Level Up Your Web App: A Guide to GraphQL Subscriptions in NEXT.js
In the fast-paced world of web development, staying up-to-date with the latest technologies is crucial. One such technology that has gained significant traction in recent years is GraphQL. In this article, we will dive into the exciting world of GraphQL subscriptions and how to implement them in NEXT.js with Apollo Server.
Table of Contents
1. What are GraphQL Subscriptions?
GraphQL is a query language for your API that enables you to request only the data you need, making it efficient and flexible. While traditional REST APIs are request-response based, GraphQL introduces a real-time aspect with subscriptions.
GraphQL subscriptions allow clients to receive real-time updates when specific events occur on the server. This feature is particularly valuable for applications that require live data, such as chat applications, notifications, or dynamic dashboards.
2. Getting Started
To implement GraphQL subscriptions in your NEXT.js application, you’ll need to follow these steps:
- Set up your NEXT.js project.
– Create a new NEXT.js project or use an existing one.
– Install the required dependencies, including Apollo Client and Apollo Server.
- Create a GraphQL schema.
– Define your GraphQL schema, including types, queries, mutations, and subscriptions.
– Specify the events you want to subscribe to, such as “newMessage” for a chat application.
- Set up Apollo Server.
– Configure Apollo Server to handle subscriptions.
– Use the Apollo Server PubSub library to manage events and subscriptions.
- Implement GraphQL subscriptions on the client-side.
– Initialize Apollo Client with WebSocket support.
– Subscribe to the desired events using GraphQL subscriptions.
3. Examples
Let’s walk through a simple example of implementing GraphQL subscriptions in a NEXT.js application.
3.1. Create a GraphQL schema
```graphql type Message { id: ID! content: String! } type Query { messages: [Message] } type Mutation { sendMessage(content: String!): Message } type Subscription { newMessage: Message } ```
3.2. Set up Apollo Server
```javascript const { ApolloServer, PubSub } = require('apollo-server'); const typeDefs = require('./schema'); const resolvers = require('./resolvers'); const pubsub = new PubSub(); const server = new ApolloServer({ typeDefs, resolvers, context: { pubsub }, subscriptions: { onConnect: () => console.log('Client connected to subscriptions'), }, }); ```
3.3. Implement GraphQL subscriptions on the client-side
```javascript import { ApolloClient, InMemoryCache, split } from '@apollo/client'; import { WebSocketLink } from '@apollo/client/link/ws'; import { getMainDefinition } from '@apollo/client/utilities'; const httpLink = createHttpLink({ uri: '/graphql' }); const wsLink = new WebSocketLink({ uri: `ws://localhost:4000/graphql`, options: { reconnect: true, }, }); const link = split( ({ query }) => { const definition = getMainDefinition(query); return ( definition.kind === 'OperationDefinition' && definition.operation === 'subscription' ); }, wsLink, httpLink ); const client = new ApolloClient({ link, cache: new InMemoryCache(), }); ```
4. External References
To further deepen your understanding of GraphQL subscriptions, here are three external resources that provide valuable insights and examples:
- Apollo Client Documentation – https://www.apollographql.com/docs/react/)
- GraphQL Subscriptions with Apollo Server – https://www.apollographql.com/docs/apollo-server/data/subscriptions/
- Real-time GraphQL with Next.js and Apollo Client – https://dev.to/ajcwebdev/real-time-graphql-with-next-js-and-apollo-client-3547
Conclusion
Incorporating GraphQL subscriptions into your NEXT.js application can take your project to the next level by providing real-time updates to your users. By following the steps outlined in this article and referencing the external resources, you’ll be well-equipped to implement this exciting feature in your tech stack. Happy coding!
Table of Contents
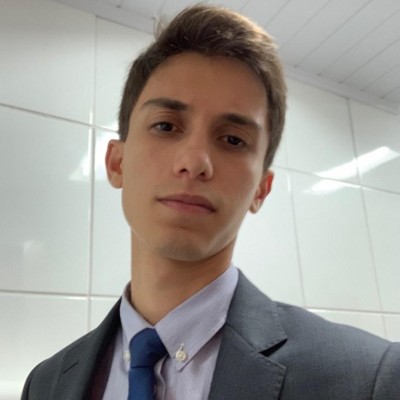
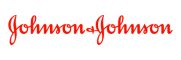