Serverless Authentication with AWS Amplify and NEXT.js
In today’s fast-paced digital world, web applications need to provide a seamless and secure authentication experience for users. Implementing authentication from scratch can be complex and time-consuming. However, with the powerful combination of AWS Amplify and NEXT.js, you can effortlessly add serverless authentication to your applications, ensuring that your users’ data remains safe while simplifying the development process.
In this blog, we will walk through the process of integrating serverless authentication using AWS Amplify and NEXT.js. AWS Amplify is a set of tools and services provided by Amazon Web Services (AWS) to build scalable and secure applications. NEXT.js is a popular React framework that simplifies server-side rendering and enables the creation of modern web applications.
Let’s get started with the step-by-step guide to integrate serverless authentication into your NEXT.js application using AWS Amplify.
Prerequisites
Before we dive into the implementation, make sure you have the following prerequisites set up:
- Node.js and npm installed on your local development environment.
- A basic understanding of React and NEXT.js.
- An AWS account with access to AWS services.
Step 1: Set up the NEXT.js Project
If you already have a NEXT.js project set up, you can skip this step. Otherwise, follow the steps below to create a new NEXT.js project:
bash npx create-next-app my-auth-app cd my-auth-app
Step 2: Install and Configure AWS Amplify
Next, we need to install and configure AWS Amplify in our project. AWS Amplify provides a convenient command-line interface (CLI) that helps us set up authentication and other services easily.
First, let’s install the AWS Amplify CLI globally:
bash npm install -g @aws-amplify/cli
Now, we need to configure AWS Amplify with our AWS account:
bash amplify configure
Follow the prompts to sign in to your AWS account and create a new IAM user with the necessary permissions for Amplify.
Once the configuration is complete, we can initialize Amplify in our NEXT.js project:
bash amplify init
Follow the prompts, and choose the options that suit your project. Amplify will create a new IAM user, an S3 bucket, and a DynamoDB table to store our application data.
Step 3: Add Authentication to the NEXT.js App
With AWS Amplify set up, we can now add authentication to our NEXT.js application. Amplify provides a high-level API for authentication, making it straightforward to integrate into our application.
Let’s add authentication using the following command:
bash amplify add auth
Again, follow the prompts to choose the authentication configuration that best fits your needs. Amplify supports various authentication methods like username/password, social media sign-ins, and more.
Once the authentication configuration is added, apply the changes to your AWS account:
bash amplify push
Step 4: Implement Authentication in the NEXT.js App
Now that we have authentication set up on the AWS side, we need to implement the frontend integration in our NEXT.js app.
First, we need to install the AWS Amplify library in our project:
bash npm install aws-amplify @aws-amplify/ui-react
Next, we will configure the Amplify library and set up authentication in our app. Open the pages/\_app.js file and add the following code:
jsx import React from 'react'; import { Amplify } from 'aws-amplify'; import { AmplifyAuthenticator, AmplifySignUp, AmplifySignIn } from '@aws-amplify/ui-react'; import awsconfig from '../src/aws-exports'; Amplify.configure(awsconfig); function MyApp({ Component, pageProps }) { return ( <AmplifyAuthenticator> <AmplifySignUp slot="sign-up" formFields={[ { type: 'username' }, { type: 'password' }, { type: 'email' }, ]} /> <AmplifySignIn slot="sign-in" /> <Component {...pageProps} /> </AmplifyAuthenticator> ); } export default MyApp;
In this code, we import the necessary components from the Amplify library and configure it with our awsconfig, which is automatically generated by Amplify.
We use the AmplifyAuthenticator component to wrap our application. This component handles the authentication flow, showing sign-up and sign-in forms based on the user’s current authentication status.
The AmplifySignUp component provides a sign-up form with fields for username, password, and email. The AmplifySignIn component displays the sign-in form. Both forms are automatically wired up to the backend AWS services we set up earlier.
Now, when you run your NEXT.js app, you should see the authentication forms when accessing the pages that require authentication.
Step 5: Protecting Routes with Authentication
In a real-world application, you would want to protect certain routes and make them accessible only to authenticated users. With Amplify, protecting routes is a breeze.
Let’s say we have a page called dashboard.js that we want to protect. Open the pages/dashboard.js file and add the following code:
jsx import React from 'react'; import { withAuthenticator } from '@aws-amplify/ui-react'; function Dashboard() { return ( <div> <h1>Welcome to the Dashboard!</h1> {/* Your dashboard content goes here */} </div> ); } export default withAuthenticator(Dashboard);
With the withAuthenticator higher-order component, the Dashboard page is now protected. If a user tries to access this page without being authenticated, Amplify will automatically redirect them to the sign-in page.
Conclusion
Congratulations! You’ve successfully implemented serverless authentication in your NEXT.js application using AWS Amplify. By leveraging the power of AWS Amplify and NEXT.js, you’ve made your application more secure and scalable while reducing development time.
Now, you can focus on building more features and functionalities for your app, knowing that authentication is taken care of by AWS Amplify. Happy coding!
Table of Contents
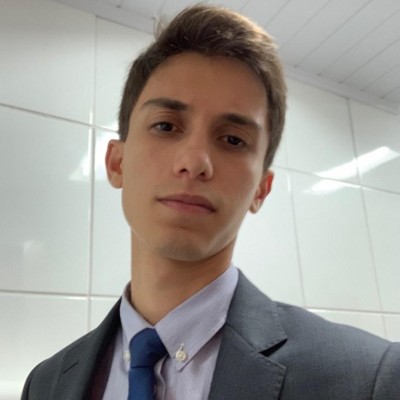
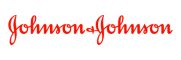