Building Web Scrapers with Cheerio and Node.js
In the dynamic landscape of the internet, data reigns supreme. From market research to competitive analysis, access to accurate and timely data can make or break a business. However, gathering data from the web can be a daunting task, especially when faced with the ever-changing structure of websites. Enter web scraping – a technique used to extract data from websites automatically. In this post, we’ll delve into the world of web scraping and learn how to harness its potential by building web scrapers with Cheerio and Node.js.
Understanding Web Scraping
Web scraping involves fetching and extracting data from websites. It allows you to automate the process of gathering information, saving you time and effort. Whether you’re collecting pricing data, monitoring market trends, or conducting sentiment analysis, web scraping can be a valuable tool in your arsenal.
Introducing Cheerio and Node.js
Cheerio is a fast, flexible, and lightweight library for parsing and manipulating HTML in Node.js. Leveraging the power of jQuery, Cheerio provides a familiar syntax for traversing and manipulating the DOM, making it an ideal choice for web scraping projects. Combined with the asynchronous nature of Node.js, Cheerio enables you to build efficient and scalable web scrapers.
Getting Started
To begin building web scrapers with Cheerio and Node.js, you’ll need to have Node.js installed on your machine. Once Node.js is set up, you can install Cheerio using npm:
npm install cheerio
Building a Simple Web Scraper
Let’s dive into a simple example to demonstrate how to use Cheerio for web scraping. Suppose we want to extract the headlines from a news website. Here’s how we can achieve that:
const axios = require('axios'); const cheerio = require('cheerio'); axios.get('https://example.com/news') .then(response => { const $ = cheerio.load(response.data); $('h2.headline').each((index, element) => { console.log($(element).text()); }); }) .catch(error => { console.log(error); });
In this example, we use Axios to make an HTTP request to the news website and Cheerio to parse the HTML response. We then use Cheerio’s each method to iterate over the headlines and extract their text.
Advanced Techniques
Web scraping is a vast field with numerous techniques and strategies. Here are a few advanced techniques you can explore to enhance your web scraping skills:
- Handling Dynamic Content: Many modern websites use JavaScript to dynamically load content. To scrape such websites, you may need to use tools like Puppeteer to render the page before extracting data.
- Rate Limiting and Throttling: When scraping data from websites, it’s essential to be respectful of their servers’ resources. Implementing rate limiting and throttling mechanisms can help prevent your scraper from being blocked.
- Data Parsing and Cleaning: Once you’ve extracted data from a website, you may need to parse and clean it before using it for analysis or storage. Tools like Regular Expressions (RegEx) and NLP libraries can be helpful in this regard.
Conclusion
Web scraping opens up a world of opportunities for gathering valuable data from the web. By leveraging tools like Cheerio and Node.js, you can build powerful web scrapers capable of extracting and processing data from a variety of sources. Whether you’re a business analyst, researcher, or developer, mastering the art of web scraping can provide you with a competitive edge in today’s data-driven world.
Now that you’ve learned the basics of building web scrapers with Cheerio and Node.js, it’s time to roll up your sleeves and start scraping! Happy scraping!
External Resources:
Remember, while web scraping can be a powerful tool, it’s essential to scrape responsibly and ethically. Always adhere to a website’s terms of service and respect their robots.txt file to avoid legal issues. Happy scraping!
Table of Contents
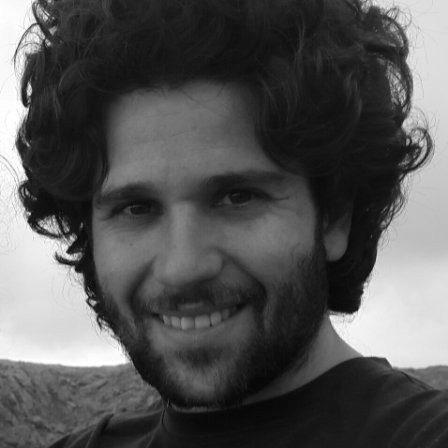
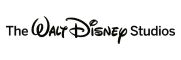