Optimizing NEXT.js Apps for Mobile Devices: Responsive Design and Touch Gestures
In today’s digital landscape, optimizing web applications for mobile devices is paramount. With the increasing number of users accessing websites on smartphones and tablets, it’s crucial to ensure that your NEXT.js apps are not only responsive but also offer a seamless touch experience. In this blog post, we’ll delve into the world of optimizing NEXT.js apps for mobile devices by focusing on responsive design and touch gesture integration. We’ll cover best practices, useful techniques, and provide code samples to help you create high-performing and user-friendly applications.
1. Why Mobile Optimization Matters
Mobile optimization is no longer a luxury; it’s a necessity. With mobile devices accounting for a significant portion of web traffic, failing to optimize your NEXT.js app for these devices can lead to poor user experiences, high bounce rates, and lost conversions. Responsive design and touch gesture integration play a pivotal role in delivering a consistent and enjoyable user experience across various screen sizes and devices.
2. Understanding Responsive Design
2.1. The Flexible Grid and Layouts
Responsive design revolves around creating layouts that adapt to different screen sizes without compromising on functionality or aesthetics. The cornerstone of responsive design is the flexible grid system. Instead of fixed pixel-based layouts, use relative units like percentages or “em” to create fluid and dynamic grids. In NEXT.js apps, you can achieve this by utilizing CSS frameworks like Flexbox or CSS Grid.
Code Sample 1: Using Flexbox for Responsive Layout
jsx import styles from './styles.module.css'; const ResponsiveLayout = () => { return ( <div className={styles.container}> <div className={styles.item}>Item 1</div> <div className={styles.item}>Item 2</div> <div className={styles.item}>Item 3</div> </div> ); }; export default ResponsiveLayout;
styles.module.css
css .container { display: flex; flex-wrap: wrap; justify-content: space-between; } .item { width: calc(33.33% - 1rem); margin: 0.5rem; background-color: lightgray; text-align: center; }
2.2. Media Queries for Breakpoints
Media queries allow you to apply different styles based on the device’s screen width. By defining breakpoints and adjusting your layout accordingly, you can ensure that your NEXT.js app looks great on both large desktop screens and small mobile displays.
Code Sample 2: Implementing Media Queries
css /* styles.module.css */ /* For screens smaller than 600px */ @media (max-width: 600px) { .item { width: calc(50% - 1rem); } } /* For screens smaller than 400px */ @media (max-width: 400px) { .item { width: 100%; } }
3. Integrating Touch Gestures
Mobile devices introduce a tactile dimension to user interactions through touch gestures. Integrating touch gestures enhances user engagement and provides a more intuitive experience. Let’s explore how to implement touch gestures in your NEXT.js app.
3.1. Tap and Double-Tap
Tapping is the fundamental touch gesture, akin to clicking with a mouse. You can capture tap events on elements using the onClick event handler in React components.
Code Sample 3: Implementing Tap Gesture
jsx import React from 'react'; const TapGesture = () => { const handleTap = () => { alert('Element tapped!'); }; return ( <div onClick={handleTap} style={{ padding: '1rem', background: 'lightblue' }}> Tap Me! </div> ); }; export default TapGesture;
3.2. Swiping and Scroll
Swiping and scrolling are common touch gestures used for navigating content. You can capture swiping or scrolling events by monitoring touch movements using the onTouchStart, onTouchMove, and onTouchEnd event handlers.
Code Sample 4: Implementing Swipe Gesture
jsx import React, { useState } from 'react'; const SwipeGesture = () => { const [position, setPosition] = useState(0); const [startX, setStartX] = useState(0); const handleTouchStart = (e) => { setStartX(e.touches[0].clientX); }; const handleTouchMove = (e) => { const deltaX = e.touches[0].clientX - startX; setPosition(deltaX); }; const handleTouchEnd = () => { // Perform action based on swipe direction and distance if (position > 100) { // Swipe right action } else if (position < -100) { // Swipe left action } setPosition(0); }; return ( <div onTouchStart={handleTouchStart} onTouchMove={handleTouchMove} onTouchEnd={handleTouchEnd} style={{ width: '100%', height: '200px', background: 'lightgray', transform: `translateX(${position}px)`, transition: 'transform 0.3s ease', }} > Swipe me! </div> ); }; export default SwipeGesture;
4. Performance Considerations
While responsive design and touch gesture integration are essential, optimizing performance for mobile devices is equally crucial. Here are some performance considerations to keep in mind:
4.1. Lazy Loading
Lazy loading involves loading assets, such as images or videos, only when they come into the user’s viewport. This reduces initial page load times and conserves data usage, making your NEXT.js app feel faster and more responsive.
4.2. Minification and Compression
Minify your JavaScript, CSS, and HTML files to remove unnecessary whitespace and reduce file sizes. Additionally, enable server-side compression to deliver compressed assets to the client, further reducing load times.
4.3. Caching and CDNs
Implement browser caching and leverage content delivery networks (CDNs) to store and serve assets from servers located closer to the user. This reduces the distance data needs to travel, improving load times.
Conclusion
Optimizing NEXT.js apps for mobile devices is a multifaceted endeavor. By embracing responsive design principles and integrating touch gestures, you can create applications that provide a seamless and enjoyable user experience across various devices. Remember to prioritize performance optimization techniques to ensure that your app not only looks great but also loads quickly. With these strategies and code samples in your toolkit, you’re well-equipped to craft high-performing and user-friendly NEXT.js apps that cater to the ever-growing mobile audience.
Table of Contents
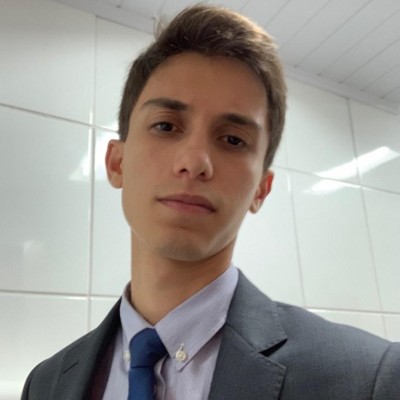
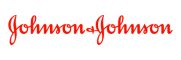