Using NEXT.js with MongoDB Atlas: Cloud Database Integration
In today’s fast-paced world of web development, building scalable and data-driven web applications is a common requirement. MongoDB Atlas, a cloud-based database service, and NEXT.js, a React framework for building server-rendered React applications, can be an excellent combination for achieving this goal. In this blog post, we’ll explore how to integrate MongoDB Atlas with NEXT.js to create powerful and efficient web applications.
1. Why MongoDB Atlas?
MongoDB Atlas is a fully managed cloud database service provided by MongoDB, Inc. It offers several advantages that make it a preferred choice for developers:
1.1. Scalability and Flexibility
MongoDB Atlas allows you to easily scale your database as your application grows. You can start with a small cluster and scale it up to meet the demands of your application without any downtime.
1.2. High Availability
MongoDB Atlas provides built-in replication and automatic failover to ensure your database is always available. This helps in creating robust and reliable applications.
1.3. Security
Security is a top priority for MongoDB Atlas. It offers features like network isolation, encryption at rest, and authentication to keep your data safe and compliant with industry regulations.
1.4. Global Reach
With MongoDB Atlas, you can deploy your database clusters in multiple regions worldwide. This ensures low-latency access to your data for users across the globe.
2. Getting Started with NEXT.js
Before we dive into integrating MongoDB Atlas, let’s briefly introduce NEXT.js for those who may not be familiar with it. NEXT.js is a React framework that provides server-side rendering (SSR) out of the box, making it a powerful choice for building SEO-friendly and high-performance web applications.
To get started with NEXT.js, you can follow these steps:
Step 1: Create a New NEXT.js App
You can create a new NEXT.js app using the following command:
bash npx create-next-app my-next-app
This command sets up a new NEXT.js project with all the necessary files and dependencies.
Step 2: Start the Development Server
Once your project is created, navigate to the project directory and start the development server:
bash cd my-next-app npm run dev
This will start the development server, and you can access your app at http://localhost:3000.
Step 3: Create Pages
NEXT.js follows a “pages” convention, where each JavaScript file in the “pages” directory becomes a route in your application. For example, creating a file named about.js in the “pages” directory would make the “About” page accessible at /about.
Step 4: Build Your Components
You can build your application components using React, and NEXT.js will take care of server-side rendering and routing for you.
Now that we have a basic understanding of NEXT.js, let’s proceed to integrate it with MongoDB Atlas.
3. Integrating MongoDB Atlas with NEXT.js
To use MongoDB Atlas with NEXT.js, you’ll need to perform the following steps:
Step 1: Create a MongoDB Atlas Account
If you don’t already have a MongoDB Atlas account, sign up for one at https://www.mongodb.com/cloud/atlas. MongoDB Atlas offers a free tier, which is suitable for development and testing.
Step 2: Create a Cluster
Once you have an account, create a new cluster in MongoDB Atlas. A cluster is a group of servers that host your database. You can choose the cloud provider and region for your cluster based on your application’s requirements.
Step 3: Whitelist IP Addresses
To connect to your MongoDB Atlas cluster, you’ll need to whitelist the IP addresses that can access it. You can do this in the “Network Access” section of your cluster configuration. Be sure to add the IP address of your development machine if you’re testing locally.
Step 4: Create a Database User
In the “Database Access” section of your cluster configuration, create a database user with appropriate permissions for your application. You’ll need the username and password of this user to connect to the database.
Step 5: Get Connection String
Now, you can obtain the connection string for your MongoDB Atlas cluster. This string contains all the information needed to connect to your database, including the username, password, cluster address, and database name.
Step 6: Install MongoDB Driver
To interact with MongoDB from your NEXT.js application, you’ll need to install the MongoDB driver for Node.js. You can do this using npm:
bash npm install mongodb
Step 7: Set Up Environment Variables
It’s essential to keep sensitive information like your database connection string secure. You can use environment variables to store this information. Create a .env.local file in your NEXT.js project and add the following:
dotenv MONGODB_URI=<your-mongodb-uri>
Replace <your-mongodb-uri> with the actual connection string you obtained in step 5.
Step 8: Create a MongoDB Client
Now, let’s create a MongoDB client in your NEXT.js application. You can do this in a separate file, such as db.js:
javascript import { MongoClient } from 'mongodb'; let client; if (!client) { client = new MongoClient(process.env.MONGODB_URI, { useNewUrlParser: true, useUnifiedTopology: true, }); if (!client.isConnected()) { client.connect(); } } export default client;
This code sets up a MongoDB client using the connection string from your environment variables.
Step 9: Use MongoDB in Your NEXT.js Pages
With the MongoDB client in place, you can now use it in your NEXT.js pages. Here’s an example of how to fetch data from MongoDB and display it on a page:
javascript import client from '../path-to-db'; // Replace with the actual path to your db.js file export async function getStaticProps() { const db = client.db(); const collection = db.collection('your-collection'); // Replace with your collection name const data = await collection.find().toArray(); return { props: { data, }, }; } function MyPage({ data }) { return ( <div> {data.map((item) => ( <div key={item._id}>{item.title}</div> ))} </div> ); } export default MyPage;
In this example, we use the getStaticProps function to fetch data from MongoDB and pass it as props to the page component.
4. Deployment Considerations
When deploying your NEXT.js application with MongoDB Atlas, you should consider the following:
4.1. Environment Variables
Make sure to set the environment variables containing your MongoDB Atlas connection string in your production environment. Most hosting platforms provide a way to configure environment variables securely.
4.2. Connection Pooling
MongoDB Atlas provides connection pooling by default, which helps manage database connections efficiently. However, be mindful of connection limits imposed by your MongoDB Atlas plan.
4.3. Error Handling
Handle database connection errors gracefully in your NEXT.js application to provide a good user experience. Implement proper error handling and logging to troubleshoot issues.
Conclusion
Integrating MongoDB Atlas with NEXT.js opens up a world of possibilities for building dynamic and data-driven web applications. With the scalability, reliability, and security offered by MongoDB Atlas and the server-side rendering capabilities of NEXT.js, you can create high-performance web applications that meet the demands of modern users.
By following the steps outlined in this blog post, you can seamlessly connect your NEXT.js application to MongoDB Atlas and leverage the power of a cloud-based database to store and retrieve data. This combination allows you to focus on building great user experiences while MongoDB Atlas takes care of data management and scalability.
So, whether you’re building a blog, an e-commerce site, or any other web application, consider using MongoDB Atlas with NEXT.js for a robust and efficient solution. Happy coding!
Do you have any questions or need further assistance with MongoDB Atlas and NEXT.js integration? Feel free to ask in the comments below!
Table of Contents
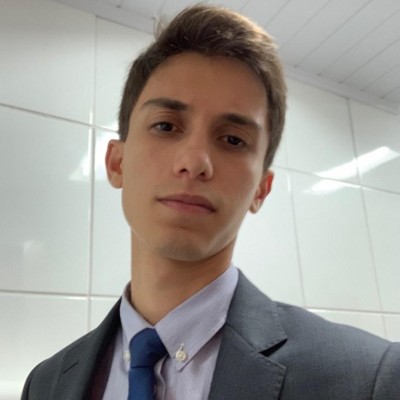
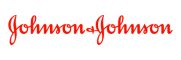