Authentication with NEXT.js: JWT and Token-based Authentication
In today’s digital landscape, where user privacy and security are paramount, implementing robust authentication mechanisms in web applications is crucial. NEXT.js, a popular React framework for building web applications, offers a seamless way to integrate authentication using JSON Web Tokens (JWT) and token-based authentication. In this blog post, we will explore the concepts of JWT, token-based authentication, and how to implement them in a NEXT.js application to ensure secure user interactions.
1. Understanding Authentication and JWT
1.1. What is Authentication?
Authentication is the process of verifying the identity of a user, ensuring they are who they claim to be. It prevents unauthorized access to sensitive resources by requiring users to provide valid credentials, such as usernames and passwords.
1.2. Introducing JSON Web Tokens (JWT)
JWT is a compact and self-contained way to represent information between two parties securely. It consists of three parts: header, payload, and signature. The header specifies the algorithm used for encryption, the payload contains the claims, and the signature ensures the integrity of the token. JWTs are often used for stateless authentication.
2. Advantages of Token-based Authentication
2.1. Stateless Nature
Token-based authentication is stateless, meaning the server doesn’t need to store session information. Each request contains the necessary authentication token, making the system more scalable and reducing the load on the server.
2.2. Enhanced Security
JWTs are signed and optionally encrypted, ensuring that the token’s content remains tamper-proof during transmission. This enhances security by preventing unauthorized modifications.
2.3. Scalability
Since the server doesn’t need to maintain session state, token-based authentication is more scalable. This is especially beneficial for applications with a large user base.
3. Setting Up a NEXT.js Application
3.1. Creating a New NEXT.js Project
To get started, let’s create a new NEXT.js project by running the following commands:
bash npx create-next-app my-auth-app cd my-auth-app
3.2. Installing Dependencies
For authentication, we’ll need some dependencies. Install them using:
bash npm install jsonwebtoken axios
4. Implementing JWT-based Authentication
4.1. Generating and Signing JWTs
In the server code (e.g., an API route), you can generate and sign a JWT using a secret key:
javascript const jwt = require('jsonwebtoken'); const user = { id: 123, username: 'example_user' }; const secretKey = 'your-secret-key'; const token = jwt.sign(user, secretKey, { expiresIn: '1h' });
4.2. Storing JWTs on the Client
Once the server sends the token to the client, it can be stored in the browser’s localStorage or cookies:
javascript // Storing in localStorage localStorage.setItem('token', token); // Storing in cookies (using a library like js-cookie) import Cookies from 'js-cookie'; Cookies.set('token', token);
4.3. Verifying JWTs on the Server
To authenticate incoming requests, you can verify the JWT on the server:
javascript const token = req.headers.authorization.split(' ')[1]; jwt.verify(token, secretKey, (err, decoded) => { if (err) { // Token is invalid } else { // Token is valid, decoded contains user info } });
5. Building Protected Routes
5.1. Creating a Protected Route Component
To create protected routes, you can build a Higher Order Component (HOC) that checks for authentication before rendering the component:
javascript import { useEffect } from 'react'; import { useRouter } from 'next/router'; import jwt from 'jsonwebtoken'; const secretKey = 'your-secret-key'; const withAuth = (WrappedComponent) => { return (props) => { const router = useRouter(); useEffect(() => { const token = localStorage.getItem('token'); if (!token) { router.replace('/login'); // Redirect to login if not authenticated } else { jwt.verify(token, secretKey, (err) => { if (err) { router.replace('/login'); // Redirect to login if token is invalid } }); } }, []); return <WrappedComponent {...props} />; }; }; export default withAuth;
5.3. Redirection for Unauthenticated Users
Use the withAuth HOC to protect routes in your application:
javascript import withAuth from '../path/to/withAuth'; const ProtectedPage = () => { return <div>This is a protected page.</div>; }; export default withAuth(ProtectedPage);
6. Token Expiration and Refresh
6.1. Setting JWT Expiration
JWTs can have an expiration time to enhance security. This can be set during token generation:
javascript const token = jwt.sign(user, secretKey, { expiresIn: '1h' });
6.2. Implementing Token Refresh
When a token expires, users should refresh it without the need to re-authenticate. This can be done by issuing a refresh token alongside the access token.
7. Handling Logouts
7.1. Clearing Tokens
To log a user out, clear the stored token:
javascript localStorage.removeItem('token');
7.2. Redirecting on Logout
After clearing the token, redirect the user to the login page:
javascript import { useEffect } from 'react'; import { useRouter } from 'next/router'; const LogoutPage = () => { const router = useRouter(); useEffect(() => { localStorage.removeItem('token'); router.replace('/login'); }, []); return <div>Logging out...</div>; }; export default LogoutPage;
8. Best Practices for JWT and Token-based Authentication
8.1. Use HTTPS
Always use HTTPS to ensure secure communication between the client and the server, preventing eavesdropping and data tampering.
8.2. Keep Tokens Secure
Store tokens in a secure manner, such as in HttpOnly cookies or encrypted local storage. This prevents cross-site scripting (XSS) attacks.
8.3. Implement Token Revocation
If needed, implement a token revocation mechanism for enhanced security. This allows you to invalidate tokens before they expire.
Conclusion
In this blog post, we explored the concepts of JSON Web Tokens (JWT) and token-based authentication, and how to implement them in a NEXT.js application. By integrating JWT-based authentication, you can ensure secure, stateless, and scalable user authentication, providing a seamless experience for your users while maintaining their privacy and data security. Remember to follow best practices and stay updated on security trends to keep your application’s authentication mechanisms robust and effective. Happy coding!
Table of Contents
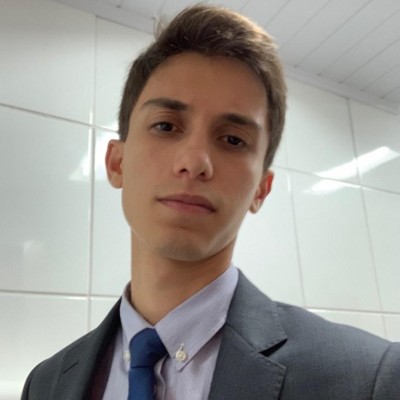
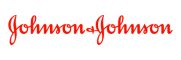