Caching Strategies in Node.js for Improved Performance
In the realm of web development, performance is paramount. Users today expect lightning-fast responses from their applications, and any delays can lead to frustration and abandonment. One powerful technique to optimize the performance of your Node.js applications is caching. Caching involves storing frequently accessed data in memory so that it can be quickly retrieved, reducing the need to fetch the same data repeatedly from the source. In this article, we’ll dive into various caching strategies in Node.js that can significantly enhance your application’s responsiveness.
Table of Contents
1. Why Caching Matters
Before we delve into the nitty-gritty of caching strategies, let’s understand why caching matters. In a Node.js application, data retrieval can often be a time-consuming process, involving database queries, API calls, or other external requests. Caching helps mitigate this overhead by storing copies of frequently used data in memory, reducing the need to repeatedly fetch the same data from its original source.
Caching not only improves response times but also reduces the load on your application’s resources. By serving cached data, your application can handle a higher number of requests without putting unnecessary strain on your servers or databases. This is particularly crucial in scenarios where the same data is requested by multiple users simultaneously.
2. Types of Caching Strategies
2.1. In-Memory Caching
In-memory caching is one of the most straightforward and effective caching strategies. It involves storing data in the application’s memory, typically using key-value pairs. This data can be quickly accessed and doesn’t require any external services. One popular library for in-memory caching in Node.js is Node-cache.
Here’s a basic example of how you can use Node-cache for in-memory caching:
javascript const NodeCache = require('node-cache'); const cache = new NodeCache(); // Store data in the cache cache.set('user:123', { name: 'Alice', age: 30 }, 3600); // Expires in 1 hour // Retrieve data from the cache const userData = cache.get('user:123'); if (userData) { console.log('User data:', userData); } else { console.log('Data not found in cache.'); }
2.2. Distributed Caching
While in-memory caching is effective for a single server, it falls short in a distributed environment or when you’re dealing with multiple instances of your application. Distributed caching systems like Redis and Memcached come to the rescue in such scenarios. They provide a centralized location to store cached data, accessible by all instances of your application.
Here’s an example of using Redis for distributed caching in Node.js:
javascript const redis = require('redis'); const client = redis.createClient(); // Store data in Redis cache client.set('product:456', JSON.stringify({ name: 'Widget', price: 19.99 })); // Retrieve and parse data from Redis cache client.get('product:456', (err, data) => { if (err) { console.error('Error retrieving data from cache:', err); } else if (data) { const productData = JSON.parse(data); console.log('Product data:', productData); } else { console.log('Data not found in cache.'); } });
2.3. Time-Based Expiration
One essential aspect of caching is managing the freshness of cached data. Data can become stale over time, leading to inaccurate or outdated information being served to users. Time-based expiration is a caching strategy that automatically removes data from the cache after a specified period.
javascript // Using Node-cache with time-based expiration cache.set('news:latest', { headlines: [...], timestamp: Date.now() }, 1800); // Expires in 30 minutes
2.4. Cache Invalidation
Cache invalidation involves removing or updating cached data when the underlying data changes. Stale data can lead to confusion and errors, so it’s crucial to invalidate the cache whenever relevant data is modified. This can be done manually or automatically, depending on the nature of your application.
javascript // Invalidating cache when a new post is created app.post('/create-post', (req, res) => { // Logic to create a new post cache.del('posts:latest'); // Invalidate the cache for latest posts res.send('Post created successfully.'); });
3. Choosing the Right Caching Strategy
The choice of caching strategy depends on various factors, including the nature of your application, the type of data you’re dealing with, and your infrastructure. Here are some tips to help you choose the right strategy:
- In-Memory Caching is excellent for small to medium-sized applications with a single server. It’s simple to implement and works well when your data doesn’t change frequently.
- Distributed Caching should be considered for larger applications with multiple instances. If your application needs to share cached data across instances or if you’re looking for high availability, Redis or Memcached can be valuable options.
- Time-Based Expiration is essential for ensuring that your cached data doesn’t become stale. Use this strategy when you have data that has a predictable expiration time.
- Cache Invalidation becomes crucial when your data is dynamic and subject to frequent changes. Implement mechanisms to automatically invalidate or update the cache when the underlying data changes.
4. Implementing Caching in Express.js
Express.js, a popular Node.js web framework, makes it relatively easy to implement caching strategies. Here’s an example of how you can integrate caching using Express.js middleware:
javascript const express = require('express'); const NodeCache = require('node-cache'); const app = express(); const cache = new NodeCache(); // Custom middleware for caching function cacheMiddleware(req, res, next) { const key = req.originalUrl || req.url; const cachedData = cache.get(key); if (cachedData) { res.send(cachedData); } else { res.sendResponse = res.send; res.send = (body) => { cache.set(key, body, 60); // Cache for 1 minute res.sendResponse(body); }; next(); } } app.get('/api/posts', cacheMiddleware, (req, res) => { // Logic to fetch and send posts data }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
In this example, the cacheMiddleware function checks if the requested URL is already cached. If cached data exists, it’s immediately sent as the response. If not, the middleware intercepts the response using res.send and caches the response before sending it to the client.
5. Monitoring and Evolving Your Caching Strategy
Implementing a caching strategy is not a “set it and forget it” process. It’s essential to monitor your application’s performance, cache hit rate, and other relevant metrics. Tools like New Relic, Datadog, and custom logging can help you gain insights into how well your caching strategy is working.
As your application evolves, your caching strategy might need adjustments. New features, changes in data patterns, or shifts in traffic can all impact the effectiveness of your caching. Regularly revisit and fine-tune your caching strategy to ensure it continues to provide optimal performance.
Conclusion
Caching strategies play a pivotal role in optimizing the performance of your Node.js applications. By strategically storing frequently used data, you can significantly reduce response times and enhance user experiences. Whether you opt for in-memory caching, distributed caching, or a combination of strategies, a well-implemented caching strategy can be the difference between a sluggish application and a lightning-fast one. Remember that the choice of strategy depends on your application’s unique requirements, so take the time to analyze and experiment to find the best approach for your use case.
Table of Contents
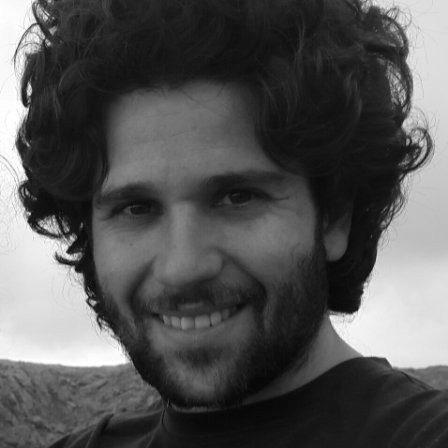
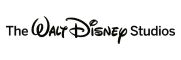