Node.js Q & A
How do you handle cross-origin requests in Node.js?
Cross-origin requests occur when a client-side JavaScript code running in a web browser makes a request to a server with a different origin (domain, protocol, or port) than the one from which the script originated. Browsers enforce the Same-Origin Policy by default to prevent such requests due to security concerns.
However, there are mechanisms to handle cross-origin requests in Node.js applications:
- CORS (Cross-Origin Resource Sharing): CORS is a mechanism that allows servers to specify which origins are permitted to access their resources. In a Node.js application, you can use the cors middleware to enable CORS handling.
javascript
Copy code
const express = require('express'); const cors = require('cors'); const app = express(); // Enable CORS for all origins app.use(cors()); // Define your routes // ... // Start the server app.listen(3000, () => { console.log('Server is running on port 3000'); });
You can also specify configuration options to allow only specific origins, methods, or headers.
- Proxying Requests: Another approach to handle cross-origin requests is to proxy requests through the server. In this scenario, the client sends the request to the same origin as the server, and the server forwards the request to the intended destination. Node.js libraries like http-proxy-middleware can be used to implement request proxying.
- JSONP (JSON with Padding): JSONP is a technique for making cross-origin requests by dynamically injecting <script> tags into the HTML document. However, JSONP has limitations and security risks, so it’s less commonly used today compared to CORS.
By implementing appropriate cross-origin request handling mechanisms in Node.js applications, developers can ensure secure communication between clients and servers while enabling cross-origin resource sharing as needed.
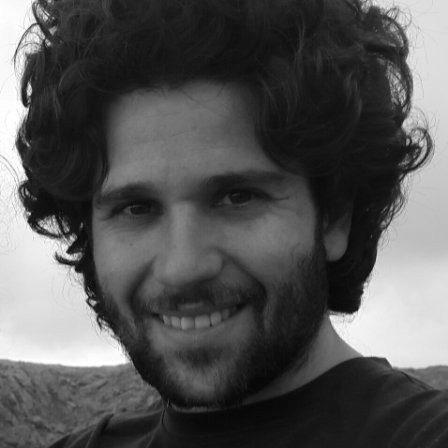
Previously at
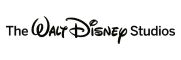
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.