Exploring the Serverless Database Realm with NEXT.js
In the ever-evolving landscape of web development, serverless architecture has emerged as a game-changer. It offers scalability, cost-efficiency, and ease of management that traditional server-based approaches struggle to match. When paired with a framework like NEXT.js, which is renowned for its flexibility and performance, serverless architecture becomes even more potent.
In this blog post, we’ll delve into the realm of serverless databases and explore how to harness their capabilities with NEXT.js. We’ll cover the basics, benefits, and step-by-step implementation to help you leverage this powerful combination for your web applications.
1. Understanding Serverless Databases
1.1. What are Serverless Databases?
Serverless databases are a paradigm shift in database management. They eliminate the need for you to provision and manage database servers, allowing you to focus solely on your application code. These databases automatically scale, handle high availability, and ensure fault tolerance without requiring manual intervention.
Key characteristics of serverless databases include:
- Automatic Scaling: Serverless databases can scale up or down based on demand, ensuring your application can handle traffic spikes and save costs during idle periods.
- Pay-as-You-Go Pricing: With serverless databases, you pay only for the resources you consume. This makes it a cost-effective choice, especially for startups and small businesses.
- Managed Infrastructure: Serverless databases are fully managed by cloud providers, reducing the operational burden on your team. You can focus on writing code instead of managing servers.
2. Benefits of Serverless Databases
2.1. Cost Efficiency
One of the most significant advantages of serverless databases is their cost-efficiency. Traditional databases require you to provision and pay for a fixed amount of server resources, whether you use them or not. In contrast, serverless databases charge you based on actual usage, making it an economical choice, especially for applications with variable workloads.
2.2. Scalability
Serverless databases can seamlessly handle sudden spikes in traffic. They automatically scale resources to accommodate increased demand, ensuring your application remains responsive even during traffic surges. This scalability is crucial for applications with unpredictable usage patterns.
2.3. Simplified Management
Managing database servers can be a complex and time-consuming task. Serverless databases eliminate this overhead by abstracting server management, allowing you to focus on building and improving your application.
2.4. High Availability
Serverless databases are designed for high availability. They replicate data across multiple data centers and automatically fail over in case of hardware failures or other issues, ensuring your data is always accessible.
3. Use Cases
Serverless databases are versatile and can be used in various scenarios:
3.1. Web Applications
Serverless databases are an excellent choice for web applications, especially those built with frameworks like NEXT.js. They provide the necessary flexibility and scalability to handle changing user loads and evolving data requirements.
3.2. Mobile Apps
Mobile applications that require backend data storage can benefit from serverless databases. They ensure that your app’s backend infrastructure scales with your user base, providing a smooth user experience.
4.3. IoT Applications
Internet of Things (IoT) applications often generate massive amounts of data. Serverless databases can handle this data influx efficiently, making them suitable for IoT use cases.
In the next section, we’ll explore how to integrate a serverless database with a NEXT.js application.
4. Getting Started with NEXT.js
Before we dive into serverless databases, let’s set up a NEXT.js project and create a simple web application to work with. If you already have a NEXT.js project, feel free to skip this section.
4.1. Setting Up a NEXT.js Project
To create a new NEXT.js project, follow these steps:
Step 1: Install Node.js
If you haven’t already, install Node.js on your machine. Node.js is required for running JavaScript on the server and managing project dependencies.
Step 2: Create a New NEXT.js Project
Open your terminal and run the following command to create a new NEXT.js project:
bash npx create-next-app my-next-app
This command sets up a new NEXT.js project with the name “my-next-app.” You can replace “my-next-app” with your preferred project name.
Step 3: Navigate to the Project Directory
Change your working directory to the newly created project folder:
bash cd my-next-app
4.2. Creating a Simple Web Application
Now that your NEXT.js project is set up, let’s create a simple web application. We’ll start with a basic “Hello, World!” example.
Step 1: Open the “pages” Directory
In your project folder, you’ll find a “pages” directory. This directory contains your application’s pages. Let’s create a new page called “index.js.”
Step 2: Create the “index.js” File
Inside the “pages” directory, create a file named “index.js” and add the following code:
jsx // pages/index.js import React from 'react'; function HomePage() { return ( <div> <h1>Hello, World!</h1> </div> ); } export default HomePage;
This code defines a simple React component that displays the text “Hello, World!” on the homepage.
Step 3: Run Your NEXT.js Application
You can now start your NEXT.js development server by running the following command:
bash npm run dev
Your NEXT.js application will be available at http://localhost:3000. Open this URL in your web browser to see your “Hello, World!” message.
With our basic NEXT.js application in place, let’s move on to integrating a serverless database.
5. Integrating a Serverless Database with NEXT.js
To integrate a serverless database with NEXT.js, you need to follow these steps:
5.1. Choosing the Right Serverless Database
The first decision you’ll need to make is selecting a serverless database that suits your project’s requirements. Some popular options include:
- Firebase Realtime Database: Firebase provides a real-time database that’s easy to set up and works seamlessly with JavaScript frameworks like NEXT.js.
- AWS DynamoDB: Amazon’s DynamoDB is a fully managed NoSQL database service that offers serverless capabilities. It’s highly scalable and suitable for various applications.
- Azure Cosmos DB: Microsoft’s Azure Cosmos DB is a globally distributed, multi-model database service. It supports various APIs, making it versatile for different development needs.
For this example, let’s choose Firebase Realtime Database.
5.2. Connecting Your NEXT.js App to the Database
To connect your NEXT.js app to Firebase Realtime Database, follow these steps:
Step 1: Create a Firebase Project
If you don’t have a Firebase project, go to the Firebase Console and create a new project.
Step 2: Add Your App to Firebase
Once your project is created, click on “Add app” and select the web platform.
Step 3: Configure Firebase
Follow the setup instructions provided by Firebase to add the Firebase configuration to your NEXT.js app. This typically involves adding a configuration object to your app’s code.
Here’s an example of how you might configure Firebase in your NEXT.js app:
javascript // In your NEXT.js app, create a file named "firebase.js" for Firebase configuration. import firebase from 'firebase/app'; import 'firebase/database'; const firebaseConfig = { apiKey: 'YOUR_API_KEY', authDomain: 'YOUR_AUTH_DOMAIN', databaseURL: 'YOUR_DATABASE_URL', projectId: 'YOUR_PROJECT_ID', storageBucket: 'YOUR_STORAGE_BUCKET', messagingSenderId: 'YOUR_MESSAGING_SENDER_ID', appId: 'YOUR_APP_ID', }; if (!firebase.apps.length) { firebase.initializeApp(firebaseConfig); } export default firebase;
Make sure to replace the placeholder values with your Firebase project’s configuration.
Step 4: Use Firebase in Your NEXT.js App
With Firebase configured, you can now use it in your NEXT.js app to perform database operations. For example, you can read and write data like this:
javascript import React, { useState, useEffect } from 'react'; import firebase from '../path-to-firebase'; function HomePage() { const [data, setData] = useState(''); useEffect(() => { // Read data from Firebase const dbRef = firebase.database().ref('your-data-path'); dbRef.on('value', (snapshot) => { const value = snapshot.val(); setData(value); }); }, []); return ( <div> <h1>Hello, World!</h1> <p>Data from Firebase: {data}</p> </div> ); } export default HomePage;
In this example, we’re reading data from Firebase and displaying it on the page. You can also write data to Firebase using the set method or perform more complex operations as needed.
5.3. CRUD Operations with Serverless Databases
Serverless databases support all the standard CRUD (Create, Read, Update, Delete) operations. Here’s a quick overview of how to perform these operations with Firebase Realtime Database:
5.3.1. Create Data:
To add data to your Firebase database, you can use the set method on a database reference.
javascript const dbRef = firebase.database().ref('your-data-path'); dbRef.set({ key: 'value' });
5.3.2. Read Data:
Reading data is achieved by listening to a reference’s value event, as shown in the previous example.
5.3.3. Update Data:
To update existing data, you can use the update method on a database reference.
javascript const dbRef = firebase.database().ref('your-data-path'); dbRef.update({ key: 'new-value' });
5.3.4. Delete Data:
Deleting data is done using the remove method on a database reference.
javascript const dbRef = firebase.database().ref('your-data-path'); dbRef.remove();
With these basic CRUD operations, you can create dynamic applications that interact with a serverless database.
In the next section, we’ll explore best practices for using serverless databases with NEXT.js.
6. Serverless Database Best Practices
When working with serverless databases in your NEXT.js applications, it’s essential to follow best practices to ensure optimal performance, security, and cost management.
6.1. Security Considerations
- Authentication and Authorization: Implement proper authentication and authorization mechanisms to restrict access to your database. Firebase, for example, provides authentication options out of the box.
- Data Validation: Validate data before writing it to the database to prevent malicious or incorrect data from being stored.
- Network Security: Ensure that your application and serverless database communicate securely over HTTPS.
6.2. Scalability and Performance Optimization
- Database Structure: Design your database structure to minimize read and write operations. Use denormalization and indexing as needed.
- Caching: Implement caching mechanisms to reduce the load on your serverless database, especially for frequently accessed data.
- Optimize Queries: Write efficient database queries to minimize the amount of data retrieved.
6.3. Cost Management
- Monitoring and Alerts: Set up monitoring and alerts to track your serverless database’s usage and costs. Many cloud providers offer cost management tools.
- Usage Analysis: Regularly review your database usage and identify opportunities to optimize queries or reduce unnecessary data storage.
- Auto-scaling: Take advantage of auto-scaling features offered by your serverless database to avoid over-provisioning and minimize costs during low-traffic periods.
By following these best practices, you can ensure that your serverless database integration with NEXT.js is both efficient and cost-effective.
7. Real-World Application: Building a Serverless Blog
To solidify your understanding of integrating serverless databases with NEXT.js, let’s embark on a real-world project: building a serverless blog.
7.1. Design and Architecture
Our serverless blog will have the following components:
- Frontend: Built using NEXT.js, it will allow users to view and interact with blog posts.
- Backend: Utilizing a serverless database (Firebase Realtime Database), it will store blog post data.
7.2. Implementation Steps
- Set Up Your NEXT.js Project: Follow the steps outlined earlier to create a new NEXT.js project.
- Design the Blog UI: Create components and pages for displaying blog posts, creating new posts, and managing content.
- Integrate Firebase: Configure Firebase in your NEXT.js app and set up the database structure to store blog post data.
- Implement CRUD Operations: Add functionality to create, read, update, and delete blog posts using Firebase.
- Authentication: Implement user authentication to allow authors to manage their posts.
- Styling: Style your blog to make it visually appealing and user-friendly.
- Deployment: Deploy your NEXT.js app and Firebase database to make it accessible to users.
Building a serverless blog is a practical way to apply the concepts discussed in this post and gain hands-on experience.
Conclusion
In this exploration of the serverless database realm with NEXT.js, we’ve learned about the benefits and use cases of serverless databases, set up a NEXT.js project, and integrated Firebase Realtime Database into our application. We’ve also discussed best practices for security, performance, and cost management.
With the knowledge gained from this blog post, you’re well-equipped to leverage serverless databases in your NEXT.js projects and build dynamic, scalable, and cost-effective web applications. Whether you’re building blogs, e-commerce sites, or any other web application, the combination of NEXT.js and serverless databases can be a game.
Table of Contents
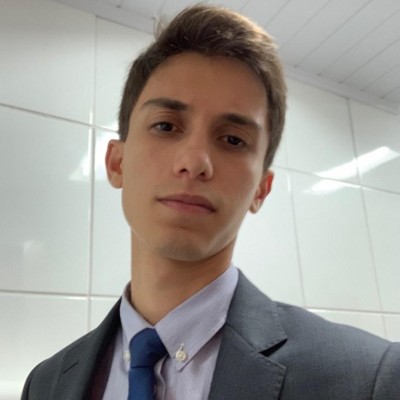
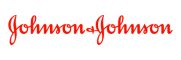