Building Data Pipelines with Node.js and Apache Kafka
In today’s data-driven world, building efficient and scalable data pipelines is crucial for processing and analyzing large volumes of data in real-time. Apache Kafka, with its high-throughput and low-latency capabilities, has become a go-to solution for streaming data pipelines. Combined with Node.js, a fast and asynchronous JavaScript runtime, you can create powerful data pipelines to handle various data processing tasks. This article explores how to build data pipelines using Node.js and Apache Kafka, with practical examples and best practices.
Understanding Data Pipelines
A data pipeline refers to a series of processes that move and transform data from one place to another. It typically involves data ingestion, processing, and storage. Real-time data pipelines are essential for applications that require immediate processing of data, such as monitoring systems, recommendation engines, and analytics platforms.
Why Use Node.js and Apache Kafka?
- Node.js: Its non-blocking, event-driven architecture makes it ideal for handling I/O-bound operations, making it a great fit for real-time data processing.
- Apache Kafka: Kafka’s distributed, fault-tolerant architecture allows it to handle large-scale data streams efficiently, making it suitable for building robust and scalable data pipelines.
1. Setting Up Kafka and Node.js
Before you start building your data pipeline, you need to set up Kafka and Node.js.
Installing Apache Kafka
Kafka can be installed locally or on a server. For a basic setup, you can follow Kafka’s official documentation to download and run Kafka on your machine.
Setting Up a Node.js Project
Create a new Node.js project and install the necessary dependencies.
```bash mkdir kafka-data-pipeline cd kafka-data-pipeline npm init -y npm install kafka-node --save ```
2. Producing Data to Kafka
The first step in building a data pipeline is to produce data to Kafka topics. Kafka topics are logical channels where data is sent and received.
Example: Producing Messages to a Kafka Topic
```javascript const kafka = require('kafka-node'); const { KafkaClient, Producer } = kafka; const client = new KafkaClient({ kafkaHost: 'localhost:9092' }); const producer = new Producer(client); const payloads = [ { topic: 'test-topic', messages: 'Hello Kafka', partition: 0 } ]; producer.on('ready', function () { producer.send(payloads, function (err, data) { console.log('Message sent:', data); }); }); producer.on('error', function (err) { console.error('Error in producer:', err); }); ```
3. Consuming Data from Kafka
Once data is produced to Kafka topics, the next step is to consume this data for further processing.
Example: Consuming Messages from a Kafka Topic
```javascript const kafka = require('kafka-node'); const { KafkaClient, Consumer } = kafka; const client = new KafkaClient({ kafkaHost: 'localhost:9092' }); const consumer = new Consumer( client, [{ topic: 'test-topic', partition: 0 }], { autoCommit: true } ); consumer.on('message', function (message) { console.log('Message consumed:', message.value); }); consumer.on('error', function (err) { console.error('Error in consumer:', err); }); ```
4. Processing Data in Real-Time
After consuming data from Kafka, you can process it in real-time. Node.js provides various libraries and techniques for data transformation, filtering, and enrichment.
Example: Real-Time Data Transformation
```javascript consumer.on('message', function (message) { const processedData = message.value.toUpperCase(); // Simple transformation console.log('Processed Data:', processedData); // Further processing can be done here }); ```
5. Storing Processed Data
Processed data can be stored in a database, data lake, or another Kafka topic for further use. Node.js supports various databases like MongoDB, PostgreSQL, and Redis.
Example: Storing Data in MongoDB
```javascript const MongoClient = require('mongodb').MongoClient; const url = 'mongodb://localhost:27017'; const dbName = 'kafkaData'; MongoClient.connect(url, function (err, client) { if (err) throw err; const db = client.db(dbName); consumer.on('message', function (message) { const processedData = { message: message.value, timestamp: new Date() }; db.collection('messages').insertOne(processedData, function (err, res) { if (err) throw err; console.log('Data inserted:', res.insertedId); }); }); }); ```
6. Monitoring and Scaling the Pipeline
Monitoring and scaling are critical aspects of maintaining a data pipeline. Kafka provides tools like Kafka Manager and JMX for monitoring, while Node.js applications can be scaled horizontally using tools like PM2 or Docker.
Example: Using PM2 for Scaling
```bash npm install pm2 -g pm2 start consumer.js -i max ```
Conclusion
Building data pipelines with Node.js and Apache Kafka offers a powerful solution for real-time data processing and streaming. By leveraging the strengths of both technologies, you can create scalable, efficient, and robust data pipelines tailored to your application’s needs. From producing and consuming data to processing and storing it, Node.js and Kafka provide the necessary tools to handle large-scale data flows with ease.
Further Reading:
Table of Contents
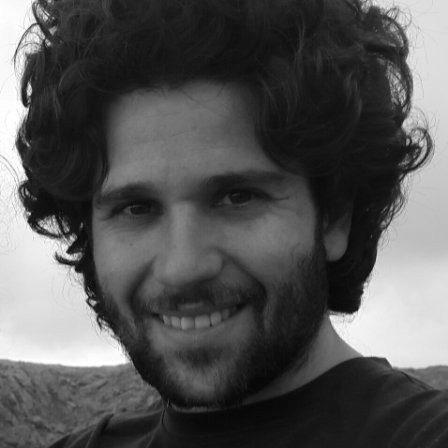
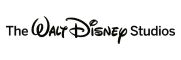