Implementing WebSockets in NEXT.js: Socket.io and WebSocket API
WebSockets have revolutionized real-time communication on the web, enabling seamless, bi-directional data exchange between clients and servers. In the world of modern web development, frameworks like Next.js have gained popularity for their ability to create dynamic and responsive applications. Combining the power of WebSockets with Next.js can take your web applications to the next level by enabling real-time features such as chat applications, live updates, and interactive gaming.
In this comprehensive guide, we’ll dive into the world of WebSockets and explore how to implement them in a Next.js application. We’ll use two popular approaches: Socket.io, a library that simplifies WebSocket implementation, and the WebSocket API, a native browser feature. By the end of this tutorial, you’ll have a solid understanding of both methods and be ready to integrate WebSockets into your Next.js projects.
1. Understanding WebSockets
1.1. What are WebSockets?
WebSockets provide a full-duplex communication channel over a single TCP connection. Unlike traditional HTTP requests, which are stateless and require constant polling for updates, WebSockets allow continuous, real-time communication between a client (typically a web browser) and a server. This bidirectional connection is perfect for scenarios where immediate data exchange is crucial, such as chat applications, online gaming, live sports updates, and collaborative tools.
1.2. Why use WebSockets in Next.js?
Next.js is a popular React framework for building server-rendered applications. While it excels at handling traditional HTTP requests, it can also integrate seamlessly with WebSockets, enhancing the real-time capabilities of your applications. Here are some compelling reasons to use WebSockets in Next.js:
- Real-Time Updates: Keep your users informed with instant updates to content or notifications.
- Live Chat: Create engaging chat applications with minimal latency.
- Interactive Gaming: Enable multiplayer gaming experiences with real-time data synchronization.
- Collaborative Tools: Build collaborative applications where multiple users can interact simultaneously.
Now that we understand the benefits, let’s start by setting up a Next.js project.
2. Setting Up a Next.js Project
Before we dive into WebSocket implementation, we need a Next.js project to work with. If you already have one, feel free to skip this section. Otherwise, follow these steps:
- Create a new Next.js project: Open your terminal and run the following command to create a new Next.js application:
bash npx create-next-app my-websocket-app
Replace my-websocket-app with your preferred project name.
- Navigate to the project directory: Move into your newly created project folder:
bash cd my-websocket-app
With our project ready, let’s explore two methods for implementing WebSockets in Next.js.
3. Implementing WebSockets with Socket.io
Socket.io is a popular library for implementing real-time communication using WebSockets. It offers a simple and robust API for handling WebSocket connections and messages. Let’s get started with Socket.io in Next.js.
3.1. Installing Socket.io
To use Socket.io in your Next.js project, you need to install it as a dependency. Open your terminal and run the following command:
bash npm install socket.io
3.2. Creating a WebSocket Server
In your Next.js project, you can create a WebSocket server using Socket.io. Here’s how to do it:
javascript // pages/api/socket.js import { Server } from 'socket.io'; export default async function handler(req, res) { if (!res.socket.server.io) { const httpServer = res.socket.server; const io = new Server(httpServer); io.on('connection', (socket) => { console.log('Client connected'); socket.on('disconnect', () => { console.log('Client disconnected'); }); }); res.socket.server.io = io; } res.end(); }
In the code above, we create a WebSocket server using Socket.io and listen for incoming connections. When a client connects, a “Client connected” message is logged, and when a client disconnects, a “Client disconnected” message is logged.
3.3. Handling WebSocket Connections
Now that we have a WebSocket server set up, let’s handle WebSocket connections in a Next.js component. Create a new React component or page (e.g., Chat.js) where you want to implement WebSocket functionality:
javascript // pages/chat.js import { useEffect } from 'react'; import io from 'socket.io-client'; export default function Chat() { useEffect(() => { const socket = io(); // Handle events here return () => { socket.disconnect(); }; }, []); return ( <div> {/* Your chat interface */} </div> ); }
In the code above, we import the io function from socket.io-client and create a WebSocket connection to our server. You can handle various events and messages within the useEffect hook.
3.4. Sending and Receiving Messages
To send and receive messages over the WebSocket connection, you can define custom events and handlers. For example, to send a message from the client to the server and vice versa:
javascript // pages/chat.js // ... export default function Chat() { useEffect(() => { const socket = io(); // Send a message to the server socket.emit('chatMessage', 'Hello, server!'); // Receive a message from the server socket.on('serverMessage', (message) => { console.log(`Server says: ${message}`); }); return () => { socket.disconnect(); }; }, []); // ... }
In this example, we emit a “chatMessage” event from the client to the server and listen for a “serverMessage” event from the server to the client.
3.5. Broadcasting Messages
Socket.io makes it easy to broadcast messages to multiple clients. To broadcast a message to all connected clients, you can use the io instance we created earlier:
javascript // pages/api/socket.js // ... io.on('connection', (socket) => { console.log('Client connected'); socket.on('disconnect', () => { console.log('Client disconnected'); }); // Broadcast a message to all connected clients socket.on('broadcastMessage', (message) => { io.emit('serverMessage', message); }); }); // …
In this example, when a client sends a “broadcastMessage” event to the server, the server broadcasts the message to all connected clients using io.emit.
Socket.io offers a wide range of features for handling real-time communication in your Next.js application. Now, let’s explore an alternative method using the WebSocket API.
4. Implementing WebSockets with the WebSocket API
The WebSocket API is a native feature of web browsers, allowing you to establish WebSocket connections without relying on external libraries like Socket.io. Here’s how you can implement WebSockets in a Next.js project using the WebSocket API.
4.1. Establishing a WebSocket Connection
To establish a WebSocket connection, you can use the WebSocket class provided by the browser. Let’s create a WebSocket connection in a Next.js component:
javascript // pages/chat.js import { useEffect } from 'react'; export default function Chat() { useEffect(() => { // Create a WebSocket connection const socket = new WebSocket('ws://localhost:3000/api/socket'); // WebSocket event handlers socket.addEventListener('open', (event) => { console.log('WebSocket connection opened', event); }); socket.addEventListener('message', (event) => { const message = event.data; console.log('Received message:', message); }); socket.addEventListener('close', (event) => { console.log('WebSocket connection closed', event); }); return () => { // Close the WebSocket connection socket.close(); }; }, []); // ... }
In the code above, we create a WebSocket connection to the server using the WebSocket constructor. We also add event listeners to handle various WebSocket events such as “open,” “message,” and “close.”
4.2. Sending and Receiving Messages
Sending and receiving messages with the WebSocket API is straightforward. You can send messages to the server using the send method and handle incoming messages in the “message” event listener:
javascript // pages/chat.js // ... export default function Chat() { useEffect(() => { const socket = new WebSocket('ws://localhost:3000/api/socket'); // Send a message to the server socket.send('Hello, server!'); // Receive a message from the server socket.addEventListener('message', (event) => { const message = event.data; console.log('Received message:', message); }); // ... return () => { socket.close(); }; }, []); // ... }
4.3. Closing the WebSocket Connection
It’s essential to close WebSocket connections when they are no longer needed to free up resources and prevent memory leaks. You can close a WebSocket connection using the close method:
javascript // pages/chat.js // ... export default function Chat() { useEffect(() => { const socket = new WebSocket('ws://localhost:3000/api/socket'); // ... return () => { // Close the WebSocket connection socket.close(); }; }, []); // ... }
With the WebSocket API, you have full control over WebSocket connections and can implement custom logic tailored to your application’s needs.
5. Choosing Between Socket.io and WebSocket API
Both Socket.io and the WebSocket API have their advantages and use cases. Choosing the right method for your Next.js project depends on your specific requirements:
5.1. Socket.io:
Pros:
Simplicity: Socket.io abstracts many complexities of WebSocket handling.
Cross-browser compatibility: Works across various browsers.
Built-in features: Includes features like reconnection and broadcasting.
Cons:
Dependency: Requires an external library.
Additional overhead: Slightly larger bundle size.
5.2. WebSocket API:
Pros:
Lightweight: No external library dependencies.
Full control: Allows custom WebSocket implementation.
Cons:
Complexity: Requires more code for advanced features.
Browser compatibility: May require additional polyfills for older browsers.
Consider your project’s complexity and requirements when choosing between Socket.io and the WebSocket API.
6. Real-World Use Cases
To illustrate the power of WebSockets in Next.js, let’s explore a couple of real-world use cases where real-time communication can greatly enhance user experiences:
6.1. Live Chat Application
Imagine building a live chat application where users can exchange messages in real time. With WebSockets, you can create a responsive chat interface where messages appear instantly, providing a seamless chatting experience.
6.2. Real-Time Collaboration Tool
For collaborative tools like online document editors or whiteboards, WebSockets enable multiple users to work together in real time. Changes made by one user are instantly reflected on the screens of all participants, allowing for true collaboration.
Conclusion
In this guide, we’ve explored two methods for implementing WebSockets in Next.js: Socket.io and the WebSocket API. You now have the knowledge and tools to enhance your Next.js applications with real-time features, such as live chat, interactive gaming, and collaborative tools.
Whether you choose Socket.io for its simplicity or opt for the WebSocket API for fine-grained control, WebSockets open up a world of possibilities for creating dynamic and engaging web applications. Experiment with these techniques, and watch your Next.js projects come to life with real-time interactions. Happy coding!
Table of Contents
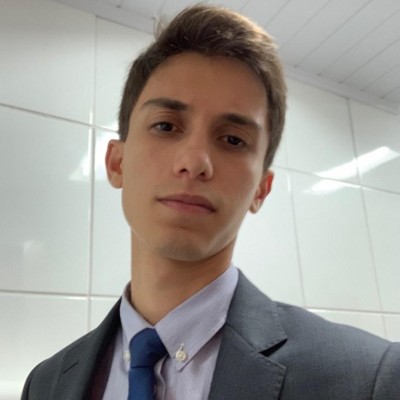
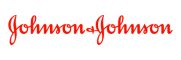