Effortless Navigation: An In-Depth Guide to Routing in Next.js
Next.js, an open-source React-based framework developed by Vercel, is renowned for its high performance and optimization features. But even the best tool in your tech stack can benefit from a little tweaking, which is why hiring proficient Next.js developers can be a game-changer. Today, we’re going to delve into some of the best practices that seasoned Next.js developers employ for optimizing your Next.js applications. We’ll discuss both general and specific optimization strategies, with plenty of examples along the way, offering valuable insights into the skill sets you should look for when hiring Next.js developers.
1. Server Side Rendering (SSR)
One of the most powerful features of Next.js is its support for Server Side Rendering (SSR). By rendering your application on the server, you can drastically improve your app’s load times and SEO performance.
```jsx export async function getServerSideProps(context) { const res = await fetch(`https://.../data`) const data = await res.json() if (!data) { return { notFound: true, } } return { props: {data}, // will be passed to the page component as props } } ```
The above snippet demonstrates how to fetch data at request time, which is an excellent example of SSR in Next.js.
2. Static Site Generation (SSG)
Although SSR is fantastic, it isn’t always the best choice. If you’re dealing with pages that don’t have dynamic data or don’t change often, you can benefit from Static Site Generation (SSG). SSG allows you to render your pages at build time, reducing the load on your server and speeding up the delivery of your pages.
```jsx export async function getStaticProps() { const res = await fetch('https://.../data') const data = await res.json() return { props: { data, }, revalidate: 1, // In seconds } } ```
The `getStaticProps` function shown above fetches data at build time. The `revalidate` parameter tells Next.js to regenerate the page at most once per second if a request comes in, perfect for low-frequency updates.
3. Incremental Static Regeneration (ISR)
A novel feature in Next.js, ISR allows you to update static content after you’ve built your page without needing a full rebuild. This approach provides the best of both worlds: the speed of static sites with the flexibility of server-side rendering.
```jsx export async function getStaticPaths() { return { paths: [ { params: { ... } } // See the "paths" section below ], fallback: 'blocking' }; } export async function getStaticProps({ params }) { // Fetch necessary data for the blog post using params.id return { props: { ... }, revalidate: 1, // In seconds } } ```
In the above code snippet, `getStaticPaths` is used in tandem with `getStaticProps` to enable ISR. The `fallback: ‘blocking‘` ensures that if a page has not been generated yet, the user will only be served the page once it’s ready, preventing a scenario where a user sees a partially rendered page.
4. Code Splitting
Next.js automatically splits your code into various bundles, which are loaded as needed rather than all at once. This technique significantly improves performance by reducing the initial load time.
Next.js handles optimization beautifully by creating separate JavaScript bundles for each page, and loading only the bundle that corresponds to the current page. However, if you have a massive component that’s only needed under certain circumstances, dynamic imports can be a great solution. This is where the expertise of professional Next.js developers shines. When you hire Next.js developers, they know how to leverage such advanced features to ensure your web application performs optimally:
```jsx import dynamic from 'next/dynamic' const HeavyComponent = dynamic(() => import('../components/HeavyComponent')) function HomePage() { return ( <div> <Header /> <HeavyComponent /> <Footer /> </div> ) } export default HomePage ```
In this example, `HeavyComponent` is loaded only when the `HomePage` component is rendered, thereby splitting the code and reducing the initial load time.
5. Optimizing Images
Next.js’s built-in Image Component and Automatic Image Optimization can dramatically improve performance by optimizing your images. The `next/image` package allows you to include images in your Next.js application seamlessly, optimizing them in various ways.
```jsx import Image from 'next/image' function MyComponent() { return ( <Image src="/me.png" // Route of the image file height={500} // Desired size with correct aspect ratio width={500} // Desired size with correct aspect ratio alt="Picture of the author" /> ) } export default MyComponent ```
In the snippet above, Next.js will automatically serve scaled images based on the device’s viewport and screen density, reducing the load time for users on smaller or lower-density screens.
6. Pre-fetching
Next.js has an in-built link component that allows you to prefetch pages automatically. This way, when a user hovers over a link, the JavaScript needed for the linked page is loaded in the background, leading to instant page transitions.
```jsx import Link from 'next/link' function Navigation() { return ( <nav> <Link href="/"> <a>Home</a> </Link> <Link href="/about"> <a>About</a> </Link> <Link href="/contact"> <a>Contact</a> </Link> </nav> ) } export default Navigation ```
In the example above, when a user hovers over the links, Next.js prefetches the necessary code in the background, ensuring the pages load instantly when clicked.
7. Using the Profiler in React Dev Tools
While this isn’t specific to Next.js, it’s a powerful tool for optimizing your React applications. With the Profiler, you can measure how often components render and what’s triggering those renders, helping you optimize your app.
You can use it by opening the React Dev Tools in your browser, switching to the “Profiler” tab, and pressing the record button. After you’ve interacted with your app a bit, press stop, and you can navigate through the render timeline, exploring what’s causing unnecessary renders.
8. Implementing Memoization
If your components render frequently and involve complex calculations or operations, memoization can help improve performance. This optimization technique involves storing the results of expensive function calls and reusing the cached result when the same inputs occur again.
In React, we use `React.memo` for memoizing components and `useMemo` for memoizing values.
Here’s an example with `React.memo`:
```jsx const MyComponent = React.memo(function MyComponent(props) { /* render using props */ }); ```
In this code snippet, `MyComponent` will only re-render if its props have changed.
Here’s an example with `useMemo`:
```jsx const memoizedValue = useMemo(() => computeExpensiveValue(a, b), [a, b]); ```
In this snippet, `computeExpensiveValue(a, b)` is only re-computed when `a` or `b` change, saving processing time on re-renders.
9. Reducing Third-Party Libraries
While third-party libraries can significantly speed up development, they can also slow down your application if not managed properly. This is where the expertise of professional Next.js developers becomes pivotal. These developers understand that some libraries might not be well optimized and can add unnecessary bloat to your bundle size, leading to longer load times.
When you hire Next.js developers, they can critically evaluate if you really need a library or if built-in Next.js features or JavaScript/React APIs can achieve the same result, an essential skill that contributes to efficient application performance. If the use of a third-party library is unavoidable, seasoned Next.js developers make sure to check if there’s a lighter version available or if they can import only the parts you need, optimizing your application’s performance and efficiency.
10. Using the Latest Versions
The Next.js team is constantly working to improve performance. By staying up to date with the latest releases, you can benefit from these enhancements.
To update Next.js, you can run:
```bash npm i next@latest ```
Keep in mind that you should also ensure compatibility with other dependencies and review the change logs for any breaking changes.
Conclusion
Next.js is a powerful tool for building optimized web applications, but there’s always room for improvement. When you hire Next.js developers, they can leverage features like SSR, SSG, ISR, code-splitting, image optimization, and prefetching, using tools like the React Dev Tools profiler to drastically improve the performance of your Next.js applications. Experienced Next.js developers understand that optimization is a continuous process, not a one-time task. With their expertise, they regularly profile and check your app for potential bottlenecks and areas to improve, ensuring your application runs at its peak performance at all times.
Table of Contents
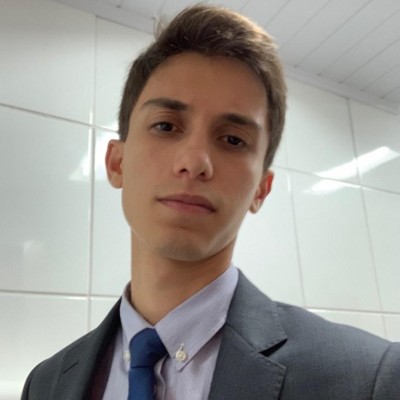
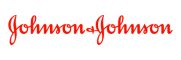