Architecting for Growth: Strategies for Building Scalable Web Applications with Node.js
Web development has significantly transformed over the past decade, largely due to JavaScript and Node.js. Node.js has emerged as a top choice for developers, including those you might hire as Node.js developers, to create scalable, efficient, and reliable web applications. This server-side runtime environment, renowned for its asynchronous, event-driven architecture, and non-blocking I/O, provides the foundation for robust web applications.
In this blog post, we delve into the process of building scalable web applications using Node.js. We will explore the key concepts, best practices, and illustrative examples, providing a comprehensive guide for those looking to hire Node.js developers or anyone interested in harnessing the power of Node.js.
Why Choose Node.js for Scalability?
Before we dive in, let’s briefly discuss why Node.js is an excellent choice for scalable applications.
- Non-Blocking I/O: Unlike traditional web servers which create a new thread for every new request, Node.js works on a single thread. It uses an event loop and callback functions to handle multiple concurrent clients, reducing system resource usage and allowing for a higher level of scalability.
- Microservice Architecture: Node.js is well-suited for the microservice architecture – a design approach where an application is a collection of small, independent services that communicate over the network. This architectural style allows for better scalability and easier deployment and maintenance.
- NPM (Node Package Manager): With over a million packages available, Node.js developers have access to numerous tools to improve scalability, such as load balancers, HTTP request routers, and task schedulers.
Scalability Concepts
Scalability is the ability of a system, network, or process to handle a growing amount of work in a capable manner or its potential to be enlarged to accommodate that growth. There are two main types of scalability:
– Vertical Scaling: Also known as “scaling up,” it involves adding more resources (like CPU, RAM) to your server to handle increased load.
– Horizontal Scaling: Also known as “scaling out,” it involves adding more machines to your server pool to handle increased load.
Node.js is particularly good at horizontal scaling, and it can also be combined with vertical scaling to provide a highly scalable solution.
Building Scalable Web Applications
To demonstrate how to build a scalable web application using Node.js, we will use the example of a hypothetical social media application.
1. Implementing a Load Balancer
In a high-traffic application, a single Node.js server instance may not be able to handle all the requests efficiently. This is where horizontal scaling comes into play, and we can achieve this by adding more server instances and implementing a load balancer.
```javascript const httpProxy = require('http-proxy'); const http = require('http'); const addresses = [ { target: 'http://localhost:8001' }, { target: 'http://localhost:8002' }, ]; const proxy = httpProxy.createProxyServer(); http.createServer((req, res) => { const target = addresses[Math.floor(Math.random() * addresses.length)]; proxy.web(req, res, target); }).listen(8000); ```
In this example, we use the `http-proxy` module to create a load balancer that listens on port 8000 and forwards the requests to either port 8001 or 8002, which could be different instances of your application.
2. Using the Cluster Module
Another way to achieve horizontal scaling is by using the built-in Cluster module in Node.js. This module allows you to create child processes (workers) that share server ports, which can improve the performance of your application.
```javascript const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); } } else { http.createServer((req, res) => { res.writeHead(200); res.end(`Process ${process.pid} says hello!`); }).listen(8000); } ```
In this example, we use the `os` module to get the number of CPU cores and create a worker for each core. Each worker can then handle requests independently of the others.
3. Applying Caching
Caching is a technique that stores data in a temporary storage area so that future requests for that data can be served faster. In our hypothetical social media application, we can cache frequently accessed data such as user profiles or posts.
```javascript const express = require('express'); const NodeCache = require( "node-cache" ); const myCache = new NodeCache(); const app = express(); app.get('/user/:id', (req, res) => { const userId = req.params.id; const user = myCache.get(userId); if (user) { res.send(user); } else { // Fetch user from database, then store in cache const fetchedUser = getUserFromDatabase(userId); myCache.set(userId, fetchedUser); res.send(fetchedUser); } }); app.listen(8000); ```
In this example, we use the `node-cache` module to implement caching. When we receive a request for a user profile, we first check if the profile is in the cache. If it is, we return the cached profile; otherwise, we fetch the profile from the database, store it in the cache, and then return it.
4. Implementing Microservices
As your application grows, it can become difficult to manage if it’s all in one monolithic structure. Breaking it up into microservices can make it easier to manage and scale.
In the context of our social media application, we could break it up into multiple services like the User Service, the Post Service, and the Comment Service. Each of these would be a separate Node.js application that can be scaled independently of the others.
```javascript // Post service const express = require('express'); const app = express(); app.get('/posts', (req, res) => { // Fetch and return posts }); app.post('/posts', (req, res) => { // Create a new post }); app.listen(8001); // User service const express = require('express'); const app = express(); app.get('/users', (req, res) => { // Fetch and return users }); app.post('/users', (req, res) => { // Create a new user }); app.listen(8002); ```
In these examples, we have a separate application for the User Service and the Post Service. They run on different ports and can be developed, deployed, and scaled independently.
Conclusion
Node.js is a dynamic platform, ideal for building scalable web applications. Its versatility and robustness, along with continuous evolution, make it a popular choice for many major companies such as Netflix, LinkedIn, and Uber. When scaling applications, it’s not just about handling increased traffic but also about designing an architecture that allows flexibility and efficient management. If you’re looking to build high-performance applications that can cater to growing demands, hiring Node.js developers could be a valuable decision. They can help you to leverage Node.js features effectively, understand your application’s performance characteristics, and ensure your scalability improvements work as intended. With their expertise, your application will be on the right path to success.
Table of Contents
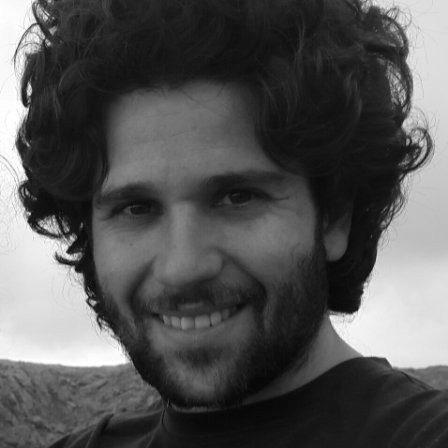
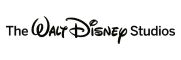