What is clustering in Node.js?
Clustering in Node.js refers to a technique for improving the performance and scalability of Node.js applications by utilizing multiple CPU cores. By spawning multiple instances of a Node.js application (workers) and distributing incoming connections among them, clustering allows applications to handle more concurrent requests and make better use of available hardware resources.
Node.js provides a built-in module called cluster that allows you to implement clustering in your applications easily. The cluster module allows you to create a cluster of worker processes, each running the same Node.js application code, and distribute incoming connections among them using a round-robin algorithm.
Here’s how you can implement clustering in a Node.js application using the cluster module:
javascript Copy code const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { // Fork worker processes equal to the number of CPU cores for (let i = 0; i < numCPUs; i++) { cluster.fork(); } // Handle worker process exit and restart if necessary cluster.on('exit', (worker, code, signal) => { console.log(`Worker ${worker.process.pid} died`); cluster.fork(); }); } else { // Worker process - create HTTP server and handle requests http.createServer((req, res) => { res.writeHead(200); res.end('Hello, World!'); }).listen(3000); console.log(`Worker ${process.pid} started`); }
In this example, the master process (identified by cluster.isMaster) creates a cluster of worker processes using cluster.fork(), one for each CPU core. Each worker process then listens for incoming HTTP requests and handles them independently. If a worker process dies for any reason, the master process detects the exit event and restarts the worker to maintain the desired number of worker processes.
By utilizing clustering, Node.js applications can effectively utilize multi-core CPUs, improve performance, and handle more concurrent connections, making them suitable for high-traffic and scalable applications.
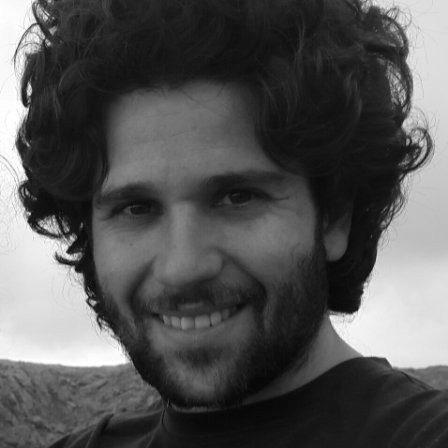
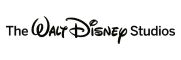