What is async/await in Node.js?
Async/await is a modern JavaScript feature introduced in ECMAScript 2017 (ES8) and widely supported in Node.js for handling asynchronous code in a synchronous-like manner. It provides a more readable and intuitive syntax for writing asynchronous code compared to traditional callback-based or Promise-based approaches.
Async/await allows you to write asynchronous code using a synchronous-looking syntax, making it easier to understand and maintain. It consists of two keywords:
- async: The async keyword is used to define asynchronous functions. Functions declared with the async keyword always return a Promise, even if they don’t explicitly return one.
- await: The await keyword is used inside async functions to pause the execution of the function until a Promise is settled (fulfilled or rejected). It allows you to wait for the result of an asynchronous operation before proceeding with the execution of the code.
Here’s an example of async/await syntax in Node.js:
javascript Copy code async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error:', error); } }
In this example, the fetchData() function is declared as an asynchronous function using the async keyword. Inside the function, the await keyword is used to wait for the response of the fetch() function and the conversion of the response to JSON format. The try-catch block is used to handle any errors that occur during the execution of the asynchronous operations.
Async/await simplifies the writing of asynchronous code in Node.js, making it more readable, maintainable, and error-resistant compared to traditional approaches.
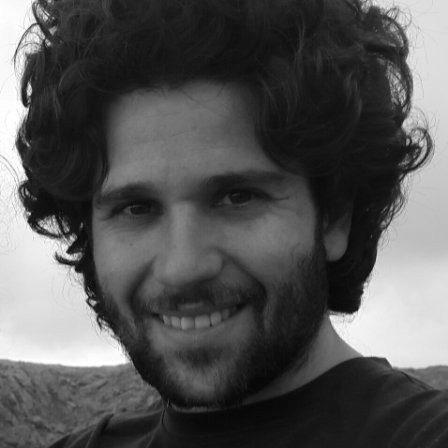
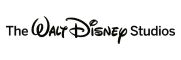