Optimizing Images in NEXT.js: Strategies for Faster Loading
In today’s fast-paced digital world, website loading speed plays a crucial role in user experience and search engine rankings. As web developers, it’s essential to optimize our applications to deliver a smooth and efficient experience for users. One significant factor that affects website performance is image optimization.
Images are an integral part of most websites, and they often contribute to the bulk of the page size. In a NEXT.js application, you have various options and techniques to optimize images and enhance the overall loading speed. In this blog, we will explore some effective strategies, best practices, and code samples to ensure that your NEXT.js website loads lightning fast without compromising on visual quality.
Why Image Optimization Matters?
Before diving into the strategies, let’s understand why image optimization is crucial for your NEXT.js application.
- Page Load Speed: Images can significantly impact page load speed, affecting user engagement and retention. Faster-loading pages lead to improved user satisfaction and retention rates.
- Mobile Experience: With a growing number of users accessing websites on mobile devices, image optimization becomes even more critical. Optimized images ensure a smooth experience across various devices and screen sizes.
- SEO (Search Engine Optimization): Search engines consider page speed as a ranking factor. By optimizing images, you can positively influence your website’s search engine rankings.
- Bandwidth and Server Costs: Unoptimized images consume more bandwidth and server resources. By optimizing images, you can save on hosting costs and deliver a better experience to users with limited data plans.
Strategies for Image Optimization
Now that we understand the significance of image optimization, let’s explore various strategies and techniques to optimize images in your NEXT.js application.
1. Choose the Right Image Format
Selecting the appropriate image format is the first step towards optimization. Different image formats have different qualities, compression levels, and use cases. The commonly used image formats are:
- JPEG (Joint Photographic Experts Group): Ideal for photographs and images with many colors and gradients. JPEG images can be compressed to reduce file size, but excessive compression may lead to a loss in visual quality.
- PNG (Portable Network Graphics): Suitable for images with transparency and sharp edges, like logos and icons. PNGs support lossless compression, making them higher in file size than JPEGs.
- WebP: A modern image format developed by Google, WebP provides both lossless and lossy compression. It usually results in smaller file sizes compared to JPEG and PNG, without compromising quality.
Choosing the right format can significantly reduce image sizes and improve loading speed.
2. Image Resizing
Using images in their original size, especially for large images, can lead to slower loading times. To ensure optimal performance, resize images to the exact dimensions required for display.
In NEXT.js, you can use the next/image component to handle image resizing automatically. Let’s take a look at an example:
jsx import Image from 'next/image'; const MyComponent = () => { return ( <div> <Image src="/images/my-image.jpg" alt="My Image" width={500} height={300} /> </div> ); };
In the above code, the image with the path /images/my-image.jpg will be resized to 500×300 pixels, ensuring it fits the container without any extra overhead.
3. Use Image Compression
Compression reduces the file size of images without compromising on quality. There are two types of image compression:
- Lossless Compression: Reduces the file size without losing any image data. This is ideal for images that require pixel-perfect accuracy, like icons and logos. Use the next/image component’s quality attribute for lossless compression:
jsx <Image src="/images/my-logo.png" alt="Logo" width={200} height={100} quality={100} />
- Lossy Compression: This type of compression reduces the file size by discarding some image data, leading to a slight loss in quality. It is suitable for photographs and images with many colors. The next/image component’s quality attribute can be used for lossy compression as well:
jsx <Image src="/images/my-photo.jpg" alt="Photo" width={800} height={600} quality={70} />
Remember to strike a balance between image quality and file size to achieve the best results.
4. Lazy Loading
Lazy loading is a technique that defers the loading of non-critical resources, such as images, until they are about to be displayed on the user’s screen. This reduces the initial page load time and saves bandwidth for users who don’t scroll down the entire page.
In NEXT.js, lazy loading can be easily achieved using the loading attribute in the next/image component:
jsx <Image src="/images/my-image.jpg" alt="My Image" width={500} height={300} loading="lazy" />
By enabling lazy loading, images will only be loaded when they are near the user’s viewport, improving the overall page loading performance.
5. Use Image CDNs
Content Delivery Networks (CDNs) distribute your images across multiple servers worldwide. This reduces the distance between the user and the server, resulting in faster image loading times. Additionally, CDNs can automatically optimize and serve images in the most efficient format for each user’s device.
Many popular CDNs offer integration with NEXT.js, making it easy to incorporate them into your application. One such CDN is Cloudinary. Here’s how you can use Cloudinary in your NEXT.js project:
jsx import Image from 'next/image'; const MyComponent = () => { return ( <div> <Image src="https://res.cloudinary.com/your-account/image/upload/v1234567/my-image.jpg" alt="My Image" width={500} height={300} /> </div> ); };
Using CDNs like Cloudinary can significantly speed up image delivery and optimize images for different devices and resolutions.
6. Implement Image Loading Placeholder
While images are being fetched, there may be a slight delay before they appear on the screen. Implementing a loading placeholder can enhance the user experience by providing visual feedback while the image loads. In NEXT.js, you can achieve this using the placeholder attribute:
jsx <Image src="/images/my-image.jpg" alt="My Image" width={500} height={300} placeholder="blur" />
The placeholder=”blur” attribute will display a blurred version of the image while it’s loading. This gives users an indication that content is loading and improves perceived performance.
7. WebP Format for Modern Browsers
As mentioned earlier, WebP is a modern image format that offers excellent compression without compromising on quality. Modern browsers that support WebP will automatically request the WebP version of an image if available. By providing WebP images for supported browsers, you can further improve loading speed and user experience.
NEXT.js makes it easy to serve WebP images using the next/image component. Here’s an example of how you can implement it:
jsx <Image src="/images/my-image.webp" alt="My Image" width={500} height={300} />
By using WebP, you ensure that users with modern browsers get the best possible image loading performance.
Conclusion
Optimizing images is a vital step in ensuring a fast and responsive NEXT.js application. By choosing the right image format, resizing and compressing images, lazy loading, using CDNs, and providing WebP images for modern browsers, you can significantly improve the loading speed and user experience of your website.
Remember to strike a balance between image quality and file size, as excessively compressed images may lead to a loss in visual appeal. Regularly analyze your website’s performance and make adjustments as needed to deliver an optimal experience to your users.
By following these image optimization strategies and best practices, you’ll not only improve your website’s loading speed but also create a positive impression on your users, leading to higher engagement and better business outcomes.
So, go ahead and implement these image optimization techniques in your NEXT.js projects to provide your users with a blazing-fast and delightful web experience!
Table of Contents
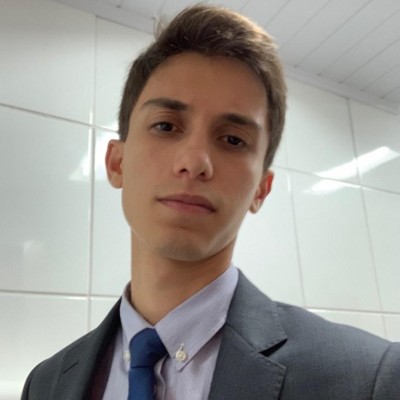
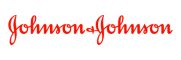