Implementing Real-time Notifications in NEXT.js with WebSockets
In today’s fast-paced digital world, user engagement and real-time updates are crucial for the success of web applications. Implementing real-time notifications can significantly enhance the user experience by keeping users informed about relevant events as they happen. In this tutorial, we’ll explore how to implement real-time notifications in a NEXT.js application using WebSockets. By the end of this guide, you’ll be able to create a seamless and engaging user experience that ensures users are always up-to-date with the latest information.
1. Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites:
- Basic understanding of JavaScript and React.
- Familiarity with NEXT.js framework.
- Node.js and npm (Node Package Manager) installed on your system.
2. Setting Up the Project
To get started, let’s set up a new NEXT.js project or use an existing one. Open your terminal and execute the following commands:
bash npx create-next-app real-time-notifications cd real-time-notifications
This will create a new NEXT.js project called “real-time-notifications” and navigate into its directory.
3. Installing Dependencies
We’ll need a WebSocket library to establish real-time communication between the server and clients. For this tutorial, we’ll use the popular socket.io library. Install it by running the following command:
bash npm install socket.io
4. Creating the WebSocket Server
Next, let’s set up the WebSocket server. Create a new file named server.js in the root of your project. This file will handle WebSocket connections and notifications broadcasting.
javascript // server.js const http = require('http'); const { Server } = require('socket.io'); const server = http.createServer(); const io = new Server(server, { cors: { origin: '*', }, }); io.on('connection', (socket) => { console.log('A user connected'); socket.on('disconnect', () => { console.log('A user disconnected'); }); }); server.listen(3001, () => { console.log('WebSocket server is listening on port 3001'); });
In this code, we create an HTTP server and attach the socket.io server to it. We allow all origins for the WebSocket connection to keep things simple for this tutorial. When a user connects, a message is logged, and when a user disconnects, another message is logged.
5. Integrating WebSocket Server with NEXT.js
Now that we have our WebSocket server, let’s integrate it with our NEXT.js application. Open the pages/_app.js file in your project and import the io instance from the WebSocket server.
javascript // pages/_app.js import { useEffect } from 'react'; import { io } from 'socket.io-client'; function MyApp({ Component, pageProps }) { useEffect(() => { const socket = io('http://localhost:3001'); socket.on('connect', () => { console.log('Connected to WebSocket server'); }); return () => { socket.disconnect(); }; }, []); return <Component {...pageProps} />; } export default MyApp;
In this code, we import the io function from the socket.io-client library and establish a connection to our WebSocket server when the application loads. We log a message when the connection is established and disconnect the socket when the component is unmounted.
6. Emitting and Receiving Notifications
With the WebSocket connection established, we can now emit and receive notifications between the server and clients. Let’s modify our server-side code to emit notifications whenever a certain event occurs.
javascript // server.js io.on('connection', (socket) => { console.log('A user connected'); socket.on('disconnect', () => { console.log('A user disconnected'); }); // Emit a sample notification after 5 seconds setTimeout(() => { socket.emit('notification', { message: 'New content is available!', }); }, 5000); });
In this example, we emit a sample notification to the connected client after 5 seconds. Now, let’s modify our client-side code to receive and display these notifications.
javascript // pages/_app.js import { useEffect, useState } from 'react'; import { io } from 'socket.io-client'; function MyApp({ Component, pageProps }) { const [notifications, setNotifications] = useState([]); useEffect(() => { const socket = io('http://localhost:3001'); socket.on('connect', () => { console.log('Connected to WebSocket server'); }); socket.on('notification', (data) => { setNotifications([...notifications, data]); }); return () => { socket.disconnect(); }; }, [notifications]); return ( <div> <h1>Real-time Notifications Example</h1> <Component {...pageProps} /> <div> <h2>Notifications</h2> <ul> {notifications.map((notification, index) => ( <li key={index}>{notification.message}</li> ))} </ul> </div> </div> ); } export default MyApp;
In this code, we use the useState hook to manage an array of notifications. When a notification event is received from the WebSocket server, we update the state to include the new notification. The notifications are then displayed in a list on the webpage.
7. Enhancing the Implementation
This basic example demonstrates the fundamentals of implementing real-time notifications in a NEXT.js application using WebSockets. However, there are various ways to enhance this implementation to suit your application’s needs:
- Authentication and Authorization: Implement authentication and authorization mechanisms to ensure that notifications are delivered only to authorized users.
- Different Types of Notifications: Extend the notification payload to include different types of notifications, such as messages, friend requests, or activity updates.
- Real Database Integration: Replace the sample notifications with real-time updates from a database, such as new comments, likes, or posts.
- Styling and UI: Customize the notification display to match your application’s design and branding.
- Error Handling: Implement error handling to gracefully handle situations where the WebSocket connection is lost or encounters errors.
Conclusion
Implementing real-time notifications in a NEXT.js application using WebSockets can significantly enhance user engagement and create a more dynamic user experience. By following this tutorial, you’ve learned the basics of setting up a WebSocket server, integrating it with your NEXT.js application, emitting and receiving notifications, and displaying them to users in real time. Remember that this is just a starting point, and you can further tailor the implementation to meet the specific requirements of your application. Real-time notifications not only keep users informed but also contribute to a more interactive and engaging application overall.
Table of Contents
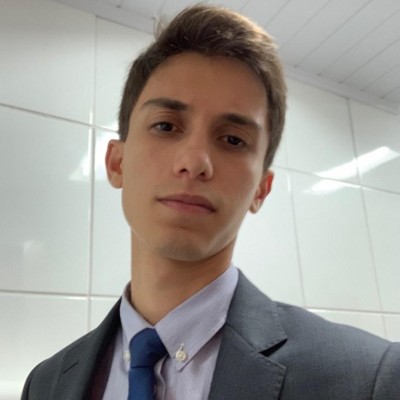
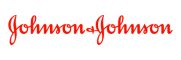