Mastering Asynchronous Programming in Node.js: From Callbacks to Async/Await
Node.js is renowned for its high performance and scalability, qualities that draw businesses to hire Node.js developers. Much of this performance can be attributed to the platform’s non-blocking, asynchronous nature. However, truly mastering asynchronous programming in Node.js, a fundamental skill for Node.js developers, involves comprehending a variety of techniques and features, from callback functions to Promises, Async/Await, and event-driven programming.
In this blog post, we will delve into the essentials of asynchronous programming in Node.js, providing clear examples to illustrate these concepts in action. These insights are not only invaluable for those looking to hire Node.js developers but also for developers aiming to refine their skill set in this widely-used runtime environment.
Understanding Synchronous vs Asynchronous Programming
Before we dive into the Node.js specific details, it’s important to grasp the fundamental difference between synchronous and asynchronous programming.
In synchronous programming, each operation blocks the execution of the subsequent operations until it completes. That is, the code executes sequentially, one operation at a time.
In contrast, asynchronous programming allows multiple operations to occur simultaneously. If an operation is time-consuming (like reading a file or making a network request), the program doesn’t wait for it to finish before moving onto the next operation. Instead, it proceeds and allows the time-consuming task to complete in the background.
Node.js is built around this asynchronous, event-driven model, which is why it’s excellent for IO-bound applications such as web servers, real-time applications, and data-intensive real-time applications (DIRT).
Callbacks
The most basic technique for asynchronous programming in Node.js is the callback function. This function is passed as an argument to another function and is invoked when the operation completes.
```javascript const fs = require('fs'); fs.readFile('/path/to/file', 'utf8', function(err, data) { if (err) { console.log(`Error: ${err}`); } else { console.log(data); } }); ```
In the code above, we use the `readFile` function from the Node.js `fs` module, which reads a file asynchronously. The callback function is the third argument, and it’s called when the file has been read.
While callbacks are simple and straightforward, they can quickly become unwieldy when handling complex asynchronous operations, leading to a problem known as callback hell, where code becomes deeply nested and hard to read.
Promises
Promises in Node.js are an improvement over callbacks, providing a more manageable structure for handling asynchronous operations. A Promise represents a value that may not be available yet but will be resolved in the future, or it may never get resolved.
```javascript const fs = require('fs').promises; fs.readFile('/path/to/file', 'utf8') .then(data => { console.log(data); }) .catch(err => { console.log(`Error: ${err}`); }); ```
In the example above, `readFile` returns a Promise that resolves with the file’s content. We can use `then` to handle the resolved value and `catch` to handle any errors. This approach avoids deep nesting and makes error handling more straightforward.
Async/Await
Async/Await is a modern approach to handle asynchronous operations in JavaScript, and by extension, Node.js. It allows us to write asynchronous code in a more synchronous-like fashion, which improves code readability and makes it easier to understand.
```javascript const fs = require('fs').promises; async function readFileAsync() { try { const data = await fs.readFile('/path/to/file', 'utf8'); console.log(data); } catch (err) { console.log(`Error: ${err}`); } } readFileAsync(); ```
In this code, we declare an asynchronous function using the `async` keyword, and inside this function, we can use the `await` keyword to pause the execution until the Promise resolves or rejects. Any error can be caught using a traditional try/catch block.
Event-Driven Programming
Another important aspect of asynchronous programming in Node.js is the event-driven model. Node.js provides the `EventEmitter` class, which is used to raise and handle custom events.
```javascript const EventEmitter = require('events'); class MyEmitter extends EventEmitter {} const myEmitter = new MyEmitter(); myEmitter.on('event', () => { console.log('An event occurred!'); }); myEmitter.emit('event'); ```
In this example, we create a custom event emitter `myEmitter` by extending the built-in `EventEmitter` class. We then use the `on` method to set up a listener for our custom event, and `emit` to trigger the event.
Conclusion
Mastering the art of asynchronous programming in Node.js is a key skill that businesses consider when they hire Node.js developers. By understanding and effectively using callbacks, Promises, Async/Await, and event-driven programming, developers can write code that is not only performant but also easy to understand and maintain. This level of proficiency is highly sought-after by companies looking to hire Node.js developers.
Asynchronous programming might seem a bit daunting initially, but with practice, it becomes second nature. Hence, for developers aspiring to be in high demand in the job market, the key to mastering Node.js and its asynchronous nature lies in hands-on experience and constant practice. And for businesses, understanding these concepts will assist you in making informed decisions when you plan to hire Node.js developers. Happy coding!
Table of Contents
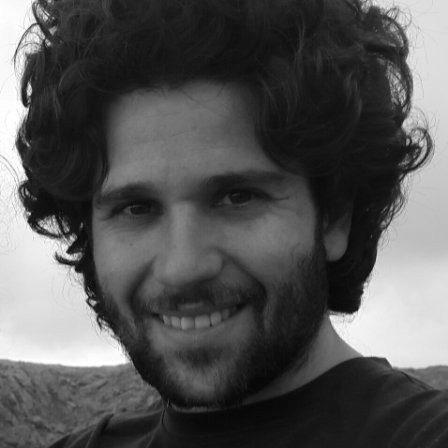
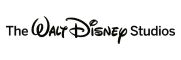