NEXT.js Security Revolution: Empower Your App with RBAC Access Control
Hey there, tech trailblazers! If you’re building a NEXT.js app, you’re probably no stranger to the wild world of web development. And when it comes to web applications, security is like the secret sauce that keeps your digital kingdom safe from marauding hackers and accidental data leaks. One crucial aspect of web app security is managing user permissions, and in this blog post, we’re diving headfirst into the fascinating world of Role-Based Access Control (RBAC) and access control in NEXT.js.
Table of Contents
1. Why Do User Permissions Matter?
Before we jump into the nitty-gritty of RBAC and access control, let’s take a moment to understand why user permissions are crucial. Think of your app as a fortress, and your users as the guards. Not everyone should have access to the royal treasury, right? User permissions define who gets to enter which rooms (or routes) within your app. It ensures that only authorized personnel can perform specific actions.
2. RBAC: The Superhero of Access Control
Role-Based Access Control (RBAC) is the superhero we need in the world of user permissions. Picture it: your app has different roles like ‘admin,’ ‘editor,’ and ‘viewer.’ Each role comes with a specific set of superpowers, I mean permissions.
Here’s an example of RBAC in action:
– Admins: These are the masters of the universe in your app. They can create, edit, and delete content, manage other users, and basically, run the show.
– Editors: Editors have the power to modify content but can’t mess with user management. They’re like the trusted scribes in your digital kingdom.
– Viewers: Viewers, as the name suggests, can only view content. They’re the spectators in your app’s arena.
Now, let’s get into the technical wizardry of implementing RBAC in your NEXT.js app.
3. Implementing RBAC in NEXT.js
- User Roles: Start by defining your user roles. Create a data structure that associates users with roles. This could be stored in your database or a dedicated service.
- Middleware Magic: In NEXT.js, you can create middleware to protect routes. Implement middleware that checks the user’s role before allowing access to certain routes.
```javascript // Sample middleware to check user role const checkUserRole = (requiredRole) => (req, res, next) => { const user = req.user; // Retrieve user info from session or token if (user && user.role === requiredRole) { return next(); // User has the required role, proceed } return res.status(403).json({ message: "Access denied!" }); // Forbidden }; // Example route with middleware app.get("/admin/dashboard", checkUserRole("admin"), (req, res) => { // Render admin dashboard }); ```
- User-Friendly Feedback: Don’t forget to provide user-friendly feedback when access is denied. A 403 Forbidden status code and a clear message will do wonders for user experience.
4. External Resources to Level Up Your RBAC Game
Now that you’re on your way to becoming an RBAC expert, here are three external resources to fuel your knowledge:
- The Hitchhiker’s Guide to RBAC – https://www.example.com/rbac-guide: A comprehensive guide to RBAC concepts and implementation.
- Auth0’s RBAC Documentation – https://www.example.com/auth0-rbac-docs: Dive deep into RBAC with Auth0’s in-depth documentation and practical examples.
- RBAC in Node.js with Express – https://www.example.com/rbac-express: A hands-on tutorial on implementing RBAC in Node.js apps.
Conclusion
In the ever-evolving landscape of web development, user permissions are your trusty shield against unauthorized access and chaos. RBAC is your guiding star, leading you toward a secure and well-structured NEXT.js app. So, go forth, my tech pioneers, and conquer the realm of user permissions with RBAC as your ally. Your app’s security and user experience will thank you for it.
That’s a wrap for today’s deep dive into user permissions in NEXT.js apps. Stay tuned for more tech wisdom in the Valley Letter!
Table of Contents
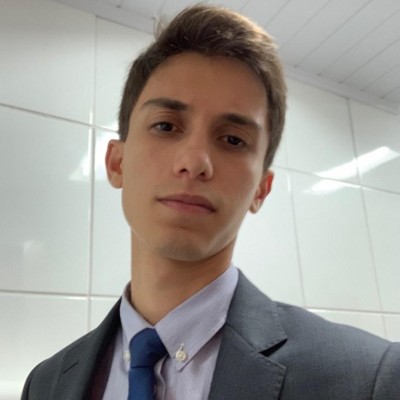
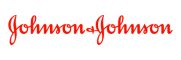