Web App Performance Redefined: NEXT.js and Prisma Unleashed
In the fast-paced world of modern web development, it’s crucial to have tools and technologies that can streamline your workflow and enhance the performance of your applications. For early-stage startup founders, VC investors, and tech leaders, staying ahead of the curve is essential. That’s where technologies like NEXT.js and Prisma come into play. In this blog post, we’ll explore how to harness the power of NEXT.js with Prisma to build high-performance web applications. So, grab your virtual shovels, fellow tech enthusiasts, because we’re about to dig deep into this powerful integration!
Table of Contents
1. NEXT.js and Prisma: A Match Made in Tech Heaven
Before we dive into the nitty-gritty details, let’s understand what NEXT.js and Prisma bring to the table:
– NEXT.js: NEXT.js is a popular React framework that simplifies the process of building server-rendered React applications. It offers server-side rendering, static site generation, and other features that optimize the performance of your web apps.
– Prisma: Prisma is a modern database ORM (Object-Relational Mapping) tool that simplifies database interactions. It supports multiple databases, including PostgreSQL, MySQL, and SQLite, making it a versatile choice for developers.
2. Getting Started with NEXT.js and Prisma
2.1. Setting Up Your NEXT.js Project
To begin, create a new NEXT.js project or use an existing one. If you’re starting fresh, you can use the following command to set up a new NEXT.js project:
```bash npx create-next-app my-next-prisma-app ```
2.2. Adding Prisma to Your Project
Next, we’ll integrate Prisma into our project. Prisma makes it easy to work with databases by auto-generating TypeScript-based query builders. Install Prisma and its dependencies using the following commands:
```bash npm install prisma @prisma/client ```
2.3. Database Configuration
Configure Prisma to connect to your preferred database. Create a `schema.prisma` file and define your data model. Here’s an example using PostgreSQL:
```prisma // schema.prisma generator client { provider = "prisma-client-js" output = "./generated/client" } datasource db { provider = "postgresql" url = env("DATABASE_URL") } model User { id Int @id @default(autoincrement()) name String email String @unique posts Post[] } model Post { id Int @id @default(autoincrement()) title String body String userId Int user User @relation(fields: [userId], references: [id]) } ```
2.4. Generating Prisma Client
After defining your schema, generate the Prisma Client by running:
```bash npx prisma generate ```
This command will generate a TypeScript-based Prisma Client that you can use to interact with your database.
2.5. Using Prisma in Your NEXT.js Application
Now that Prisma is set up, you can start using it in your NEXT.js application. Here’s an example of how to fetch data from your database and display it in your application:
```javascript import { PrismaClient } from '@prisma/client' const prisma = new PrismaClient() export default function Home({ posts }) { return ( <div> <h1>Welcome to My Prisma-Powered NEXT.js App</h1> <ul> {posts.map((post) => ( <li key={post.id}> <h2>{post.title}</h2> <p>{post.body}</p> </li> ))} </ul> </div> ) } export async function getStaticProps() { const posts = await prisma.post.findMany() return { props: { posts, }, } } ```
3. External Resources for Further Learning
To deepen your understanding of NEXT.js and Prisma, here are three external resources worth exploring:
- Official NEXT.js Documentation – https://nextjs.org/docs – The official documentation provides comprehensive guides and examples to help you master NEXT.js.
- Prisma Documentation – https://www.prisma.io/docs – Dive into the Prisma documentation to learn about its features, queries, and best practices.
- Introduction to NEXT.js Course on Udemy – https://www.udemy.com/course/nextjs-react-the-complete-guide – If you prefer video tutorials, this Udemy course offers a comprehensive introduction to NEXT.js.
Conclusion
Combining NEXT.js with Prisma can supercharge your web development projects, whether you’re an early-stage startup founder, VC investor, or tech leader. With server-side rendering, database interactions, and a robust ecosystem of libraries at your disposal, you’ll be well-equipped to outperform the competition and build cutting-edge web applications.
So, go ahead, experiment with this powerful duo, and take your web development game to the next level! Happy coding!
Table of Contents
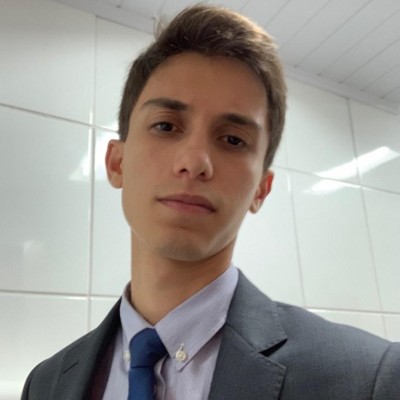
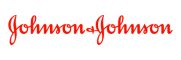