Mastering Asynchronous Control Flow in Node.js
In the world of web development, creating responsive and efficient applications is crucial. Node.js, a runtime environment built on Chrome’s V8 JavaScript engine, allows developers to write server-side applications using JavaScript. Asynchronous programming is a fundamental aspect of Node.js that enables developers to handle multiple tasks simultaneously without blocking the execution of other operations. Mastering asynchronous control flow in Node.js is essential for building high-performance applications that can handle a large number of concurrent operations efficiently.
Table of Contents
1. Understanding Asynchronous Programming
1.1. What is Asynchronous Programming?
At its core, asynchronous programming is a programming paradigm that allows tasks to be executed independently of the main program flow. In a synchronous program, tasks are executed one after the other, blocking the execution until each task is complete. In contrast, asynchronous programming enables tasks to be initiated and continue running in the background while the main program flow continues executing. This approach is particularly useful for tasks that involve waiting for external resources like file I/O, network requests, or database queries.
2. The Event Loop
Node.js uses an event-driven, non-blocking I/O model that leverages the concept of an event loop. The event loop is a central component of Node.js that allows it to efficiently handle asynchronous operations. Here’s how it works:
- Event Loop Initialization: When a Node.js application starts, the event loop is initialized. It continuously monitors the execution stack and the callback queue for tasks to execute.
- Executing Tasks: Asynchronous tasks, such as reading a file or making a network request, are initiated and placed in the background. The event loop continues to monitor the stack and the queue.
- Callback Queue: When an asynchronous task completes, its callback function is placed in the callback queue.
- Event Loop Iteration: During each iteration of the event loop, it checks the callback queue for completed tasks. If there are any callbacks, they are executed one by one.
- Non-Blocking: Asynchronous operations do not block the execution of the main program flow. This allows the application to remain responsive and handle multiple tasks concurrently.
Understanding the event loop is crucial for mastering asynchronous control flow in Node.js. Let’s delve deeper into some techniques and tools that can help you effectively manage asynchronous operations.
3. Promises: Handling Asynchronous Operations
Promises are a powerful abstraction for handling asynchronous operations in a more structured and readable way. A promise represents a value that may not be available yet but will be resolved in the future. It simplifies error handling and allows you to chain multiple asynchronous operations together.
3.1. Creating Promises
In Node.js, you can create a new promise using the Promise constructor. The promise constructor takes a single argument: a function that receives two parameters, resolve and reject. These parameters are functions themselves, which you call to indicate the success or failure of the promise.
javascript const fetchData = new Promise((resolve, reject) => { // Simulate fetching data asynchronously setTimeout(() => { const data = { message: "Data fetched successfully" }; resolve(data); // Resolve the promise with the data }, 1000); }); fetchData.then((data) => { console.log(data.message); }).catch((error) => { console.error("Error fetching data:", error); });
In this example, the fetchData promise simulates fetching data asynchronously and resolves with the fetched data after a delay of 1000 milliseconds.
3.2. Chaining Promises
One of the advantages of promises is their ability to chain multiple asynchronous operations together in a readable manner. This is achieved using the .then() method, which returns a new promise representing the result of the asynchronous operation.
javascript const fetchUserData = () => { return new Promise((resolve, reject) => { // Simulate fetching user data asynchronously setTimeout(() => { const userData = { username: "john_doe", role: "user" }; resolve(userData); }, 1500); }); }; const fetchUserProfile = (userData) => { return new Promise((resolve, reject) => { // Simulate fetching user profile asynchronously setTimeout(() => { const userProfile = { ...userData, email: "john@example.com" }; resolve(userProfile); }, 1000); }); }; fetchUserData() .then(fetchUserProfile) .then((userProfile) => { console.log("User Profile:", userProfile); }) .catch((error) => { console.error("Error:", error); });
In this example, the fetchUserData promise is chained with the fetchUserProfile promise to fetch and combine user data and profile information.
4. Async/Await: A Syntactic Bliss
While promises provide a significant improvement over traditional callback-based asynchronous code, async/await takes it a step further by offering a more synchronous-looking syntax. Async/await is built on top of promises and allows you to write asynchronous code in a style that closely resembles synchronous code.
4.1. Using Async/Await
To use async/await, you declare a function as async, which allows you to use the await keyword within it to pause the execution of the function until a promise is resolved. This makes the code look like it’s executing synchronously while still maintaining the benefits of asynchronous programming.
javascript const fetchUserDetails = async () => { try { const userData = await fetchUserData(); const userProfile = await fetchUserProfile(userData); console.log("User Profile:", userProfile); } catch (error) { console.error("Error:", error); } }; fetchUserDetails();
In this example, the fetchUserDetails function uses async and await to fetch and display the user profile details in a more readable and structured manner.
5. Handling Multiple Promises
In many scenarios, you might need to execute multiple asynchronous operations concurrently and wait for all of them to complete. Promise utilities like Promise.all() and Promise.race() are incredibly helpful in such cases.
5.1. Promise.all()
Promise.all() takes an array of promises and returns a new promise that resolves with an array of resolved values from the input promises. The resulting promise will only resolve if all input promises resolve successfully. If any of the promises reject, the resulting promise will be rejected.
javascript const fetchMultipleData = async () => { const [userData, weatherData] = await Promise.all([ fetchUserData(), fetchWeatherData(), ]); console.log("User Data:", userData); console.log("Weather Data:", weatherData); }; fetchMultipleData();
In this example, the fetchMultipleData function fetches user data and weather data concurrently using Promise.all().
5.2. Promise.race()
Promise.race() is used when you want to execute multiple promises concurrently but only care about the result of the first one that resolves or rejects. The resulting promise resolves or rejects as soon as any of the input promises do.
javascript const fetchFastestData = async () => { try { const result = await Promise.race([ fetchUserData(), fetchCachedData(), // Fetch data from cache ]); console.log("Fastest Data:", result); } catch (error) { console.error("Error:", error); } }; fetchFastestData();
In this example, the fetchFastestData function fetches user data and cached data concurrently using Promise.race(). It will use whichever promise completes first.
Conclusion
Mastering asynchronous control flow in Node.js is essential for building responsive and efficient applications. Promises and async/await are powerful tools that help you handle asynchronous operations in a structured and readable manner. Understanding the event loop and utilizing tools like Promise.all() and Promise.race() enables you to manage multiple asynchronous tasks effectively.
By embracing these techniques and concepts, you’ll be well-equipped to create Node.js applications that can handle a large number of concurrent operations without sacrificing performance or responsiveness. As you continue your journey in Node.js development, remember that asynchronous programming is a skill that takes time and practice to perfect, but the rewards are well worth the effort. Happy coding!
Table of Contents
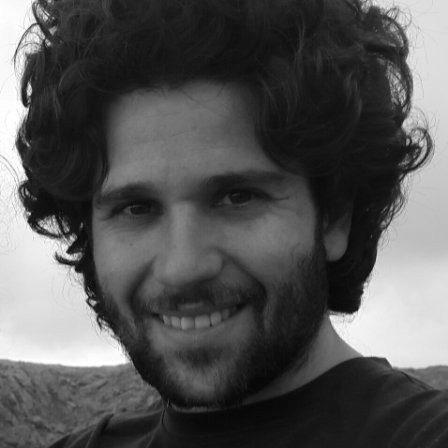
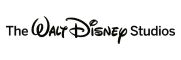