Building a Real-time Dashboard with NEXT.js and Chart.js
In today’s data-driven world, the ability to visualize data in real-time is a crucial aspect of decision-making and analysis. Real-time dashboards provide a dynamic and interactive way to monitor changes and trends as they happen. In this tutorial, we’ll walk through the process of creating a real-time dashboard using NEXT.js, a popular React framework, and Chart.js, a versatile JavaScript charting library. By the end of this guide, you’ll have the knowledge and tools to build your own data visualization dashboard.
1. Prerequisites
Before we dive into the implementation, make sure you have a basic understanding of HTML, CSS, JavaScript, React, and Node.js. Additionally, ensure that you have Node.js and npm (Node Package Manager) installed on your machine.
2. Setting Up the Project
To get started, let’s create a new NEXT.js project. Open your terminal and run the following commands:
bash npx create-next-app real-time-dashboard cd real-time-dashboard
This will set up a new NEXT.js project in a directory named real-time-dashboard. Navigate to the project directory using cd real-time-dashboard.
3. Adding Dependencies
To integrate Chart.js into our project, we need to install the required packages. Run the following command to install Chart.js and its React wrapper:
bash npm install chart.js react-chartjs-2
4. Creating the Real-time Dashboard Component
Now that we have our project set up and the necessary packages installed, let’s start building our real-time dashboard component. In the pages directory, create a new file named dashboard.js. This will be our dashboard’s main page.
jsx // pages/dashboard.js import React, { useState, useEffect } from 'react'; import { Line } from 'react-chartjs-2'; const Dashboard = () => { const [data, setData] = useState({ labels: [], datasets: [ { label: 'Real-time Data', data: [], borderColor: 'rgba(75, 192, 192, 1)', backgroundColor: 'rgba(75, 192, 192, 0.2)', }, ], }); // Simulate real-time data updates useEffect(() => { const interval = setInterval(() => { const newLabel = new Date().toLocaleTimeString(); const newData = Math.floor(Math.random() * 100); setData((prevData) => ({ labels: [...prevData.labels, newLabel], datasets: [ { ...prevData.datasets[0], data: [...prevData.datasets[0].data, newData], }, ], })); }, 2000); // Update every 2 seconds return () => clearInterval(interval); }, []); return ( <div className="dashboard"> <h1>Real-time Dashboard</h1> <Line data={data} /> </div> ); }; export default Dashboard;
In this component, we’ve used the useState and useEffect hooks from React to manage the data and simulate real-time updates. The Line component from react-chartjs-2 is used to render the line chart. We’re updating the chart data every 2 seconds for demonstration purposes, but you can replace this logic with actual data fetching and updates.
5. Styling the Dashboard
Let’s add some basic styling to our dashboard to make it visually appealing. Create a file named dashboard.module.css in the styles directory and add the following CSS:
css /* styles/dashboard.module.css */ .dashboard { text-align: center; padding: 2rem; } h1 { font-size: 2rem; margin-bottom: 1rem; } canvas { max-width: 100%; border: 1px solid #ddd; }
6. Running the Application
With the component and styles in place, it’s time to see our real-time dashboard in action. In your terminal, run the following command:
bash npm run dev
This command starts the development server, and you can access the dashboard by opening your browser and navigating to http://localhost:3000/dashboard.
7. Customizing the Dashboard
Now that you have a working real-time dashboard, you can customize it further based on your needs. Here are a few ideas:
7.1. Data Fetching
Instead of simulating data, you can fetch real data from APIs or databases. Utilize libraries like axios or the built-in fetch API to fetch data at regular intervals and update the chart accordingly.
7.2. Multiple Charts
Extend the dashboard to display multiple charts representing different data sets. You can add additional Line or other chart components and manage their data independently.
7.3. Additional Chart Types
Chart.js supports various chart types like bar charts, pie charts, and more. Experiment with different chart types to visualize your data effectively.
7.4. Styling and Theming
Enhance the visual appeal of your dashboard by exploring Chart.js customization options and applying your own CSS styles. You can also implement theming to match your application’s design.
Conclusion
In this tutorial, we’ve explored the process of building a real-time dashboard using NEXT.js and Chart.js. We started by setting up a new NEXT.js project, installing the required packages, and creating a dynamic dashboard component. Through the use of React hooks and the react-chartjs-2 library, we simulated real-time data updates and visualized them using a line chart. Additionally, we touched on styling and customization options to make the dashboard uniquely yours.
Real-time dashboards provide valuable insights into evolving data trends, making them a powerful tool for businesses and developers alike. By following the steps outlined in this guide, you now have the foundation to create your own real-time data visualization tools and take your data analysis to the next level. So go ahead, experiment, and build impressive real-time dashboards that empower better decision-making.
Table of Contents
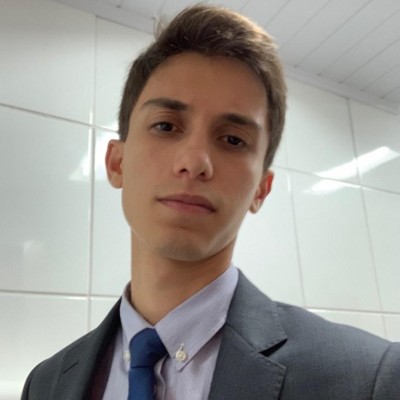
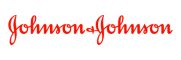