create a new NEXT.js project and install the necessary dependencies.
3. Integrating Google Maps API
To use Google Maps API in your application, you need an API key. You can obtain one by following the instructions on the [Google Cloud Console](https://console.cloud.google.com/). Once you have your API key, you can integrate it into your NEXT.js project.
- Create a `.env.local` file in your project’s root directory.
- Add your Google Maps API key to the `.env.local` file:
```env GOOGLE_MAPS_API_KEY=your-api-key-here ```
Now, you can access this API key in your code using `process.env.GOOGLE_MAPS_API_KEY`.
4. Creating a Location Component
Let’s create a reusable React component that will display a Google Map. Create a new file called `LocationMap.js` in your project’s `components` directory:
```javascript import React, { useEffect, useRef } from 'react'; const LocationMap = () => { const mapRef = useRef(null); useEffect(() => { const googleMapsScript = document.createElement('script'); googleMapsScript.src = `https://maps.googleapis.com/maps/api/js?key=${process.env.GOOGLE_MAPS_API_KEY}`; googleMapsScript.async = true; googleMapsScript.defer = true; googleMapsScript.addEventListener('load', initializeMap); document.body.appendChild(googleMapsScript); }, []); const initializeMap = () => { const map = new window.google.maps.Map(mapRef.current, { center: { lat: 37.7749, lng: -122.4194 }, // Default to San Francisco zoom: 12, }); // Add your location-based features here }; return ( <div ref={mapRef} style={{ height: '400px', width: '100%' }}></div> ); }; export default LocationMap; ```
In this component, we create a `div` element to hold the map and load the Google Maps API script dynamically. You can customize the map’s initial center and zoom level as needed.
5. Adding Location-based Features
Now that you have the basic map set up, you can add location-based features to your application. Here are some examples:
- Displaying User’s Location: Use the browser’s Geolocation API to get the user’s current location and display it on the map.
- Search for Places: Implement a search bar that allows users to search for nearby places, such as restaurants, parks, or landmarks, and display the results on the map.
- Directions and Routing: Utilize the Google Maps Directions API to provide directions and routing for users.
- Custom Markers: Add custom markers to the map to highlight specific locations or points of interest.
- Geofencing: Create geofencing features to trigger actions when a user enters or exits a specific geographical area.
Conclusion
In this blog post, we explored how to use NEXT.js with the Google Maps API to create location-based applications. With the power of NEXT.js and the flexibility of Google Maps, you can build interactive and dynamic maps that enhance your web applications.
If you’re looking to take your location-based app to the next level, consider exploring additional features and libraries that can complement your project. Whether you’re building a delivery tracking app, a local business directory, or a travel planning tool, integrating maps can greatly enhance the user experience.
Here are three links for further reading:
- NEXT.js Documentation – https://nextjs.org/docs – Explore the official documentation for NEXT.js to dive deeper into its features and capabilities.
- Google Maps JavaScript API Documentation – https://developers.google.com/maps/documentation/javascript/overview – Learn more about the Google Maps JavaScript API and its various functionalities.
- Geolocation API – https://developer.mozilla.org/en-US/docs/Web/API/Geolocation_API– Discover how to use the Geolocation API to access the user’s device location in web applications.
Table of Contents
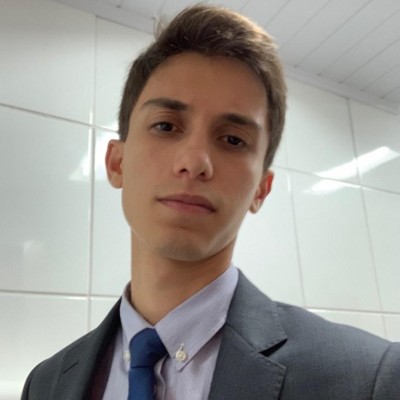
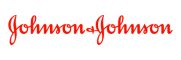