Deploying NEXT.js Apps to Kubernetes: Containerization and Orchestration
In today’s fast-paced development landscape, deploying web applications efficiently and reliably has become a crucial aspect of software engineering. Traditional methods of deploying applications often lack scalability, ease of management, and quick scaling capabilities. This is where Kubernetes comes into play. Kubernetes, an open-source container orchestration platform, provides a powerful solution for deploying, managing, and scaling containerized applications. In this tutorial, we’ll delve into the process of deploying NEXT.js apps to Kubernetes, taking advantage of containerization and orchestration for a streamlined and scalable deployment workflow.
1. Prerequisites
Before we dive into the deployment process, let’s ensure you have the necessary tools and knowledge in place:
- Kubernetes Cluster: Set up a Kubernetes cluster using a platform like Minikube or a managed Kubernetes service like Google Kubernetes Engine (GKE).
- Docker: Familiarize yourself with Docker, as we’ll be containerizing our NEXT.js app using Docker images.
- NEXT.js App: Have a NEXT.js application ready to deploy. If you don’t have one, you can quickly create a simple app using the NEXT.js CLI.
2. Containerization with Docker
Containerization encapsulates an application along with its dependencies and runtime environment into a lightweight, portable container. Docker is a popular tool for creating and managing containers. Let’s containerize our NEXT.js app using Docker.
2.1. Create a Dockerfile
In your NEXT.js app’s root directory, create a file named Dockerfile (without any file extension) and add the following content:
Dockerfile # Use an official Node.js runtime as the base image FROM node:14 # Set the working directory within the container WORKDIR /usr/src/app # Copy package.json and package-lock.json to the container COPY package*.json ./ # Install app dependencies RUN npm install # Copy the rest of the application code COPY . . # Build the app RUN npm run build # Expose the port the app runs on EXPOSE 3000 # Define the command to run the app CMD [ "npm", "start" ]
2.2. Build the Docker Image
In your terminal, navigate to the directory containing the Dockerfile and execute the following command to build the Docker image:
bash docker build -t nextjs-app .
This command builds an image named nextjs-app using the instructions specified in the Dockerfile.
2.3. Test the Docker Image
To test the Docker image locally, run the following command:
bash docker run -p 3000:3000 nextjs-app
Visit http://localhost:3000 in your browser to verify that your NEXT.js app is running within a Docker container.
3. Deploying to Kubernetes
With our NEXT.js app successfully containerized using Docker, it’s time to deploy it to our Kubernetes cluster.
3.1. Kubernetes Deployment Configuration
Create a Kubernetes deployment configuration file, named nextjs-deployment.yaml, to define how our app should be deployed within the cluster:
yaml apiVersion: apps/v1 kind: Deployment metadata: name: nextjs-app-deployment spec: replicas: 3 # Number of replicas (pods) to maintain selector: matchLabels: app: nextjs-app template: metadata: labels: app: nextjs-app spec: containers: - name: nextjs-app image: nextjs-app # Use the Docker image we built earlier ports: - containerPort: 3000
3.2. Apply the Deployment
In your terminal, apply the deployment configuration to your Kubernetes cluster:
bash kubectl apply -f nextjs-deployment.yaml
This command instructs Kubernetes to create and manage the deployment based on the configuration in the YAML file.
3.3. Exposing the Service
To make our app accessible from outside the cluster, we need to expose it using a Kubernetes Service. Create a file named nextjs-service.yaml with the following content:
yaml apiVersion: v1 kind: Service metadata: name: nextjs-app-service spec: selector: app: nextjs-app ports: - protocol: TCP port: 80 targetPort: 3000 type: LoadBalancer
Apply the service configuration:
bash kubectl apply -f nextjs-service.yaml
3.4. Accessing the App
After applying the service configuration, your NEXT.js app will be accessible through the LoadBalancer’s external IP address. You can find this IP by running:
bash kubectl get services nextjs-app-service
Visit the provided IP address in your browser to see your deployed NEXT.js app.
4. Scaling and Updating
One of Kubernetes’ strengths is its ability to scale and update applications seamlessly.
4.1. Scaling the App
To scale your app, you can simply adjust the replicas field in the deployment configuration (nextjs-deployment.yaml). For instance, to scale up to 5 replicas:
yaml spec: replicas: 5
Apply the updated configuration with:
bash kubectl apply -f nextjs-deployment.yaml
4.2. Updating the App
When you need to update your app, make changes to your code and build a new Docker image. Then, update the image tag in the deployment configuration (nextjs-deployment.yaml):
yaml spec: template: spec: containers: - name: nextjs-app image: nextjs-app:newtag # Update the tag
Apply the updated configuration to apply the changes:
bash kubectl apply -f nextjs-deployment.yaml
Kubernetes will perform a rolling update, ensuring zero downtime during the process.
Conclusion
Deploying NEXT.js apps to Kubernetes empowers developers to efficiently manage, scale, and update their applications. By containerizing the app with Docker and leveraging Kubernetes’ orchestration capabilities, you can achieve a highly scalable and manageable deployment workflow. Whether you’re launching a new app or migrating an existing one, embracing Kubernetes streamlines the deployment process and sets the stage for future growth and innovation. So, get ready to elevate your deployment game with Kubernetes and take your NEXT.js apps to the next level of performance and scalability.
Table of Contents
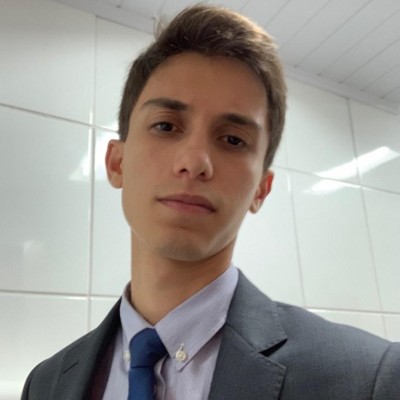
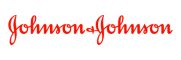