Implementing Server-side Analytics in NEXT.js with Google Analytics
In the world of web development, analytics play a crucial role in understanding user behavior, optimizing performance, and making informed decisions. Google Analytics is one of the most popular tools used for tracking website activity and gathering valuable insights. While client-side analytics can provide a wealth of information, server-side analytics offer enhanced accuracy and improved user privacy. In this tutorial, we’ll explore how to implement server-side analytics in a NEXT.js application using Google Analytics.
1. Why Server-side Analytics?
Before diving into the implementation, let’s briefly discuss why server-side analytics are worth considering. Traditional client-side analytics involve adding a tracking script to your web pages. This script collects data as users interact with your site and sends it to the analytics provider’s servers. However, this approach has some limitations:
- JavaScript Dependencies: Client-side analytics depend on JavaScript. If a user disables JavaScript in their browser, the tracking won’t work.
- Ad Blockers: Some users employ ad blockers or tracking protection extensions that can block client-side tracking scripts.
- Data Accuracy: Client-side analytics might provide inaccurate data if users leave a page before the tracking script executes fully.
- User Privacy: With increasing concerns about user privacy, some visitors might be hesitant to allow client-side scripts to track their activity.
- Server-side analytics address these issues. By collecting data on the server before rendering content, you can ensure accurate tracking regardless of user behavior. Additionally, server-side tracking is less likely to be blocked by ad blockers, as it doesn’t rely on client-side JavaScript.
2. Setting Up Google Analytics
Before we proceed, ensure you have a Google Analytics account and have created a property for your NEXT.js application.
- Get Tracking ID: Once you’ve set up your property, you’ll receive a tracking ID. This ID is a unique identifier for your property and is required to send data to Google Analytics.
- Enable Server-Side Tracking: Google Analytics now supports server-side tracking. This is achieved by sending analytics data directly from your server to Google Analytics, rather than relying solely on client-side scripts.
3. Integrating Google Analytics with NEXT.js
Let’s dive into the implementation process of server-side analytics in a NEXT.js application.
3.1. Installing Dependencies
To get started, make sure you have Node.js and npm (Node Package Manager) installed. If you haven’t already, create a new NEXT.js project using the following commands:
bash npx create-next-app my-analytics-app cd my-analytics-app
Now, install the necessary packages for integrating Google Analytics and server-side tracking:
bash npm install react-ga universal-cookie
The react-ga package provides a wrapper for the Google Analytics tracking code, while universal-cookie allows you to work with cookies across the server and client.
3.2. Setting Up the Analytics Wrapper
Create a new file named analytics.js in your project’s root directory. This file will contain the setup for your Google Analytics tracking. Add the following code to the file:
javascript import ReactGA from 'react-ga'; export const initGA = () => { ReactGA.initialize('YOUR_TRACKING_ID'); }; export const logPageView = () => { ReactGA.set({ page: window.location.pathname }); ReactGA.pageview(window.location.pathname); };
Replace ‘YOUR_TRACKING_ID’ with the actual tracking ID you obtained from your Google Analytics property.
3.3. Implementing Server-Side Tracking
In NEXT.js, you can use the getServerSideProps function to fetch data on the server side before rendering the page. We’ll use this function to implement server-side tracking. Open the _app.js file in your pages directory and modify it as follows:
javascript import { useEffect } from 'react'; import { initGA, logPageView } from '../analytics'; import { useRouter } from 'next/router'; function MyApp({ Component, pageProps }) { const router = useRouter(); useEffect(() => { initGA(); logPageView(); router.events.on('routeChangeComplete', logPageView); return () => { router.events.off('routeChangeComplete', logPageView); }; }, []); return <Component {...pageProps} />; } export default MyApp;
In this code, we import the initGA and logPageView functions from the analytics.js file. In the useEffect hook, we initialize Google Analytics and log the page view when the component mounts. We also set up a listener for route changes so that the page view is tracked whenever the user navigates to a new page.
3.4. Testing the Implementation
With the setup in place, you can now test your server-side analytics implementation. Start your NEXT.js development server using the command:
bash npm run dev
Visit your application in a web browser, and as you navigate through different pages, you should see the data being tracked in your Google Analytics property.
4. Benefits of Server-side Analytics in NEXT.js
Implementing server-side analytics in your NEXT.js application offers several benefits:
- Accurate Data: Server-side tracking ensures that data is collected accurately, regardless of user behavior or JavaScript settings.
- Ad Blocker Resilience: Server-side analytics are less likely to be affected by ad blockers or tracking protection extensions.
- Improved User Privacy: Some users have concerns about client-side tracking scripts. Server-side tracking is less invasive and respects user privacy.
- SEO Optimization: Server-side rendering enhances SEO by providing search engines with fully rendered HTML pages.
Conclusion
In this tutorial, we explored the advantages of implementing server-side analytics in a NEXT.js application using Google Analytics. We discussed the limitations of traditional client-side tracking and how server-side tracking addresses these issues. By setting up server-side tracking, you can ensure accurate data collection, enhanced user privacy, and improved SEO optimization for your application. With the steps outlined in this guide, you’re well on your way to gathering meaningful insights and making informed decisions to enhance your web application’s performance and user experience.
Table of Contents
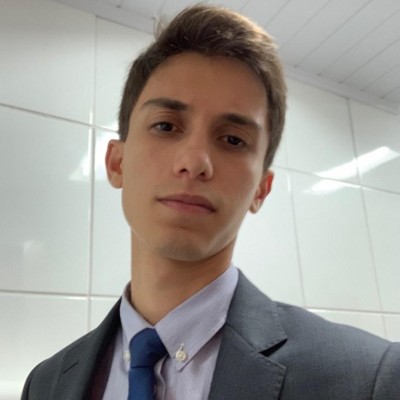
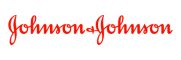