How do you create a server in Node.js?
Creating a server in Node.js is a fundamental task that involves using the built-in http or https modules to create an HTTP or HTTPS server respectively. Here’s a basic example of how to create a simple HTTP server in Node.js:
javascript Copy code // Import the http module const http = require('http'); // Define the hostname and port number const hostname = '127.0.0.1'; const port = 3000; // Create an HTTP server const server = http.createServer((req, res) => { // Set the HTTP status code and content type res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); // Send a response to the client res.end('Hello, World!\n'); }); // Start the server and listen on the specified port server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`); });
In this example, we import the http module, define the hostname and port number, create an HTTP server using the createServer() method, and specify a callback function to handle incoming HTTP requests. Inside the callback function, we set the HTTP status code, content type, and send a response to the client using the res.end() method.
To run this server, save the code in a file (e.g., server.js) and execute it using Node.js:
Copy code
node server.js
This will start the server, and you can access it by navigating to http://127.0.0.1:3000 in your web browser. You should see the message “Hello, World!” displayed in the browser, indicating that the server is running successfully.
Creating an HTTPS server follows a similar process but requires additional steps such as generating SSL certificates and configuring the server to use HTTPS encryption. However, the basic principles of creating and running a server remain the same.
Creating a server in Node.js is simple yet powerful, allowing developers to build scalable and high-performance web applications and APIs.
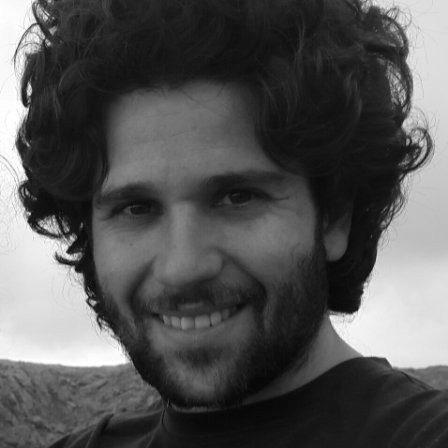
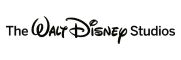