Node.js and Big Data: Processing and Analyzing Large Datasets
In the realm of big data, the ability to efficiently process and analyze massive datasets is paramount. Node.js, known for its scalability and non-blocking I/O model, has emerged as a powerful tool for handling such tasks. In this article, we’ll explore how Node.js can be leveraged for processing and analyzing large datasets, along with real-world examples showcasing its capabilities.
Processing Large Datasets with Node.js
One of the key advantages of Node.js in processing large datasets is its non-blocking I/O model. Instead of waiting for each I/O operation to complete before moving on to the next, Node.js employs callbacks or promises to execute code asynchronously. This enables Node.js applications to handle multiple concurrent operations efficiently, making it ideal for processing large datasets without experiencing performance bottlenecks.
Example 1: Reading and Parsing CSV Files
Consider a scenario where you need to analyze a massive CSV file containing millions of records. Using Node.js, you can utilize libraries like csv-parser or fast-csv to read and parse the file asynchronously, without blocking the event loop. This allows you to stream data from the file, process it in chunks, and perform analysis in real-time.
const fs = require('fs'); const csvParser = require('csv-parser'); fs.createReadStream('large_dataset.csv') .pipe(csvParser()) .on('data', (row) => { // Process each row asynchronously }) .on('end', () => { console.log('CSV file processed successfully'); });
Example 2: Distributed Computing with Node.js and Apache Spark
Node.js can also be integrated with distributed computing frameworks like Apache Spark to analyze large datasets in parallel. By leveraging libraries such as spark-node-client, you can distribute data processing tasks across multiple nodes, harnessing the power of a cluster for faster analysis.
Analyzing Big Data with Node.js
In addition to processing large datasets, Node.js can also be used for real-time data analysis and visualization. With the help of libraries like D3.js or Plotly, you can generate interactive visualizations to gain insights from your data dynamically.
Example 3: Real-Time Dashboard for Streaming Data
Imagine you’re monitoring real-time sensor data from IoT devices. With Node.js, you can build a web-based dashboard that continuously updates with live data streams. By utilizing technologies like WebSockets or server-sent events (SSE), you can push data from the server to the client in real-time, providing instant insights into changing trends or anomalies.
const express = require('express'); const http = require('http'); const socketIO = require('socket.io'); const app = express(); const server = http.createServer(app); const io = socketIO(server); // Emit real-time data to connected clients setInterval(() => { const sensorData = /* Get live sensor data */; io.emit('sensorData', sensorData); }, 1000);
Conclusion
Node.js offers a versatile platform for processing and analyzing large datasets, thanks to its asynchronous nature and scalability. Whether you’re dealing with massive CSV files, distributed computing tasks, or real-time data streams, Node.js provides the tools and libraries necessary to tackle big data challenges efficiently.
By harnessing the power of Node.js, developers can unlock new insights from their data and drive innovation in various domains, from e-commerce and finance to healthcare and IoT.
External Resources:
In this blog post, we’ve only scratched the surface of what Node.js can do in the realm of big data. As technology continues to evolve, Node.js is poised to remain a valuable tool for processing, analyzing, and deriving insights from large datasets.
Table of Contents
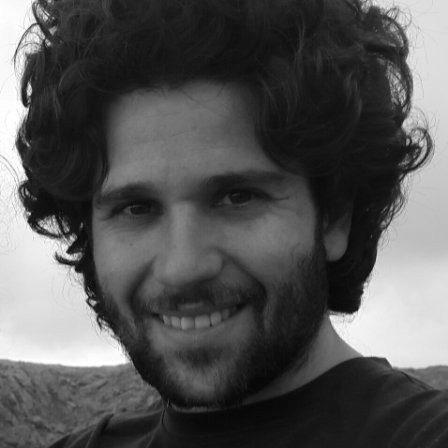
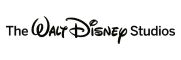