Exploring Serverless Architectures with Node.js and AWS Lambda
Serverless architectures are transforming the way applications are built and deployed by allowing developers to focus on writing code without managing server infrastructure. Node.js, with its non-blocking I/O and asynchronous capabilities, is an excellent choice for serverless computing. AWS Lambda, a leading serverless platform, enables you to run code in response to events without provisioning or managing servers. This article explores how to utilize Node.js and AWS Lambda for building efficient and scalable serverless solutions, with practical examples and best practices.
Understanding Serverless Architectures
Serverless computing allows developers to build and run applications without managing the underlying infrastructure. Instead of managing servers, you deploy code that runs in response to events and scales automatically. AWS Lambda is a key player in this ecosystem, enabling you to run backend functions in a serverless environment.
Using Node.js with AWS Lambda
Node.js is well-suited for serverless environments due to its lightweight runtime and asynchronous programming model. AWS Lambda provides native support for Node.js, allowing you to write and deploy functions with minimal setup.
1. Creating Your First Lambda Function with Node.js
To get started with AWS Lambda and Node.js, you need to create a simple function that responds to an event. Below is an example of a Lambda function that processes an incoming request and returns a response.
Example: A Simple AWS Lambda Function
```javascript exports.handler = async (event) => { console.log('Event:', event); return { statusCode: 200, body: JSON.stringify({ message: 'Hello from Lambda!' }), }; }; ```
To deploy this function, you can use the AWS Lambda console or the AWS CLI.
2. Integrating Lambda with API Gateway
AWS API Gateway allows you to expose your Lambda functions as HTTP endpoints, making it easy to build RESTful APIs. Here’s an example of how to set up an API Gateway to trigger your Lambda function.
Example: Configuring API Gateway
- Go to the API Gateway console.
- Create a new API.
- Add a new resource and method (e.g., GET).
- Configure the method to trigger your Lambda function.
- Deploy the API to a stage.
You can now access your Lambda function via the API Gateway endpoint.
3. Managing Lambda Function Dependencies
Node.js Lambda functions often require external libraries. AWS Lambda allows you to include dependencies by packaging them with your function code.
Example: Including Dependencies
- Create a `package.json` file with your dependencies.
```json { "name": "lambda-function", "version": "1.0.0", "dependencies": { "axios": "^0.21.1" } } ```
- Run `npm install` to install the dependencies.
- Package your function code and `node_modules` directory into a ZIP file.
- Upload the ZIP file to AWS Lambda.
4. Handling Asynchronous Operations
Node.js excels at handling asynchronous operations. AWS Lambda supports asynchronous invocations, allowing you to process events in the background.
Example: Asynchronous Lambda Function
```javascript exports.handler = async (event) => { // Simulate an asynchronous operation const result = await new Promise((resolve) => { setTimeout(() => { resolve('Data processed'); }, 2000); }); return { statusCode: 200, body: JSON.stringify({ message: result }), }; }; ```
5. Monitoring and Debugging Lambda Functions
AWS CloudWatch provides logging and monitoring capabilities for Lambda functions. You can use CloudWatch Logs to view logs and CloudWatch Metrics to monitor performance.
Example: Enabling CloudWatch Logging
- In the AWS Lambda console, go to the Monitoring tab.
- Enable CloudWatch Logs.
- Use `console.log` statements in your code to write logs.
Conclusion
Node.js and AWS Lambda offer a powerful combination for building serverless applications. By leveraging Node.js’s asynchronous capabilities and AWS Lambda’s scalability, you can create efficient and responsive serverless solutions. From creating basic Lambda functions to managing dependencies and integrating with other AWS services, Node.js and AWS Lambda provide a robust platform for modern application development.
Further Reading:
Table of Contents
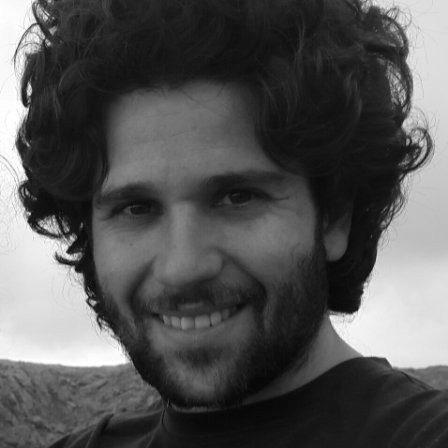
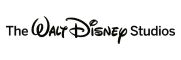