Authentication and Authorization in Node.js Applications
In today’s digital landscape, security is paramount when it comes to web applications. With the increasing amount of sensitive data being exchanged over the internet, ensuring that only authorized users can access and manipulate this data is of utmost importance. This is where authentication and authorization come into play. In this guide, we’ll delve into the concepts of authentication and authorization in the context of Node.js applications. We’ll explore their differences, delve into implementation details, and provide you with practical code samples to get you started on the path to building more secure applications.
Table of Contents
1. Understanding Authentication and Authorization
Before we dive into the technical aspects, let’s clarify the difference between authentication and authorization.
1.1. Authentication: Verifying User Identity
Authentication is the process of confirming the identity of a user, ensuring that they are who they claim to be. In a Node.js application, this involves collecting user credentials, such as a username and password, and verifying them against stored records. Proper authentication prevents unauthorized users from gaining access to the application. Common authentication methods include:
- Username and Password: This is the most traditional method. Users enter their username and password, which are then compared to a stored database of credentials.
- Token-based Authentication: Tokens, such as JSON Web Tokens (JWT), provide a secure way to authenticate users without storing their credentials. After the initial login, the server issues a token that is sent with each subsequent request to identify the user.
- OAuth: Often used for third-party authentication, OAuth allows users to log in using their existing credentials from platforms like Google or Facebook. It eliminates the need to create new credentials for every application.
1.2. Authorization: Controlling Access
Authorization comes into play after a user’s identity is verified. It involves determining what actions a user is allowed to perform within the application. For instance, an authenticated user might have permission to view certain resources but not modify them. Authorization ensures that users only have access to the parts of the application that they are authorized to use.
3. Implementing Authentication and Authorization in Node.js
Now that we have a clear understanding of these concepts, let’s explore how to implement them in Node.js applications.
3.1. Setting Up a Node.js Project
Before we proceed, make sure you have Node.js installed on your system. If not, you can download it from the official Node.js website. Once you have Node.js installed, follow these steps to set up a basic Node.js project:
3.2. Implementing Authentication with Passport.js
Passport.js is a popular authentication middleware for Node.js that provides a flexible and modular approach to authentication. It supports various authentication strategies, including username and password, token-based authentication, and OAuth. Let’s see how to implement local authentication (username and password) using Passport.js.
3.3. Implementing Token-Based Authentication with JWT
Token-based authentication, using JSON Web Tokens (JWT), is a powerful method to secure APIs and applications. JWTs are compact, URL-safe tokens that can carry information about the user and their permissions. Here’s how you can implement token-based authentication using JWT in your Node.js application:
3.4. Integrating OAuth for Third-Party Authentication
Enabling users to log in using their existing credentials from platforms like Google, Facebook, or GitHub can enhance user experience and save them from creating yet another set of login credentials. OAuth provides a secure way to implement this feature. Here’s a high-level overview of how to integrate OAuth using Passport.js:
3.5. Implementing Role-Based Authorization
Role-based authorization involves assigning different roles to users and granting access based on those roles. For instance, an application might have roles like “user,” “admin,” and “moderator,” each with different levels of access. Here’s how you can implement role-based authorization in your Node.js application:
3.6. Best Practices for Security
While implementing authentication and authorization, it’s crucial to follow security best practices to ensure the highest level of protection for your application and its users. Here are some tips to keep in mind:
Conclusion
In this guide, we’ve explored the fundamental concepts of authentication and authorization in the context of Node.js applications. We’ve seen how to implement various authentication methods using Passport.js, how to set up token-based authentication with JWT, and how to integrate OAuth for third-party authentication. Additionally, we’ve covered role-based authorization and highlighted security best practices.
By implementing robust authentication and authorization mechanisms, you can significantly enhance the security of your Node.js applications. Remember that security is an ongoing process, and it’s essential to stay updated with the latest security practices and vulnerabilities to keep your applications safe. With the knowledge and code samples provided in this guide, you’re well-equipped to build secure and user-friendly Node.js applications that protect both your data and your users’ privacy.
Table of Contents
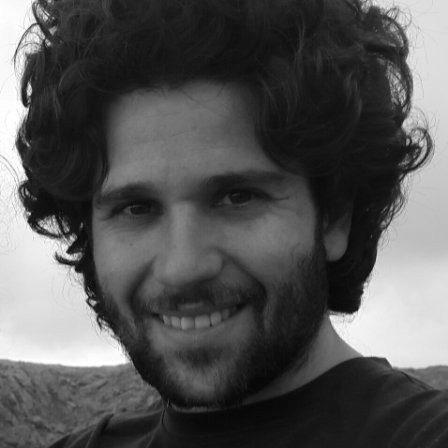
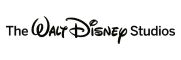