Developing Custom CLI Tools with Commander.js and Node.js
Command-line interfaces (CLI) are essential for many development tasks, offering a powerful way to interact with software tools and automate processes. Node.js, combined with the Commander.js library, provides a robust environment for developing custom CLI tools. This blog delves into how Node.js and Commander.js can be used to create effective CLI applications and offers practical examples to help you get started.
Understanding Custom CLI Tools
Custom CLI tools are programs designed to be executed from the command line. They provide a flexible and efficient way to perform tasks, automate workflows, and manage systems. With custom CLI tools, developers can create tailored solutions to meet specific needs.
Using Node.js for CLI Development
Node.js is an excellent choice for developing CLI tools due to its non-blocking I/O, extensive ecosystem, and ability to handle various tasks asynchronously. Commander.js is a popular library for building command-line interfaces in Node.js, offering a simple and intuitive way to manage commands, options, and arguments.
Below are some key aspects and code examples demonstrating how to use Node.js and Commander.js for creating custom CLI tools.
1. Setting Up a Node.js CLI Project
To start, you need to set up a Node.js project and install Commander.js.
Example: Initializing a New Node.js Project
```bash mkdir my-cli-tool cd my-cli-tool npm init -y npm install commander ```
2. Defining Commands and Options
Commander.js makes it easy to define commands and options for your CLI tool. You can set up commands, specify arguments, and handle user input effectively.
Example: Creating a Basic CLI Tool with Commander.js
```javascript const { Command } = require('commander'); const program = new Command(); program .name('mycli') .description('A simple CLI tool') .version('1.0.0'); program .command('greet') .description('Greet someone') .argument('<name>', 'name to greet') .action((name) => { console.log(`Hello, ${name}!`); }); program.parse(); ```
3. Handling Command-Line Arguments
CLI tools often need to handle various command-line arguments. Commander.js allows you to define and process these arguments easily.
Example: Handling Optional and Required Arguments
```javascript const { Command } = require('commander'); const program = new Command(); program .command('sum') .description('Calculate the sum of two numbers') .argument('<num1>', 'first number') .argument('<num2>', 'second number') .action((num1, num2) => { const sum = parseFloat(num1) + parseFloat(num2); console.log(`The sum is: ${sum}`); }); program.parse(); ```
4. Adding Configuration Files
For more complex CLI tools, you might want to support configuration files. You can use libraries like `fs` to read and parse configuration files in JSON or YAML format.
Example: Reading Configuration from a JSON File
```javascript const { Command } = require('commander'); const fs = require('fs'); const program = new Command(); program .command('read-config') .description('Read configuration from a file') .argument('<path>', 'path to config file') .action((path) => { fs.readFile(path, 'utf8', (err, data) => { if (err) { console.error('Error reading file:', err); return; } const config = JSON.parse(data); console.log('Config:', config); }); }); program.parse(); ```
5. Creating Interactive CLI Tools
Sometimes, interactive prompts are useful for CLI tools. You can use libraries like `inquirer` to add interactive features to your tool.
Example: Adding Interactive Prompts with Inquirer
```javascript const { Command } = require('commander'); const inquirer = require('inquirer'); const program = new Command(); program .command('ask') .description('Ask user for their name') .action(async () => { const answers = await inquirer.prompt([ { type: 'input', name: 'name', message: 'What is your name?', }, ]); console.log(`Hello, ${answers.name}!`); }); program.parse(); ```
Conclusion
Node.js, combined with Commander.js, provides a powerful toolkit for developing custom CLI tools. From defining commands and handling arguments to integrating configuration files and interactive prompts, you can create robust and flexible CLI applications tailored to your needs. Leveraging these capabilities effectively will enhance your development workflow and automation processes.
Further Reading:
Table of Contents
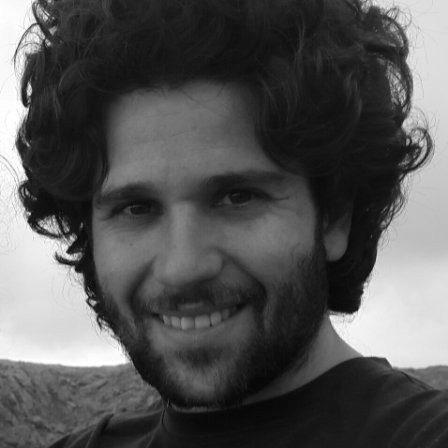
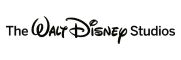