Exploring CMS Options in NEXT.js: WordPress, Ghost, and ButterCMS
In the ever-evolving landscape of web development, choosing the right Content Management System (CMS) for your NEXT.js project can be a pivotal decision. The CMS you select can significantly impact your website’s functionality, scalability, and ease of maintenance. In this comprehensive guide, we’ll take a deep dive into three popular CMS options for NEXT.js: WordPress, Ghost, and ButterCMS. We’ll explore their strengths, weaknesses, and how to integrate them seamlessly into your NEXT.js application.
1. Introduction to NEXT.js
Before delving into CMS options, let’s briefly introduce NEXT.js. NEXT.js is a powerful React framework used to build server-rendered React applications and websites. Its core features include server-side rendering (SSR), automatic code splitting, and easy deployment, making it a go-to choice for building high-performance web applications.
When it comes to building dynamic websites with NEXT.js, integrating a CMS is often a necessity. Let’s explore the top CMS options and understand which one aligns best with your project’s requirements.
2. WordPress: The Titan of CMS
Pros
- Vast Ecosystem: WordPress boasts a massive ecosystem with thousands of themes and plugins. You can find a solution for almost any feature you need.
- User-Friendly Interface: Its intuitive dashboard makes it easy for content creators and editors to manage content without technical expertise.
- SEO Capabilities: WordPress has excellent SEO plugins like Yoast SEO, helping you optimize your content for search engines.
- Community Support: You can rely on a vast community of developers, designers, and users for help and guidance.
- Customization: WordPress is highly customizable, allowing you to create unique designs and functionalities.
Cons
- Performance Challenges: As your website grows, WordPress may face performance issues, necessitating optimization efforts.
- Security Concerns: Being a popular platform, WordPress is a common target for hackers. Regular security updates are crucial.
- Learning Curve: While the dashboard is user-friendly, developers may encounter complexities when customizing themes or plugins.
- Bloat: The abundance of themes and plugins can lead to feature bloat if not managed carefully.
- Cost: While WordPress itself is free, premium themes and plugins can add up, making it costly for some projects.
3. Integrating WordPress with NEXT.js
To integrate WordPress with your NEXT.js project, you can use the WP REST API. Here’s a simple code snippet to fetch WordPress posts and display them in your NEXT.js app:
jsx // pages/index.js import { useState, useEffect } from 'react'; function Home() { const [posts, setPosts] = useState([]); useEffect(() => { // Fetch WordPress posts using the WP REST API fetch('https://your-wordpress-site.com/wp-json/wp/v2/posts') .then((response) => response.json()) .then((data) => setPosts(data)); }, []); return ( <div> <h1>Welcome to my WordPress-powered NEXT.js site!</h1> <ul> {posts.map((post) => ( <li key={post.id}>{post.title.rendered}</li> ))} </ul> </div> ); } export default Home;
With this basic setup, you can display WordPress content seamlessly in your NEXT.js application.
4. Ghost: The Minimalist’s Choice
Pros
- Simplicity: Ghost is known for its minimalist approach, offering a clean and straightforward user interface.
- Performance: Ghost is designed for speed, making it an excellent choice for fast-loading websites.
- Content Focus: It’s built specifically for blogging and content creation, ensuring your content takes center stage.
- Security: Ghost places a strong emphasis on security, reducing the risk of vulnerabilities.
- Markdown Support: If you prefer writing in Markdown, Ghost is a great fit as it natively supports it.
Cons
- Limited Ecosystem: While Ghost’s ecosystem is growing, it’s not as extensive as WordPress, which means fewer themes and plugins.
- Niche Use Case: It’s ideal for blogs and content-heavy sites but may not be the best choice for complex web applications.
- Customization Challenges: Customizing Ghost may require more technical expertise compared to WordPress.
- Cost: While there is a free self-hosted option, the hosted version comes with a subscription fee.
5. Integrating Ghost with NEXT.js
Integrating Ghost with NEXT.js is relatively straightforward. Ghost provides a Content API that allows you to fetch your content as JSON. Here’s an example of how to fetch and display Ghost posts in your NEXT.js app:
jsx // pages/index.js import { useState, useEffect } from 'react'; function Home() { const [posts, setPosts] = useState([]); useEffect(() => { // Fetch Ghost posts using the Content API fetch('https://your-ghost-site.com/ghost/api/v4/content/posts/', { headers: { 'Authorization': 'Bearer YOUR_API_KEY', }, }) .then((response) => response.json()) .then((data) => setPosts(data.posts)); }, []); return ( <div> <h1>Welcome to my Ghost-powered NEXT.js blog!</h1> <ul> {posts.map((post) => ( <li key={post.id}>{post.title}</li> ))} </ul> </div> ); } export default Home;
This code snippet demonstrates how to fetch and display Ghost content in your NEXT.js application.
6. ButterCMS: The Headless CMS Solution
Pros
- Headless Architecture: ButterCMS is a headless CMS, allowing you to use it with any front-end framework, including NEXT.js.
- Ease of Use: Its user-friendly interface makes it accessible to both developers and content creators.
- Speed: ButterCMS provides a Content Delivery Network (CDN), ensuring fast content delivery.
- SEO Features: It offers SEO-friendly features like meta tags and automatic sitemaps.
- Version Control: You can track content changes with built-in version control.
Cons
- Cost: ButterCMS is not free, and pricing may not be suitable for small projects or personal blogs.
- Limited Customization: While it’s flexible, extensive customizations may require more effort compared to self-hosted solutions.
- Smaller Ecosystem: It has a smaller community and fewer third-party integrations compared to WordPress.
- Dependency on the Service: As a hosted service, you rely on ButterCMS’s infrastructure and uptime.
7. Integrating ButterCMS with NEXT.js
ButterCMS provides a JavaScript SDK that makes integrating it with NEXT.js straightforward. Here’s a code snippet demonstrating how to fetch and display ButterCMS content:
jsx // pages/index.js import { useState, useEffect } from 'react'; import Butter from 'buttercms'; const butter = Butter('YOUR_API_KEY'); function Home() { const [posts, setPosts] = useState([]); useEffect(() => { // Fetch ButterCMS posts butter.post.list({ page: 1, page_size: 10 }).then((response) => { setPosts(response.data.data); }); }, []); return ( <div> <h1>Welcome to my ButterCMS-powered NEXT.js site!</h1> <ul> {posts.map((post) => ( <li key={post.slug}>{post.title}</li> ))} </ul> </div> ); } export default Home;
This code snippet demonstrates how to fetch and display ButterCMS content in your NEXT.js application using the ButterCMS JavaScript SDK.
8. Choosing the Right CMS for Your NEXT.js Project
Selecting the right CMS for your NEXT.js project depends on your specific needs and priorities. Here’s a summary to help you make an informed decision:
Choose WordPress If:
- You need extensive customization options.
- You plan to build a complex website with various features.
- You prioritize a vast ecosystem of themes and plugins.
Choose Ghost If:
- You want a clean, minimalist interface.
- Your focus is primarily on content and blogging.
- Speed and security are top priorities.
Choose ButterCMS If:
- You prefer a headless CMS for flexibility.
- Ease of use is crucial for both developers and content creators.
- You need a reliable CDN for fast content delivery.
Remember that no CMS is a one-size-fits-all solution, so evaluate your project’s requirements carefully. Additionally, consider factors like budget, scalability, and the technical expertise of your team.
Conclusion
Choosing the right CMS for your NEXT.js project is a critical decision that can impact your website’s performance, functionality, and ease of maintenance. WordPress, Ghost, and ButterCMS each have their strengths and weaknesses, making them suitable for different types of projects.
WordPress offers a vast ecosystem but may require optimization and security efforts as your website grows. Ghost provides a minimalistic, content-focused experience with excellent performance but has a more niche use case. ButterCMS, as a headless CMS, offers flexibility and ease of use, making it a strong contender for various projects.
Ultimately, the choice depends on your project’s specific needs and your team’s expertise. Carefully evaluate the pros and cons of each CMS to make an informed decision that aligns with your NEXT.js development goals.
With this guide, you’re now equipped to explore these CMS options and integrate them seamlessly into your NEXT.js application, ensuring a robust and dynamic web presence. Happy coding!
Table of Contents
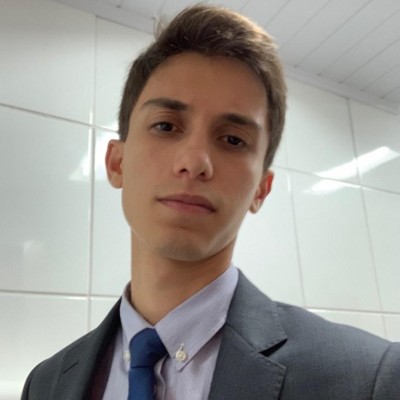
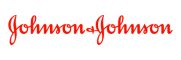