Optimizing SEO in NEXT.js: Meta Tags, Sitemaps, and Open Graph
Search Engine Optimization (SEO) is crucial for any website looking to improve its online visibility and attract organic traffic. When it comes to building high-performance and SEO-friendly web applications, NEXT.js, a popular React framework, offers a powerful platform. In this blog, we will explore essential SEO practices within NEXT.js, focusing on optimizing meta tags, sitemaps, and Open Graph.
1. Understanding SEO in NEXT.js
NEXT.js is a powerful framework for building server-rendered React applications, providing great performance out of the box. However, to ensure optimal SEO, we need to configure specific elements, such as meta tags, sitemaps, and Open Graph metadata. These elements play a crucial role in how search engines index and display our web pages in search results.
2. Leveraging Meta Tags for SEO
Meta tags provide essential information to search engines about the content and structure of a web page. By correctly utilizing meta tags, we can influence how search engines perceive and present our website in search results.
2.1. Setting Title and Description Tags
The title tag (<title>) is one of the most critical meta tags for SEO. It defines the title of a web page and appears as the clickable headline in search engine results. To optimize the title tag in NEXT.js, we can utilize the “Head” component provided by the “next/head” module.
jsx // pages/index.js import Head from 'next/head'; const HomePage = () => { return ( <> <Head> <title>Your Website Title</title> <meta name="description" content="Your website description for search engines." /> </Head> {/* Rest of the page content */} </> ); }; export default HomePage;
2.2. Defining Canonical URLs
The canonical tag (rel=”canonical”) is used to specify the preferred version of a web page when multiple URLs have the same content. It helps prevent duplicate content issues, which can negatively impact SEO. To implement the canonical tag in NEXT.js, we can update our Head component as follows:
jsx // pages/blog/[slug].js import Head from 'next/head'; const BlogPost = ({ title, content, canonicalURL }) => { return ( <> <Head> <title>{title}</title> <meta name="description" content={content} /> {canonicalURL && <link rel="canonical" href={canonicalURL} />} </Head> {/* Rest of the page content */} </> ); }; export default BlogPost;
In this example, we pass the “canonicalURL” prop to the “BlogPost” component, indicating the preferred URL for the current blog post.
2.3. Implementing Robots Meta Tags
Robots meta tags (meta name=”robots”) allow us to control how search engine crawlers interact with our web pages. We can instruct crawlers to index, follow, or ignore specific pages. This is helpful for preventing sensitive pages from being indexed or avoiding crawling certain parts of the website.
jsx // pages/sensitive-page.js import Head from 'next/head'; const SensitivePage = () => { return ( <> <Head> <title>Sensitive Page</title> <meta name="robots" content="noindex, nofollow" /> </Head> {/* Rest of the page content */} </> ); }; export default SensitivePage;
In this example, the “noindex” and “nofollow” directives instruct crawlers not to index or follow any links on the “SensitivePage.”
3. Creating an SEO-friendly Sitemap
A sitemap is an XML file that lists all the pages on a website, allowing search engines to crawl and index them more efficiently. By providing a sitemap, we can ensure that search engines discover and understand the structure of our website.
3.1. Installing Sitemap Package
To generate dynamic sitemaps in NEXT.js, we can use the “next-sitemap” package. First, let’s install it:
bash npm install next-sitemap
3.2. Generating Dynamic Sitemaps
Next, we need to configure the sitemap in our NEXT.js project. Create a “next-sitemap.js” file in the root directory with the following content:
jsx // next-sitemap.js module.exports = { siteUrl: 'https://www.example.com', generateRobotsTxt: true, // Other configuration options };
Here, we set the “siteUrl” to our website’s URL, and “generateRobotsTxt” to true to generate the robots.txt file along with the sitemap.
Next, we can update our “next.config.js” to include the sitemap plugin:
jsx // next.config.js const withSitemap = require('next-sitemap'); module.exports = withSitemap({ // Your NEXT.js configuration options });
With these configurations in place, we can now run the following command to generate the sitemap:
bash npx next-sitemap
This command will create a “sitemap.xml” file in the public directory of your NEXT.js project.
3.3. Submitting Sitemaps to Search Engines
Once the sitemap is generated, we should submit it to major search engines like Google and Bing. This step ensures that search engines regularly crawl and index our website’s pages.
For Google, you can submit the sitemap through Google Search Console:
- Sign in to your Google Search Console account.
- Select your website property.
- Navigate to “Sitemaps” under “Index” on the left-hand side.
- Enter “sitemap.xml” in the provided field and click “Submit.”
For Bing, you can submit the sitemap through Bing Webmaster Tools:
- Sign in to your Bing Webmaster Tools account.
- Select your website from the dashboard.
- Click “Sitemaps” on the left-hand side.
- Enter your sitemap URL and click “Submit.”
4. Utilizing Open Graph for Social Media
Open Graph metadata enables better integration of web pages when shared on social media platforms like Facebook, Twitter, and LinkedIn. By setting Open Graph tags, we control how our content appears when shared, including the title, description, and thumbnail image.
4.1. Installing Open Graph Package
To simplify the process of adding Open Graph tags to our NEXT.js project, we can use the “next-opengraph” package:
bash npm install next-opengraph
4.2. Adding Open Graph Tags
After installing the package, we can add the necessary Open Graph tags to our page components. Let’s modify the “HomePage” component to include Open Graph tags:
jsx // pages/index.js import Head from 'next/head'; const HomePage = () => { return ( <> <Head> <title>Your Website Title</title> <meta name="description" content="Your website description for search engines." /> {/* Open Graph tags */} <meta property="og:title" content="Your Website Title" /> <meta property="og:description" content="Your website description for social media sharing." /> <meta property="og:image" content="https://www.example.com/og-image.jpg" /> <meta property="og:url" content="https://www.example.com" /> <meta property="og:type" content="website" /> </Head> {/* Rest of the page content */} </> ); }; export default HomePage;
In this example, we included Open Graph tags such as “og:title,” “og:description,” “og:image,” “og:url,” and “og:type.” Replace the “content” attributes with relevant information for your website. The “og:image” tag should point to the URL of the image that you want to display as the thumbnail when your website is shared on social media platforms.
4.3. Testing Open Graph Metadata
To ensure that the Open Graph metadata is correctly implemented, we can use the Open Graph Debugger tool provided by Facebook. This tool allows us to preview how our web pages will look when shared on Facebook and helps troubleshoot any issues with the Open Graph tags.
Follow these steps to use the Open Graph Debugger:
- Go to the Open Graph Debugger tool at https://developers.facebook.com/tools/debug/.
- Enter the URL of the web page you want to test in the provided field and click “Debug.”
- Review the information displayed, including the title, description, and thumbnail image.
- If there are any issues, the tool will provide suggestions on how to fix them.
Conclusion
In this blog, we explored essential SEO practices within NEXT.js, focusing on optimizing meta tags, sitemaps, and Open Graph metadata. By leveraging these techniques, you can significantly enhance your website’s visibility on search engines and improve its presentation when shared on social media platforms.
Remember to carefully craft title and description tags to accurately represent your web pages to both search engines and users. Use canonical tags to consolidate duplicate content and robots meta tags to control search engine crawler behavior on specific pages.
Additionally, generate dynamic sitemaps to help search engines discover and crawl all the pages on your website efficiently. By submitting the sitemap to search engine webmaster tools, you can ensure regular updates and better indexing.
Finally, utilize Open Graph tags to customize how your web pages appear when shared on social media platforms. A compelling title, description, and eye-catching thumbnail image can significantly impact click-through rates and engagement.
SEO is an ongoing process, and staying up-to-date with the latest best practices is essential. Keep monitoring your website’s performance and make necessary adjustments to maintain a competitive edge in search engine rankings.
Implementing these SEO strategies in NEXT.js will not only boost your website’s visibility but also improve user experience and drive more organic traffic to your web pages. Happy optimizing!
Table of Contents
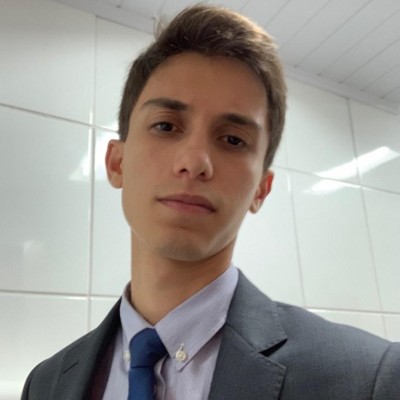
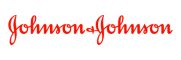