Developing Real-Time Chatbots with Node.js and Rasa
Understanding Real-Time Chatbots
Real-time chatbots are designed to engage users in real-time, providing instant responses to their queries. These chatbots are increasingly being used across various industries to enhance customer service, automate routine tasks, and provide personalized experiences. Leveraging Node.js for backend development and Rasa for natural language understanding (NLU) and dialogue management offers a powerful combination for building responsive and intelligent chatbots.
Why Node.js and Rasa?
- Node.js: Known for its non-blocking I/O and event-driven architecture, Node.js is ideal for developing real-time applications like chatbots. It allows for efficient handling of multiple connections and seamless integration with various services.
- Rasa: Rasa is an open-source machine learning framework for building contextual chatbots. It provides tools for intent classification, entity recognition, and dialogue management, enabling developers to create bots that understand and manage complex conversations.
1. Setting Up the Development Environment
Before we dive into building the chatbot, let’s set up the environment.
- Install Node.js: Ensure that Node.js is installed on your machine. You can download it from [Node.js official website](https://nodejs.org/).
- Install Rasa: Rasa can be installed using pip (Python’s package manager). Make sure Python is installed on your system, then run:
```bash pip install rasa ```
2. Building a Basic Node.js Server
The first step in developing the chatbot is setting up a Node.js server that will act as the backend for the bot.
```javascript const express = require('express'); const bodyParser = require('body-parser'); const app = express(); app.use(bodyParser.json()); app.post('/webhook', (req, res) => { const userMessage = req.body.message; // Process the message or send it to Rasa for NLU processing res.send({ reply: `Received: ${userMessage}` }); }); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
3. Integrating with Rasa for Natural Language Understanding
Next, we integrate the Node.js server with Rasa to leverage its NLU capabilities. This allows the bot to understand user intents and extract relevant information from the conversation.
```javascript const axios = require('axios'); app.post('/webhook', async (req, res) => { const userMessage = req.body.message; // Send message to Rasa for intent recognition const rasaResponse = await axios.post('http://localhost:5005/model/parse', { text: userMessage, }); const intent = rasaResponse.data.intent.name; const entities = rasaResponse.data.entities; res.send({ intent, entities }); }); ```
4. Managing Conversations with Rasa
To handle complex conversations, Rasa’s dialogue management capabilities come into play. Define your bot’s conversation logic using Rasa’s stories and actions.
- Define Intents and Entities: In `nlu.yml`, define the various intents and entities your bot should recognize.
```yaml - intent: greet examples: | - hello - hi - good morning ```
- Define Stories: In `stories.yml`, outline the conversation paths.
```yaml stories: - story: greet the user steps: - intent: greet - action: utter_greet ```
- Create Custom Actions: Implement custom actions in `actions.py` if your bot needs to perform specific tasks.
5. Real-Time Communication with Socket.IO
To enable real-time communication, integrate Socket.IO with your Node.js server. This will allow your chatbot to handle live interactions with users.
```javascript const http = require('http'); const socketIo = require('socket.io'); const server = http.createServer(app); const io = socketIo(server); io.on('connection', (socket) => { socket.on('user_message', async (message) => { const rasaResponse = await axios.post('http://localhost:5005/webhooks/rest/webhook', { sender: socket.id, message, }); const botReply = rasaResponse.data[0].text; socket.emit('bot_reply', botReply); }); }); server.listen(3000, () => { console.log('Real-time server running on port 3000'); }); ```
Testing and Deployment
Once the chatbot is developed, thoroughly test it across different scenarios. Ensure that the bot can handle multiple intents, maintain context, and provide relevant responses. For deployment, consider using cloud platforms like AWS, Google Cloud, or Azure, and containerize your application with Docker for scalability.
Conclusion
Node.js and Rasa provide a powerful framework for developing real-time chatbots that are both responsive and intelligent. By integrating the real-time capabilities of Node.js with the NLU and dialogue management features of Rasa, you can create chatbots that offer a seamless and engaging user experience. Whether you’re building a customer service bot or an interactive assistant, this combination will help you deliver a robust solution.
Further Reading:
Table of Contents
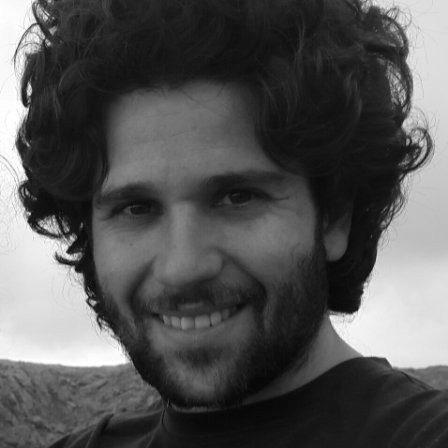
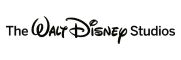