Node.js Decoded: A Deep Dive into Its Core Features and Functionalities
Over the last decade, Node.js has consistently maintained its place as one of the most popular environments for server-side web development. Its ability to handle asynchronous I/O with its event-driven architecture, along with its flexibility and compatibility with JavaScript, have made it a favorite amongst developers worldwide. This is also why businesses often look to hire Node.js developers to harness these capabilities for their projects.
In this blog post, we are going to dive deep into the core features of Node.js, demystifying the elements that have contributed to its popularity. Whether you’re a developer seeking to deepen your skills, or a business looking to hire Node.js developers to strengthen your team, this exploration will enhance your understanding of Node.js.
For each feature, we will examine how it works and provide practical examples for a hands-on understanding. With a comprehensive grasp of Node.js, developers can unleash its full potential, and businesses can make an informed decision when they hire Node.js developers. So, let’s get started on this enriching journey!
1. Asynchronous and Event-Driven Architecture
At the heart of Node.js lies its asynchronous and event-driven nature, which fundamentally distinguishes it from other server-side environments. Unlike traditional, synchronous programming environments where operations are executed sequentially, Node.js’s asynchronous nature means that I/O operations like reading files, making database calls, or handling network requests do not block the execution of other operations.
This asynchronous behavior is powered by Node.js’s event-driven architecture. When an operation is initiated, Node.js fires it off and then moves to the next task without waiting for the operation to complete. Upon completion, an event is triggered, which is then handled by an associated callback function.
Consider the following code that reads a file:
```javascript var fs = require('fs'); fs.readFile('sample.txt', 'utf8', function(err, contents) { console.log(contents); }); console.log('After calling readFile'); ```
In this example, `readFile` is an asynchronous operation. The message ‘After calling readFile’ will be logged before the contents of the file because Node.js does not wait for the file reading operation to complete before moving to the next operation.
2. Non-blocking I/O
The non-blocking I/O model is a feature closely related to the asynchronous, event-driven architecture of Node.js. In a blocking I/O model, operations are performed sequentially, which can result in significant performance issues when handling heavy traffic or dealing with large files or data.
In contrast, Node.js’s non-blocking I/O model allows it to handle multiple operations simultaneously, making it well-suited for applications that need to handle a high volume of simultaneous connections, such as chat servers, collaborative tools, or real-time analytics.
The following example demonstrates a server handling multiple requests:
```javascript var http = require('http'); http.createServer(function (req, res) { fs.readFile('bigfile.txt', function(err, data) { if (err) throw err; res.end(data); }); }).listen(8080); ```
In this example, even if the file is large and takes a long time to read, the server can still accept new connections, providing much better performance than a server built with a blocking I/O model.
3. Single-Threaded, Yet Scalable
While Node.js is single-threaded, leveraging JavaScript’s single-threaded event loop model, it’s a misconception that Node.js can’t handle concurrent operations. Due to its non-blocking I/O and event-driven architecture, Node.js can handle a high volume of requests without the need for creating new threads, which can be resource-intensive.
However, for CPU-intensive tasks, a single Node.js process might not be enough. To handle this, Node.js offers child processes and worker threads that allow you to offload heavy computation to separate threads, and clustering which allows running multiple instances of the application in parallel.
```javascript const cluster = require('cluster'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { console.log(`Master ${process.pid} is running`); for (let i = 0; i < numCPUs; i++) { cluster.fork(); } cluster.on('exit', (worker, code, signal) => { console.log(`worker ${worker.process.pid} died`); }); } else { // Workers can share any TCP connection // In this case, it is an HTTP server http.createServer((req, res) => { res.writeHead(200); res.end('hello world\n'); }).listen(8000); console.log(`Worker ${process.pid} started`); } ```
In this example, Node.js uses the cluster module to create a new worker process for each CPU core on the system, each capable of handling incoming connections.
4. Built on Chrome’s V8 JavaScript Engine
Node.js is built on top of Chrome’s V8 JavaScript engine, which compiles JavaScript directly into machine code, resulting in high performance. The V8 engine is continuously updated by Google, which ensures that Node.js users are able to take advantage of the latest JavaScript features.
Moreover, the fact that Node.js uses JavaScript, one of the most popular programming languages, reduces the barrier to entry, making it accessible to a broad base of developers.
5. Extensive Package Ecosystem – NPM
Node.js includes access to the Node Package Manager (NPM), an extensive ecosystem of open-source libraries. With over a million packages, NPM provides tools and modules that can help with virtually any project, reducing development time and complexity.
Installing a package from NPM is straightforward:
```javascript npm install express ```
In this example, the Express.js framework is installed, which can then be used to simplify the development of web applications.
Conclusion
Node.js’s core features – its asynchronous and event-driven architecture, non-blocking I/O, scalability despite being single-threaded, and its reliance on Chrome’s high-performance V8 engine – all contribute to its stature as a go-to environment for server-side web development. It’s no wonder that businesses are frequently looking to hire Node.js developers to leverage these benefits.
In addition to these, the platform boasts an extensive package ecosystem, NPM, further enhancing its appeal. With a vast range of tools and modules available, development time and complexity can be significantly reduced.
Understanding these core features empowers you to make full use of Node.js’s potential and create fast, scalable, and efficient applications. Whether you are a developer looking to upskill, or a business seeking to hire Node.js developers, a deeper grasp of these aspects will surely prove beneficial. In the world of server-side web development, Node.js has cemented its place as a robust and efficient choice. So delve in, explore its features, and happy coding!
Table of Contents
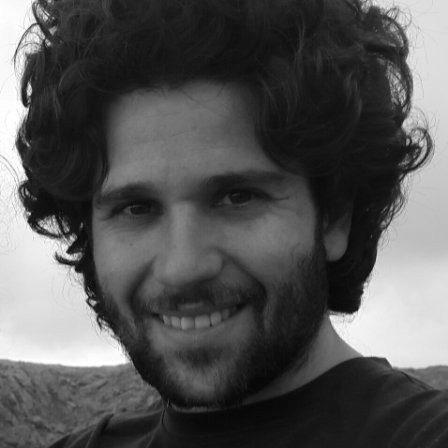
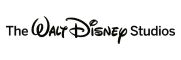