Building Blockchain Applications with Node.js and Ethereum
In the ever-evolving landscape of technology, blockchain has emerged as a revolutionary force, offering decentralized solutions with unparalleled security and transparency. Ethereum, a prominent blockchain platform, has empowered developers to build decentralized applications (DApps) using smart contracts. In this guide, we’ll explore how to leverage Node.js, a popular JavaScript runtime environment, to develop blockchain applications on the Ethereum network.
Understanding Ethereum and Smart Contracts
Before delving into the development process, let’s grasp the fundamentals. Ethereum is a decentralized platform that enables the creation of smart contracts – self-executing contracts with predefined rules and conditions. These contracts are deployed on the Ethereum Virtual Machine (EVM) and can automate complex processes without the need for intermediaries.
Setting Up the Development Environment
To start building blockchain applications with Ethereum and Node.js, you’ll need to set up your development environment. Begin by installing Node.js and npm (Node Package Manager) on your system. Then, you can use npm to install tools like Truffle and web3.js, essential for Ethereum development.
1. Truffle
Truffle is a development framework for Ethereum that simplifies the process of creating, testing, and deploying smart contracts. You can install Truffle globally using npm:
npm install -g truffle
2. web3.js
web3.js is a JavaScript library that allows interaction with Ethereum nodes, enabling developers to interact with smart contracts from their applications. Install web3.js using npm:
npm install web3
Creating Smart Contracts with Solidity
Solidity is the programming language used for writing smart contracts on the Ethereum platform. Let’s create a simple smart contract that acts as a decentralized ledger:
// SimpleStorage.sol pragma solidity ^0.8.0; contract SimpleStorage { uint256 public data; function setData(uint256 _data) public { data = _data; } function getData() public view returns (uint256) { return data; } }
Save the above code in a file named SimpleStorage.sol.
Compiling and Deploying Smart Contracts
Once you’ve written your smart contract, you’ll need to compile it using Truffle. Navigate to your project directory and run the following commands:
truffle compile
Next, deploy the smart contract to the Ethereum network. You can use tools like Ganache for local development or connect to a test network like Rinkeby or Ropsten.
Interacting with Smart Contracts Using Node.js
With the smart contract deployed, let’s build a Node.js application to interact with it. First, create a JavaScript file (e.g., app.js) and import the necessary dependencies:
// app.js const Web3 = require('web3'); const contractAbi = require('./build/contracts/SimpleStorage.json').abi; // Connect to a local Ethereum node const web3 = new Web3('http://localhost:8545'); // Instantiate the contract const contractAddress = 'CONTRACT_ADDRESS'; const contract = new web3.eth.Contract(contractAbi, contractAddress); // Example: Set and get data async function setData() { await contract.methods.setData(42).send({ from: 'YOUR_ADDRESS' }); } async function getData() { const data = await contract.methods.getData().call(); console.log('Data:', data); } // Call the functions setData(); getData();
Replace ‘CONTRACT_ADDRESS’ with the address of your deployed smart contract and ‘YOUR_ADDRESS’ with your Ethereum address.
Conclusion
Building blockchain applications with Node.js and Ethereum opens up a world of possibilities for developers. From decentralized finance (DeFi) platforms to non-fungible tokens (NFTs), the potential applications are limitless. By leveraging the power of smart contracts and the Ethereum network, developers can create secure, transparent, and immutable solutions that revolutionize industries.
External Links:
Happy coding, and may your blockchain endeavors pave the way for a decentralized future!
Table of Contents
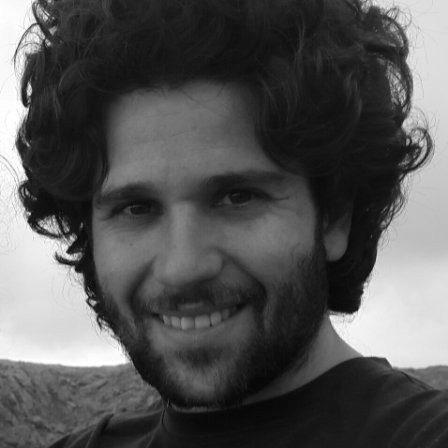
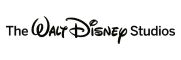